Code Your Own Video App Player in Android Studio!
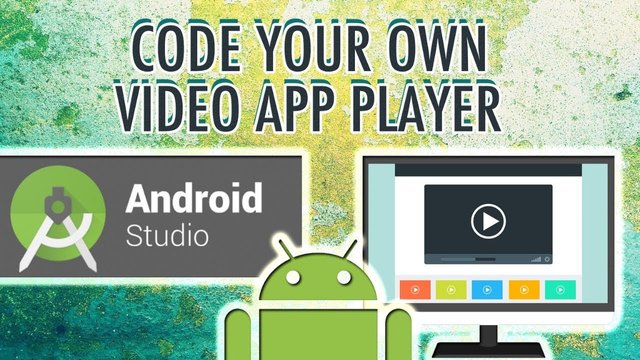
Hello and welcome to this new Android development course. This is part one of how to make a video player application for Android.
We’re going to start by opening our Android Studio environment and clicking on 'Start a new Android Studio project'. We will select an empty activity, and then we will click on next.
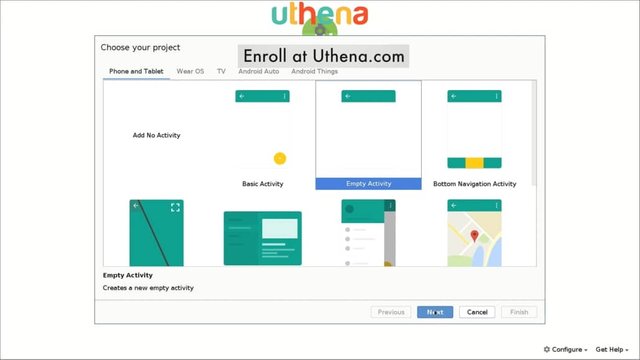
Here we change the name of the application to video player. We make sure the language that is selected is the Java programming language and the minimum API level is at least 16.
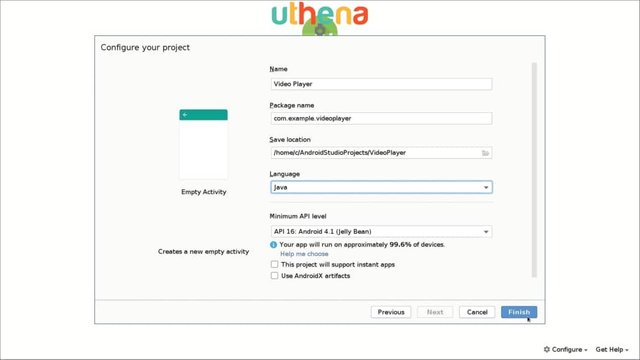
We will click on Finish and we will wait for the project to be loaded. This project, this application will basically do two simple things. The first one is getting all the mp4 files or other compatible video files, and then it will enable the user to play those files using the default video view widget which is the default way to play video in the Android operating system.
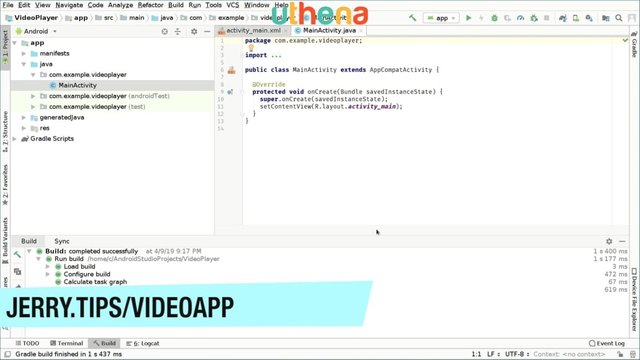
Right now, as you can see the project has finished loading and the first thing we’re going to do is to change the root element of our XML file.
If you will enjoy reading and contributing to the discussion for this post, will you please join us on the YouTube video above and leave a comment there because I read and respond to most comments on YouTube?
If you find anything helpful in this video or funny, will you please leave a like because you will feel great helping other people find it?
For that we will go to the left under the res folder and under the layout folder. We will find the activity_main.xml file. We’ll right click on it and then click on delete.
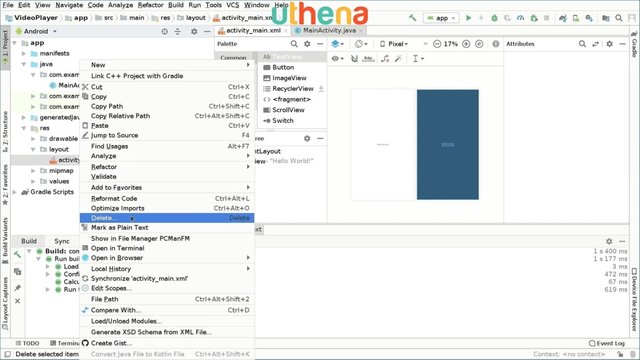
If you have these options checked please uncheck them and press in OK.
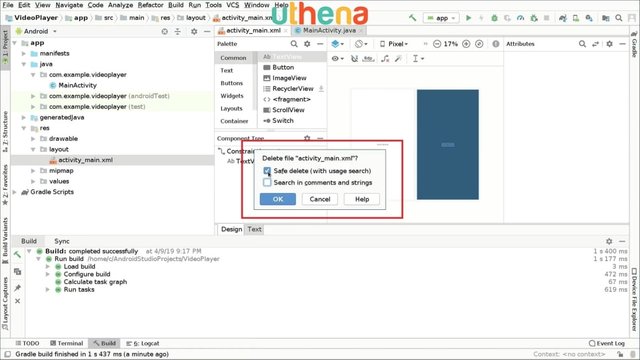
We have deleted that layout file as you can see we have an arrow right here which is normal, and now we will create it again but with a different root element.
For that, click right on the layout folder here on the left. Right click here, go to the new and layout resource file.
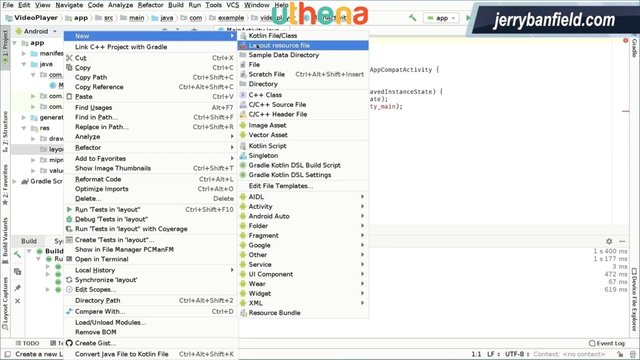
As the filename we will write the same it was previously which is activity_main just like this. We will change the root element to a linear layout as you can see right here.
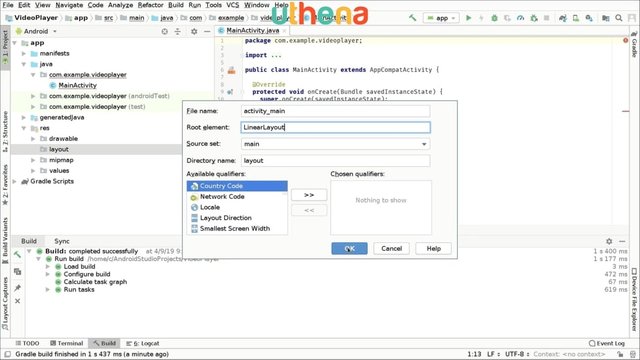
We will click on OK and as you can see here we have a new linear layout XML file. If we switch to text mode you can see that we have this linear layout as the root element of our layout.
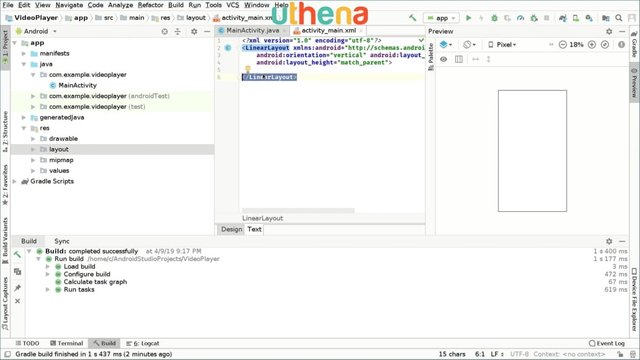
If we go back to our main_activity.java, we can see that these red warning disappears which is cool. For this tutorial, I will be running the application on an emulator. You can do it in an emulator too or you can do it on a real device.
Right now, I’m starting the emulator in order for us to make it faster in future app launches. The emulator is starting right now. I will leave it to load in the background.
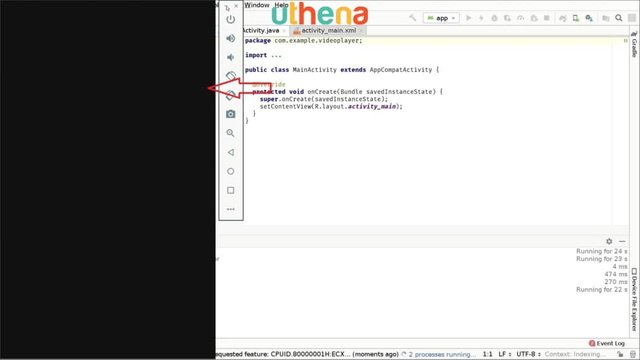
It’s loading right there and as you can see, I’ve sent the app to the device but now, we will leave the emulator aside for a moment, and we will focus on our application.
As I told you we have to first be able to get the files. For that, we will go into our manifest file right here and we will have the read and write files permission.
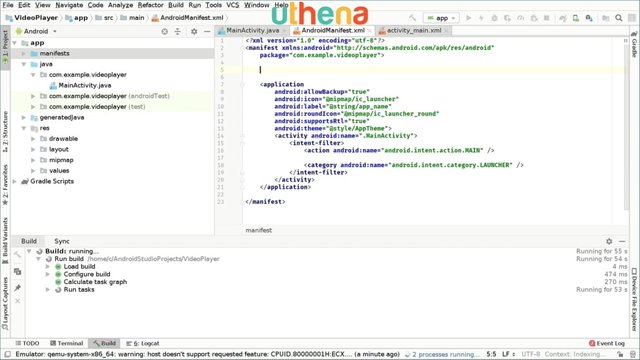
We will go ahead and start by saying user’s permission and we will specify the read and write permission for our storage. The IDE doesn’t auto-generated these for me because it’s loading something else. Right now, it’s installing the APK but now that it has finished installing it, I can start getting the help of the IDE right here. You go say users permission and we are going to type WRITE_EXTERNAL_STORAGE as you can see, and after that we are going to go and say user’s permission READ_EXTERNAL_STORAGE like that.
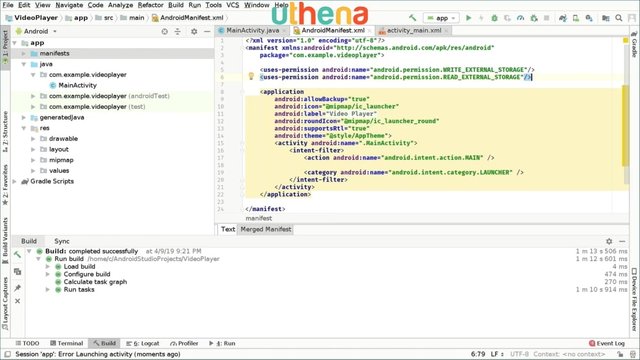
We have this right here and then the activity right here. Right here we will also set the screen orientation for the portrait because that’s the one we will be using.
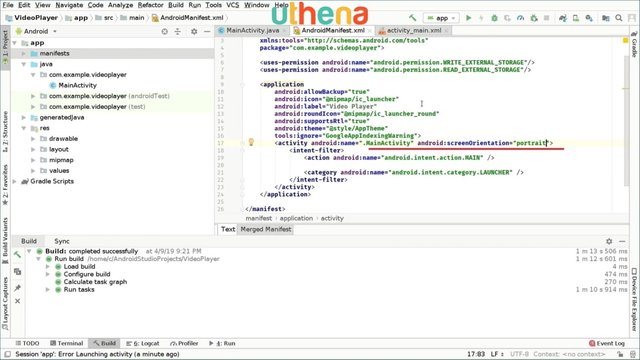
I will run the app on the emulator to see if it works or maybe it’s not working. I’m compiling it, I’m installing the APK as you can see and soon it will run here on the left.
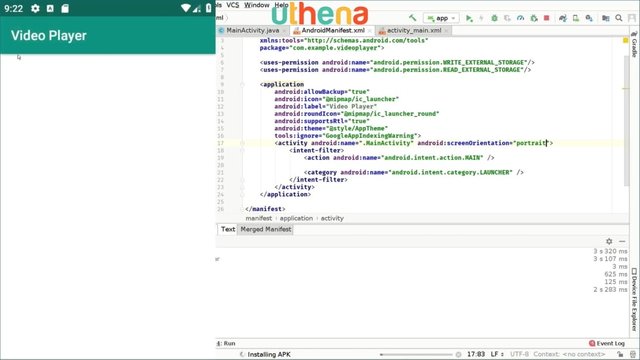
As you can see it is a simple thing that has nothing on it. It only has the video player title and also has the status bar title. I will remove both of those bars. To remove those bars, we go under the res folders and under the value’s folders, we open this style.xml file. Right here we can modify our style.
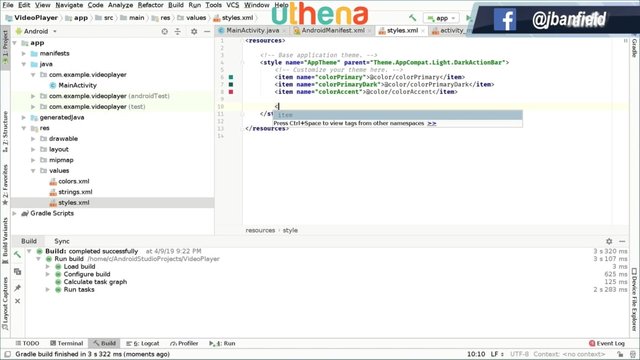
We will start by typing item windowNoTitle, and we will set these values to the truth as you can see, and we will open another item. Here we say windowFullscreen. We will also set these to true.
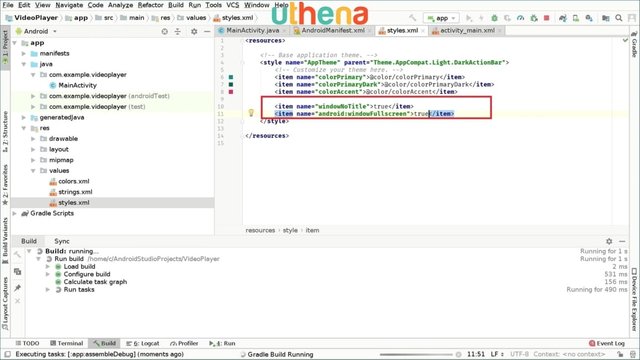
With only those two lines we have removed the bars so I will run it to test if that works well. I am compiling an app. It’s now sending it to the emulator as you can see. It’s empty. Everything is just a white rectangle.
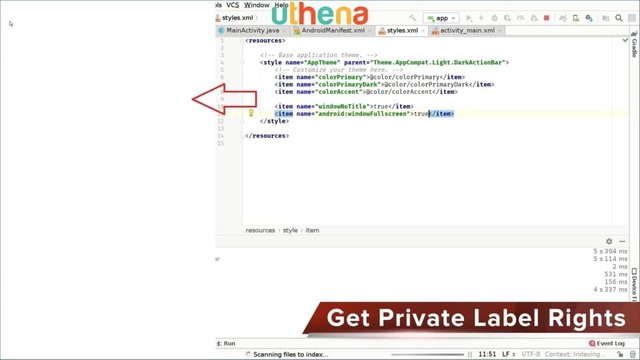
The title bars - the previous two bars were removed. We went back. We can now close the style file. We can now close this manifest file and we will have to create a way in which we can ask the user for permissions. If we are targeting Marshmallow or higher which is pretty common these days, we have to make sure that we ask for permissions, so that’s a very, very important thing to keep in mind.
We will be asking for permissions and creating a dialogue that will ask hey, we will use this permission and the user will click and enable or allow or not allow the permission. This is the onCreate method.
Right now we are in the main_activity. java file. After that we will start actually coding. The first thing is a code that will ask for the permission so the permission request code for that will define it as private static int. Actually, private static final because this will be fine value, so private static final int, and we will call it in uppercase REQUEST_ PERMISSIONS, and we will set this body to any identifier.
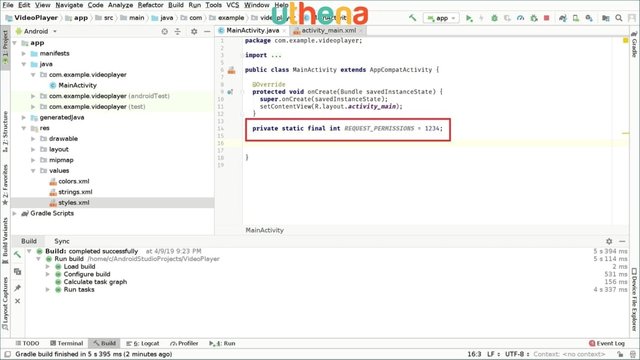
For example, I would set it to one, two, three, four, okay?
Then we will create an array of strings that will specify the actual permissions that we will ask the user to grant. We’ll go and say private static final but now string - this is string array - so we specify an array right here and we will call this PERMISSIONS as you can see. We open brackets and we end the line with a semicolon. Inside here we will go and type Manifest.permission.READ_EXTERNAL_STORAGE. As you can see will type a comma and the next line will type Manifest.permission.WRITE_EXTERNAL_STORAGE.
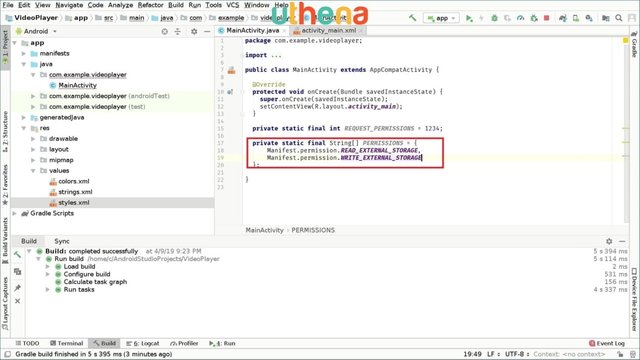
Now, we have specified the request code and the permissions we want to request. We also have to specify the number of permissions, in this case, we’re asking for two, so we’re going to go ahead and type private static final int PERMISSIONS_COUNT. We will set these to two.
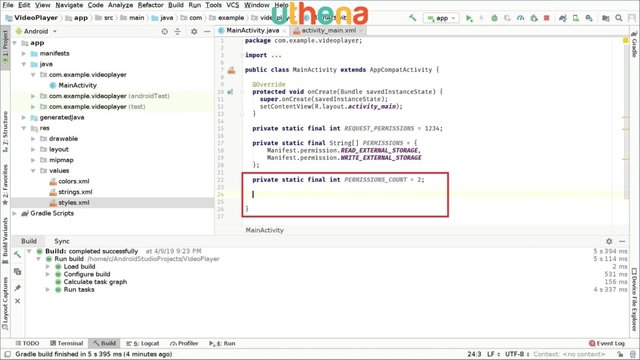
Now, we will ask for permissions and we will preparing the method. The first method that we will use is to check if the user denied any permission. For that we will create the following method, private boolean which we’ll return true or false. Whether the permissions are denied or not. So we will call this arePermissionsDenied as you can see right here. Inside this, we will write a for loop so we go ahead and type for, we open parenthesis and type int i=0; and then we go and say i < PERMISSIONS_COUNT. So we will check this for every permission. Then i++ as you can see right here.
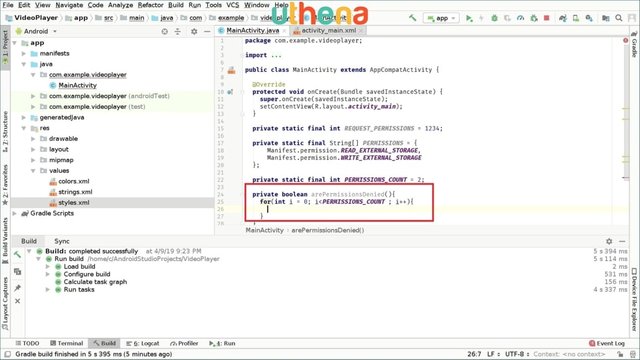
Inside this for loop, we will check if the user has granted permission or not, so for that we go ahead and say if checkSelfPermission. We will pass the current permissions which is permission at index i. If checkSelfPermission is different than PackageManager.PERMISSIONS_GRANTED. So basically here, what we are saying is if the permission is not granted then do something, okay? Pretty easy, right?
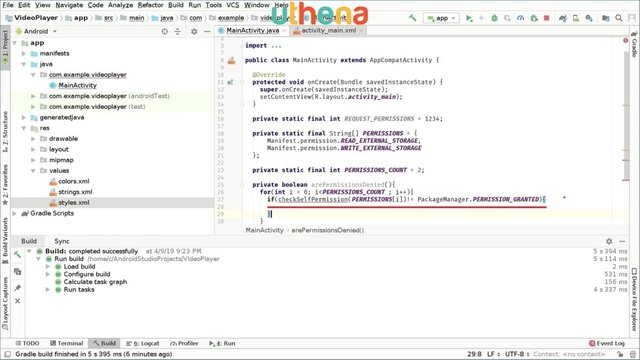
If the permission is not granted, we will return it true because we are returning the permissions are not granted. That’s the name of the method. arePermissionsDenied? So if they are not granted they are denied.
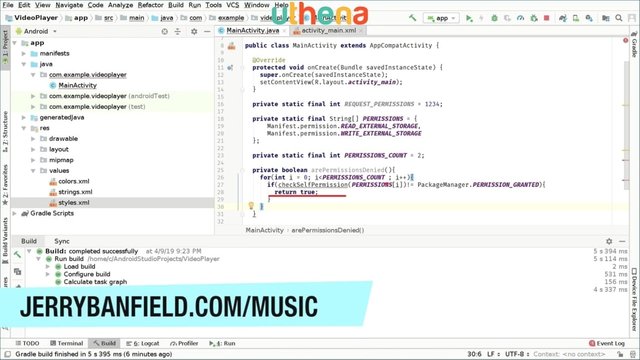
After the for loop we, of course, have to return false. This means there was no permission denied. Every permission was allowed.
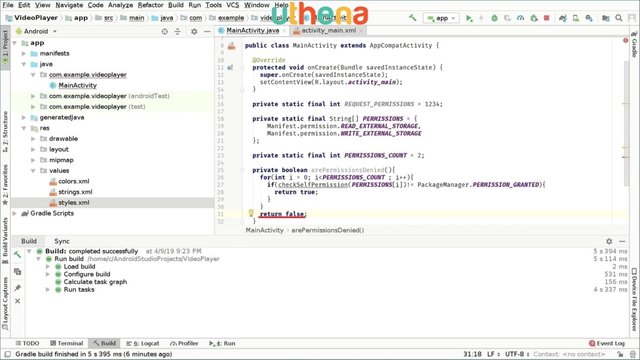
As you can see here we have a warning that says call requires API level 23, but we don’t really care about this warning because we will call it only when API level is 23 or later, so we can go ahead and say suppressLint new API annotation. Now, the warning is gone.
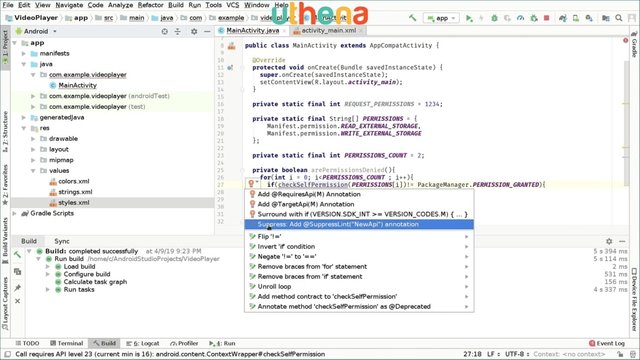
This is the first method to check the permissions. We have to also check for another method which will be called when the user clicks denied or allow permission. We’re going to type override public void onRequestPermissionsResult. As parameters, we’ll go with say final int requestCode. So this is the code that we passed.
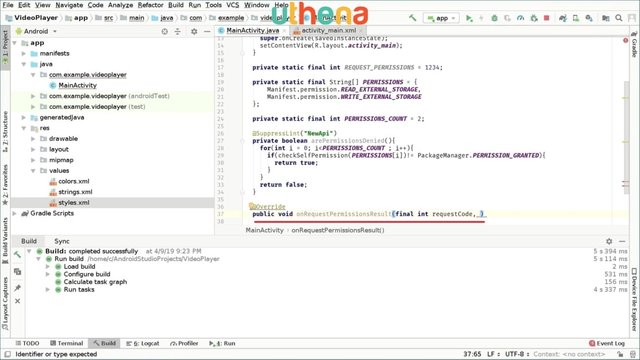
We will check if this gets passed the code that we passed previously. That’s the request code. Final int requestCode. Final string[] permissions and I’ll go to the next line and say also final int[] grantResult. These are kind of the parameters that will be passed to this method.
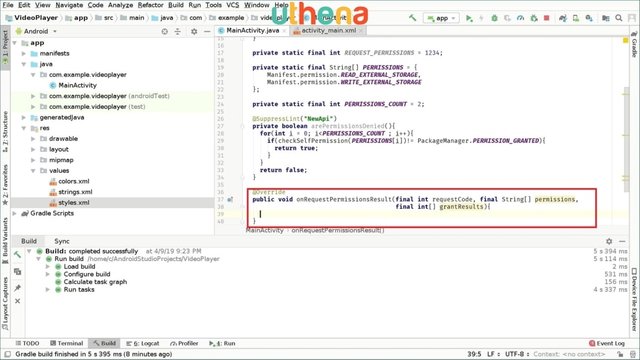
We are overriding it so we will have to call the super method. For that we’re going to say super.onRequestPermissionResult, and we’ll pass the same parameters. So the first one will be requestCode. The second parameter we will pass will be permissions, and the third will be grantResult as you can see.
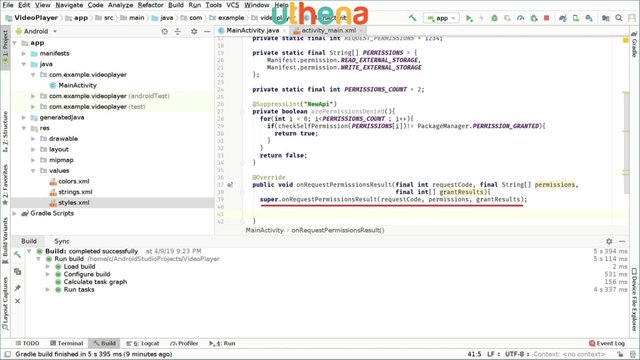
Now, we want to go and check if the request code is actually the same that we sent and we will send these code right here which is one, two, three, four, which is called REQUEST_PERMISSIONS.
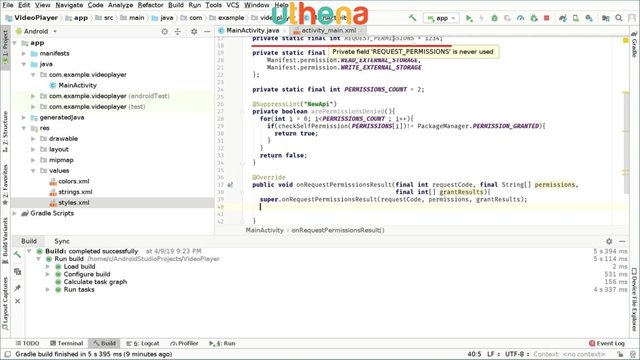
We’re going to go and say if requestCode == REQUEST_PERMISSIONS. We also check if and grantResults.length is greater than zero which checks if the results are not empty. Inside here we will have to check if the permissions are denied or not, so we’re going to say if arePermissionsDenied, so we call the method that we created previously. Here what we will do if the permissions are denied, well, the app cannot work without these permissions, and if the user denies the permissions then the app is not useful. So the user should allow the permissions in order to use the app. Here as a quick fix we will open two parentheses. Inside the inner one we’re going to type ActivityManager. We go outside this and type objects.requireNonNulll and we’ll type these.getSystemService as you can see.
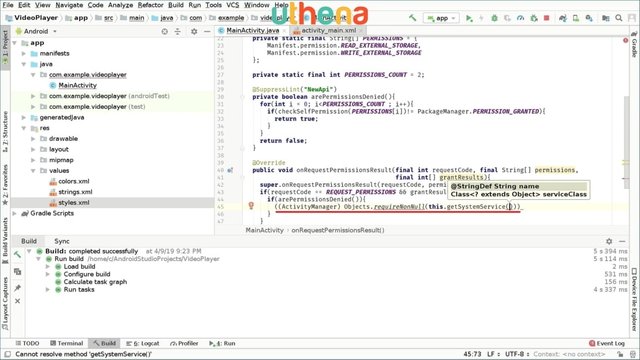
What this is doing is basically telling the system to delete the application user data in order to enable us to keep asking for the permission every time the user launches the app. It’s just a quick fix. So we’re going to say ACTIVITY_SERVICE. We’ll pass the parameter right here and after these three parentheses we’re going to type .clearApplicationUserData. As you can see here it has a kind of warning again that requires API 19 but we will only call this if API is greater than 23 actually.
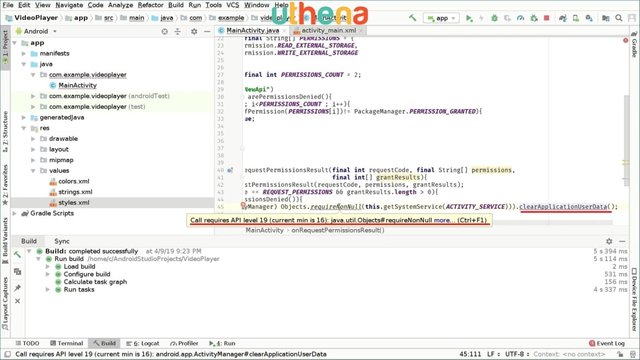
We go and click suppressLint warning.
That happens if the user denies this and to close the app we will just type recreate. Right here what will happen if the user actually enables or allows access for those permissions, we will call the onResume method as you can see right here.
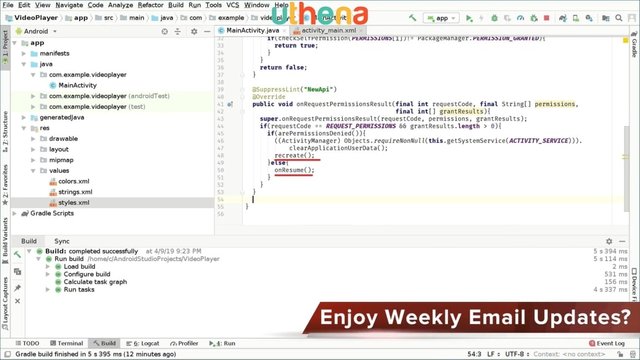
Since we called the onResume method we have to override that and that method will actually be the main method of our activity. For that, we’re going to go after these and type @override then protected void onResume. We will open this and type super.onResume.
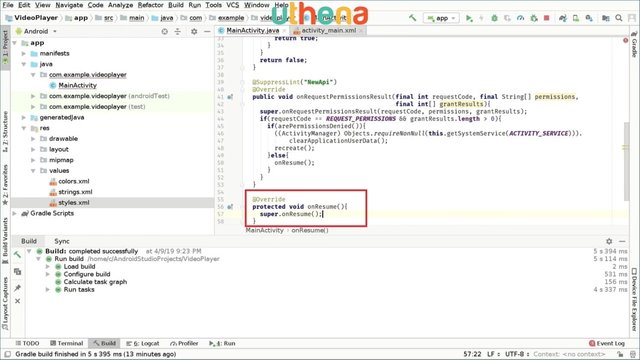
Here is when we do the check of the Android version. To check the current version the Android we will type the following. Build.VERSION.SDK_INT. So if Build.VERSION.SDK_INT is greater or equal than Build.VERSION_CODE.M which means Marshmallow. We check first if it’s Marshmallow or above and we also check something else, and arePermissionsDenied right here. We will request permissions. So we’ll type requestPermissions and we will pass permissions and the request permission code which is REQUEST_PERMISSIONS as you can see. And after this, we will return. As simple as that.
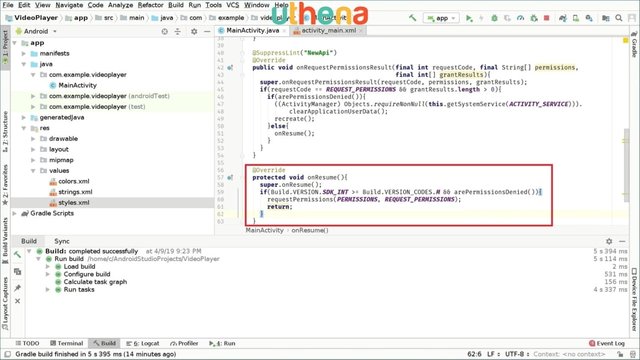
We also have to check this every time the onResume is called because the user may disable the permissions for our application. This is basically how we do it. Now, we’re going to check if the app was initialized because the onResume method will be called many times and we just have to initialize the app ones. For that, we will create a flag which will be called private boolean isVideoPlayerInitialized and this defaults to false, of course, in Java.
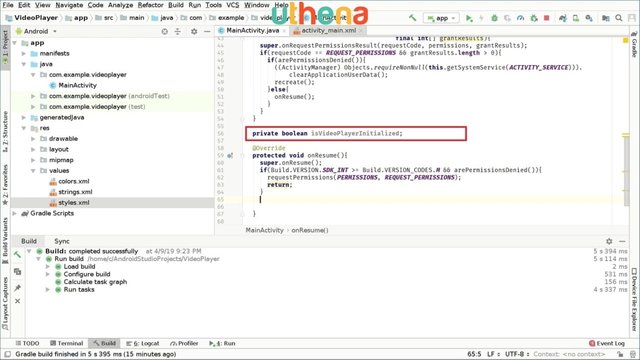
Inside the onResume method, we will check if !isVideoPlayerInitialize which means if it’s not initialized, we will initialize it. The last line of this if statement, we will say that the video player is actually initialized, so we’ll set these to true.
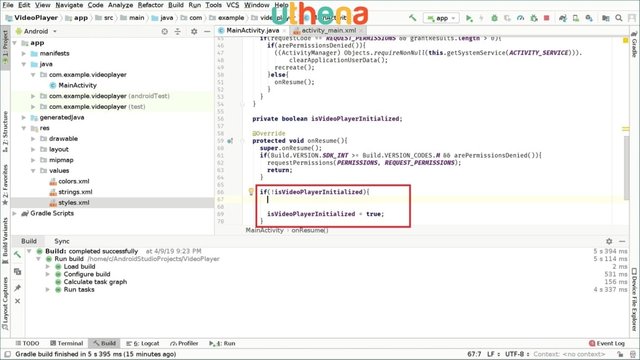
Right now, we have these right here and to initialize this, we’ll have to actually have some UI elements. The first thing we will do is to list the videos. To list the videos, we will go and create a ListView, so we go inside our linear layout and type ListView with match_parent, height 0dp, weight 1. We will add an ID to this ListView which will be listView as you can see.
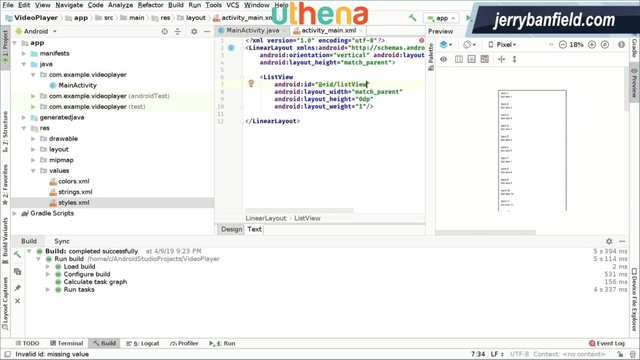
We go back to our main_activity.java file and right here we’re going to say final ListView listView, and we will assign this to findViewById R.id.listView as you can see.
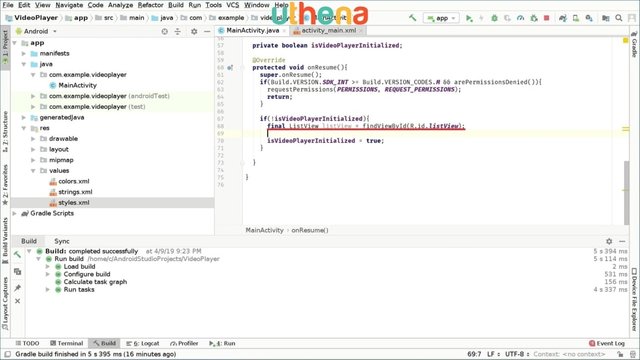
We have our ListView right here but we also have to create the items that will be displayed on the ListView. In this case, the video name and the extension of the video, for example. For that, we open the layout folder, we right-click the layout folder and click on new layout resource file.
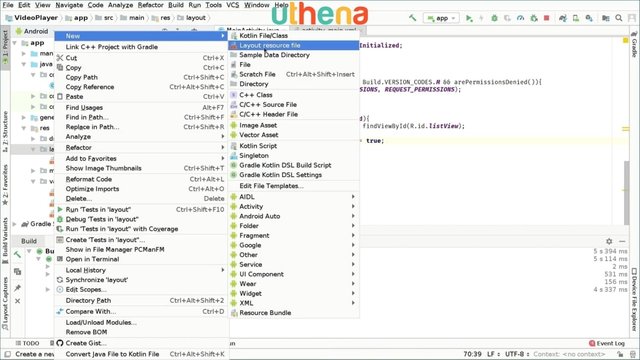
We will create a file name. We will type item and the root element of this will be textview because it will show text.
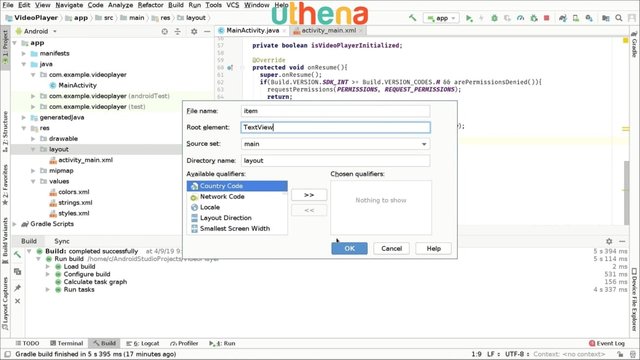
As you can see the item is created right here. I can close the preview to see better the code right here. Inside this text view element right here I will add an ID. This ID will be my item, for example, or something like that. Now, we have created this item which should be accessed from our main_activity.java file.
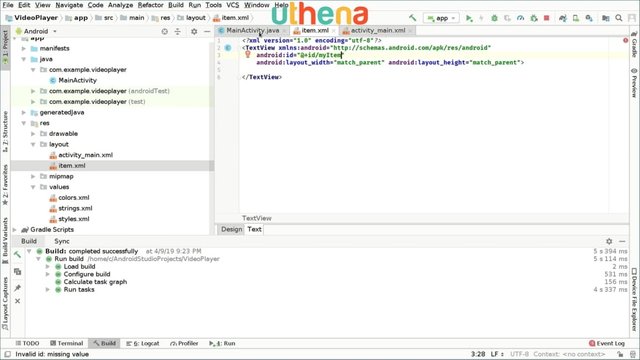
The height of this could be different because we don’t want it to be match_parent. Maybe we can change the height to 50DP. As you can see I can do this in a new line in order to make it easier to see. We have basically with the match_parent, height 50DP and we also want to center it vertically, so we’re going to say gravity center_vertical as you can see right here. Maybe the default font size is too small. We will increase it by saying text size, and we’ll go and say 25 SP. Maybe not. Maybe 22 SP will be great. I will leave it there. Maybe we can change the color too. So we’re going to go and say textColor, and we will go and define a color.
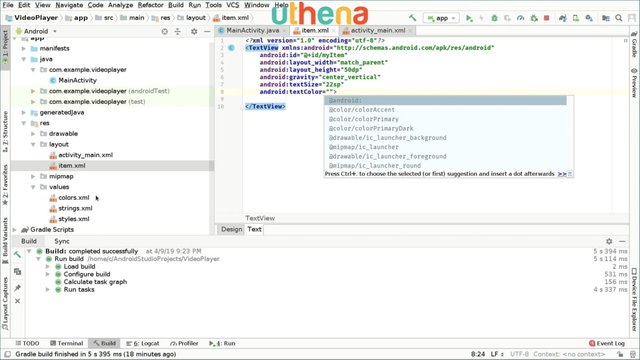
For that we will go under the Values folder, colors.xml file. We will open an element named color, and the name of the element will be black. To define the black color in hexadecimal RGB values we go and type the number sign or hash tag and then we’re going to type six zeros. As you can see we defined the black color.
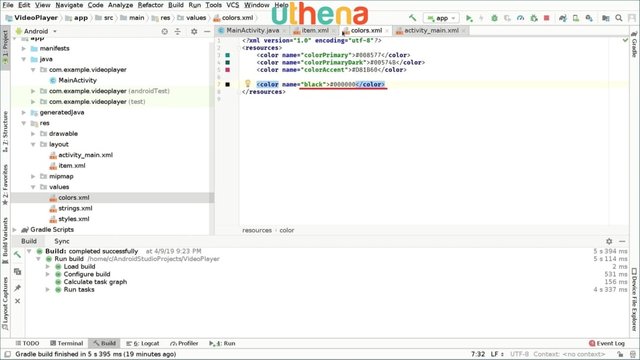
We’ve got an item and in text color we’ll type black, so that’s the color we defined as black. As in the preview here you can see the size of the textview, and If I add a text field right here like hello we have this.
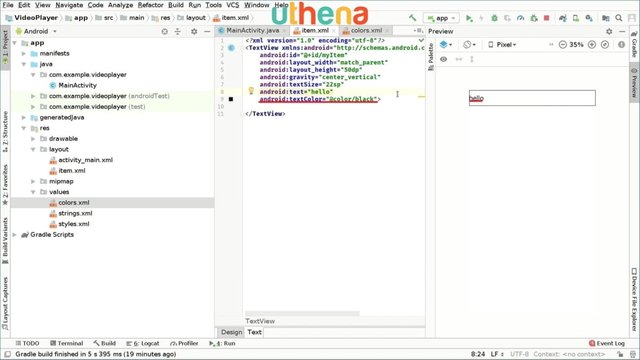
but this is not nice because it doesn’t have padding. We will add paddingStart. We will add 10 DP maybe 16 DP. We will also add paddingLeft 16 DP which in some regions of the world this is used in some Android versions. We will also add padding at the end so we’re going to say paddingEnd 16 DP and paddingRight 16 DP.
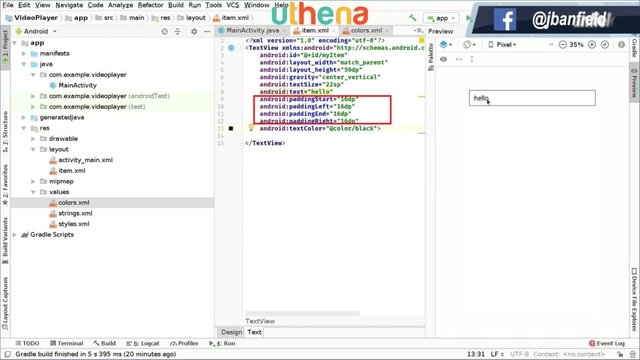
Right now, we have our hello thing right here. We can now just delete this hello text because we don’t need it for our application. In the next part we will initialize it, the video files and start doing more features.
This Is Part Two Of How To Make A Video Player Application For Android.
In the previous tutorial, we initialized the UI and some elements as well as asking permissions, and that kind of stuff.
Right now, we will continue working on our app, and for this we will go to our main_activity.java file.
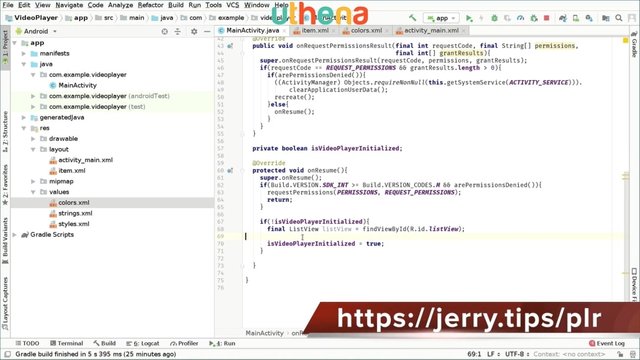
As you can see we previously initialized ListView right here. We will create the adapters.
What’s an adapter?
Well, an adapter is a class that sets the elements or the information in every cell of the ListView as it is required. It depends on which part of the ListView the user is navigating at any given moment in time, so the text adapter will figure that out and actually set the needed and required information. For that, we will create an inner class in order to maintain everything on a single source file for simplicity.
We go and say class and we’ll call this TextAdapter because this is an adapter that will show text.
What text will we be showing?
Well, the file name of our video files. So, TextAdapter and this will be set to extend BaseAdapter which is the base class of an adapter. Inside this BaseAdapter as you can see we have a warning right here that tells us to implement the method.
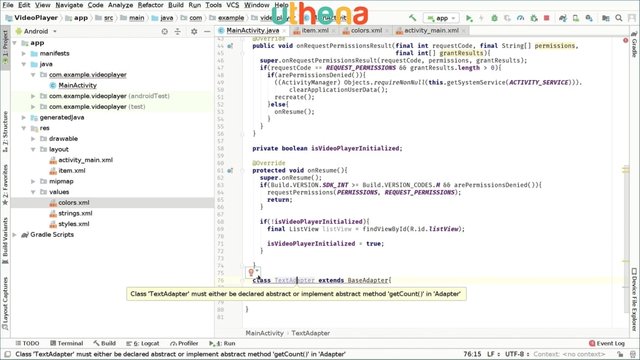
We will click on that and we’ll click on OK and the method will be automatically implemented for us. Before this getCount method, we will start creating our method which will be the setData method. For now, we have to create a global variable that will define the data we’ll be using. For that we will say private List <String>. We call these data. So it’s an array list of strings which is great. Make sure you import the list thing and type string. It’s called data and we’ll set this to new ArrayList as you can see.
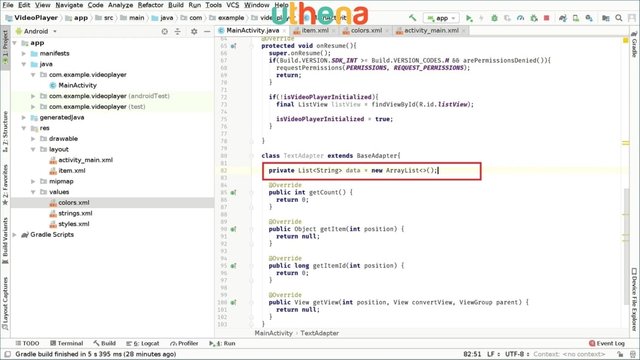
Right now, we’re inside here and we will create our setData method. For that we will type public void setData, and inside this, we have to pass some data which will be passed from the outside world. That data is an array list, of course, of strings. I have to use the same type and this will be called mData for us to be clear with what we mean. Now, we will set and check if everything is okay, so we will go and clear the data. We are going to say data.clear(). It clears the previous data as you can see, and we’ll add all the data that we have right here. We’re going to say data.addAll and it’s here we will say mData because that’s the parameter that it’s passed. After we do this, we will tell the adapter that we have new data. For that, there is a default method, we have to call which is notifyDataSetChanged() and that will be it for our set data method.
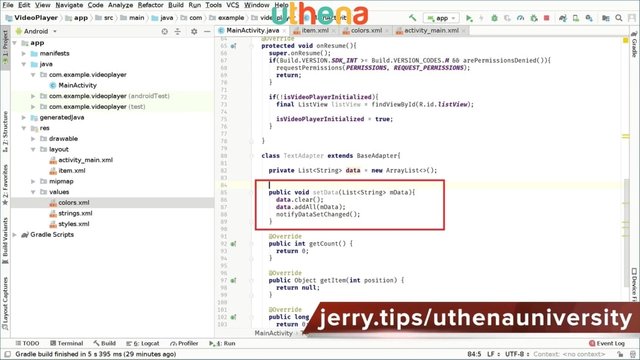
On the getCount method, this method will be called by the ListView to know how many items do we have? That will return data.size. Pretty simple. The getItem method won’t be used for us for now and the getItemId won’t be used either. Now, we have those methods. The getView method is actually a pretty important one. It’s the most important method actually which defines how the adapter works. First we will check the convertView which is to be passed if it’s null. So we will say if convertView equals null then we will assign it. So we’re going to say convertView, and we’ll set it to layoutInflater.from we open parentheses and type parent which is the parent, the element passed as a view group type. So parent.getContext and outside this we’re going to type .inflate.Here we will type the name of our item which is this item XML right here, so we’re going r.layout.item because that is the item we will inflate which is the text view on every cell of this view.
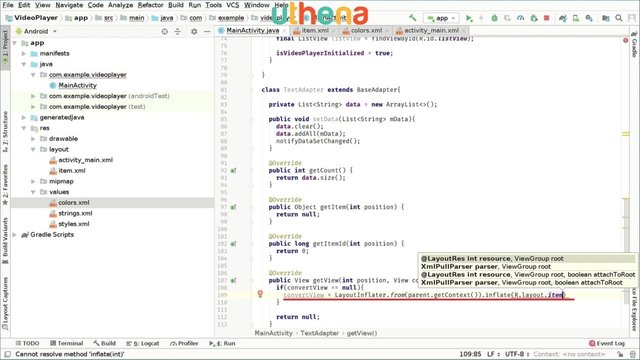
We’re going to go ahead and type comma, parent and we’ll attach to root false. Don’t forget to end it with a semicolon. I can put this in the new line in order for you to see what I’m doing right here. If convert view is null which, for example, we will know the first time, and when a new cell comes into focus, into scope, then this will be called again. We will set the tag which the identifier that we will retrieve. So for that we go and say convert view.setTag and we will pass a classed loop just create right now.
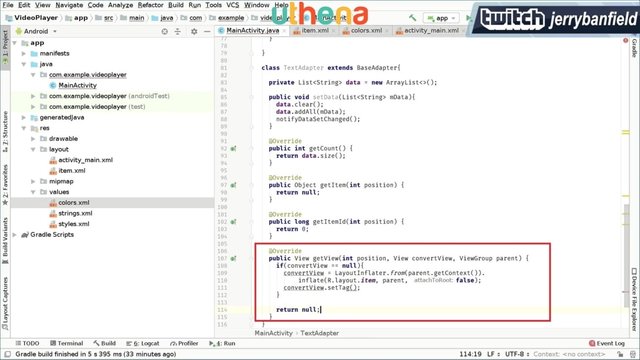
Outside the get view method we will create a view holder class which is just a simple class that enables us to set the text view stuff. Here we’re going to say class ViewHolder. This is outside the getView method but still inside the adapter class. Class ViewHolder and we will open brackets right now, and we’ll type TextView info.
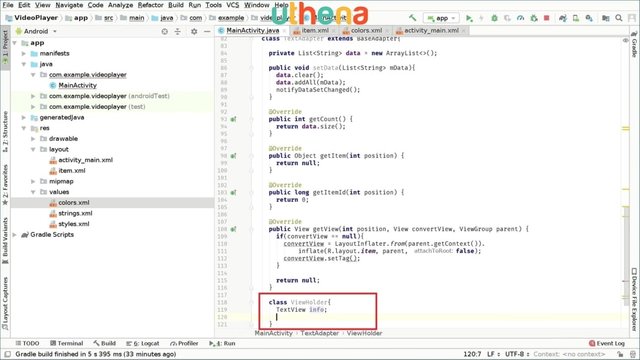
As a constructor method we go head and say ViewHolder. We’ll pass, of course, TextView, type will be called mInfo for us to clarify more. When the ViewHolder method is called this will set info which is the outside element to mInfo and that’s pretty much it.
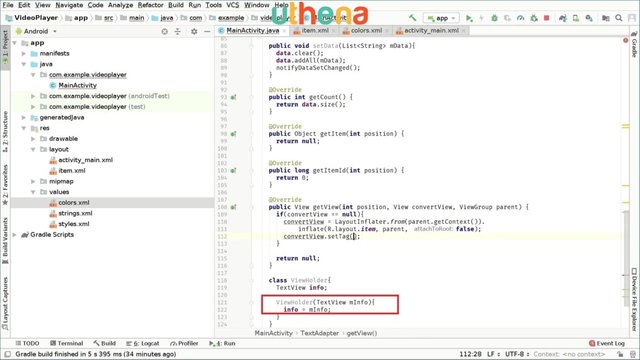
Now, we can go back to our setTag we were calling previously, so right here. We can say new ViewHolder which is the class that we just created and we will type another parenthesis. Inside this parenthesis will be TextView outside that will be findViewById. You will find the actual ID that was inside our item. So we go ahead and say item, and that was called myItem. We go and findViewById. We’ll type R.id.myItem as you can see. We have to find our item right there and we can continue to work in our getView method.
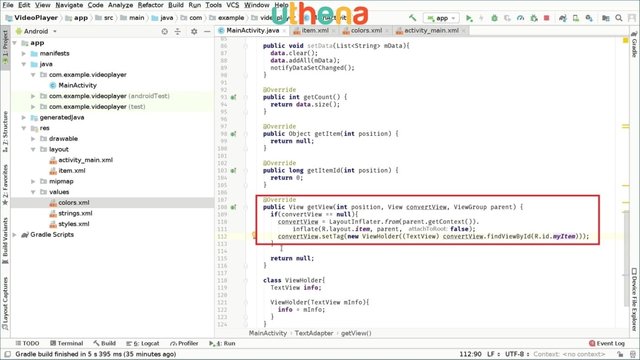
After the if statement we will create a ViewHolder item which will be ViewHolder holder. We will set this and we will cast this to ViewHolder and this will be equal to convertView.getTag. So we are getting the tag we previously created. Now, we will get the item of this in order for us to know what we’re doing right here and we will set the text to the item that we want, but the items will contain the path of the file, so we don’t want to display all that thing. We only want to display the name of the video file.
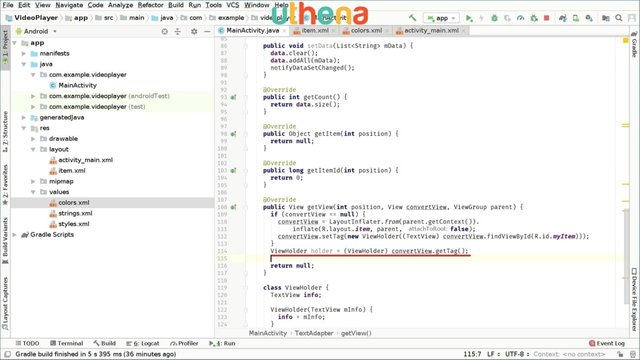
For that, we will create a string, so final String Item, and we will set these to data.get(position). We’re getting the current element of the current position of the data array list. So that’s item. Then we will go and set the text . For that we are going to say holder.info.setText, and we will set this to Item.substring. We’re defining the part of the whole string we want to print. So substring will be item.lastIndexOf and will be single quote, forward slash and outside this we’ll type +1.
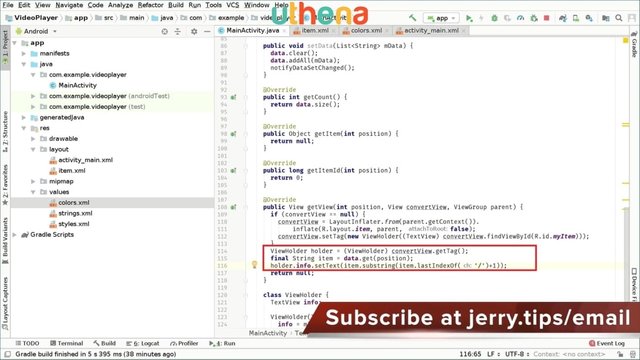
What this is saying is basically just display the name. So you’re getting the last forward slash so just before the name starts. So as simple as that. Right now, we’re getting this and instead of returning null, we have to return convertView as you can see right here.
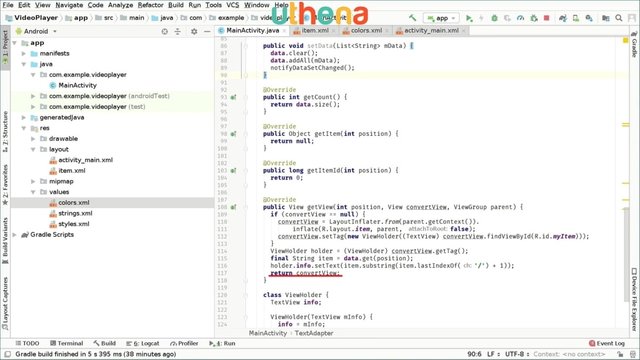
Now, our adapter is ready to be used. So we have to use our adapter right now because it’s just ready for us to use it. To use the adapter we have to just set it up once. We will set it up in the onResume method. We will kind of link that adapter to our ListView. So we go to the onResume method inside this if bracket, and we will say final TextAdapter. I’ll call it textAdapter and we’ll say new TextAdapter.
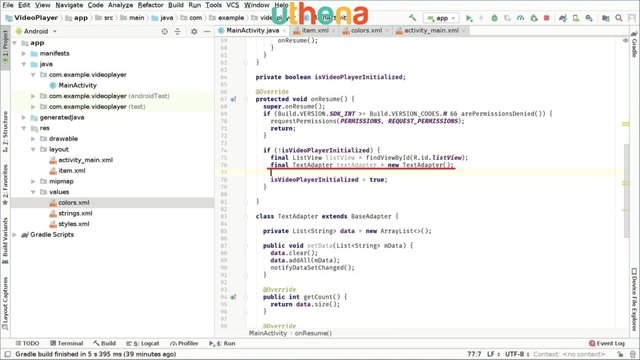
After this we’ll say TextAdapter.setData, because we have to set data to something. We don’t have data yet so we’ll create some right here. Final List<String> because remember that was the data type we used. We will call this videosList, for example, and this will be set to new ArrayList<>.
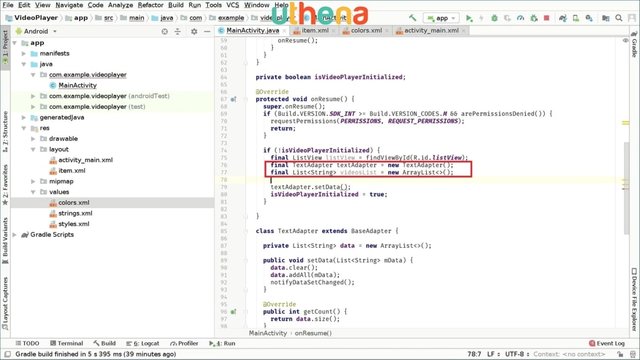
Here we will create a for loop that will populate the data so you can say for(int i=0; i< 100; i++. So this is just for testing. Inside this we’re going to say videosList.add and here we will add String.valueof(i) just for us to test, and use the index as the number of our cells.
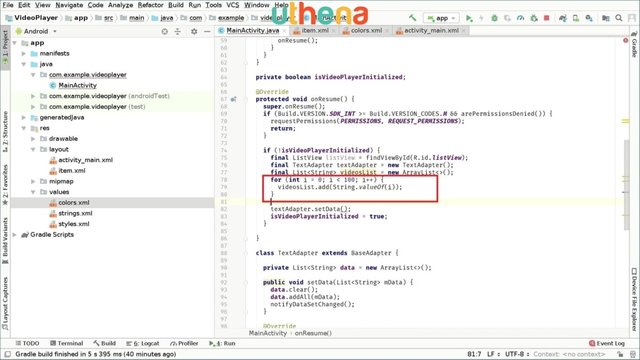
We have populated this and now we have setData and the data we will pass will be videosList.
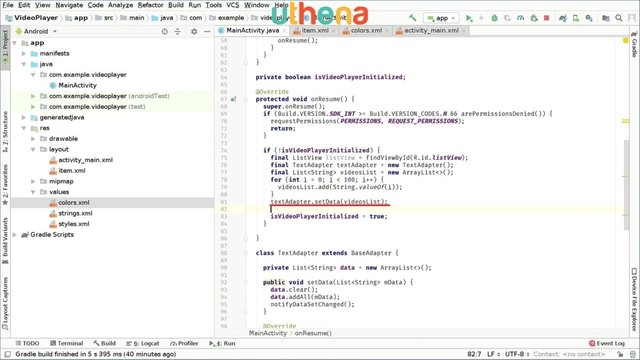
After this, we’re going to go and say listView.setAdapter and these will be textAdapter. Now, we will run the app and wait for it. Hopefully it works.
Now it’s been compiling and running and as you can see it is asking for permissions.
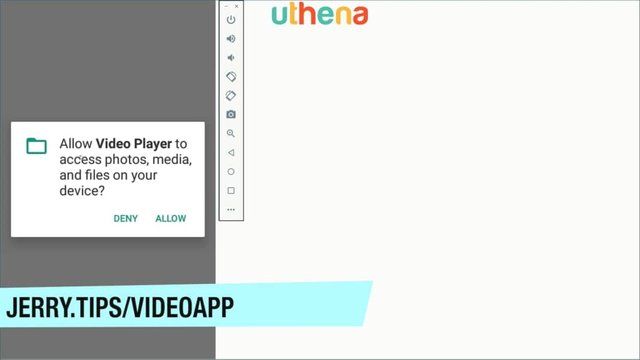
It says 'Allow Video Player to access photos media and files in your device' and you will click on allow. Actually, just to test these I will click on deny. So if you say deny then the app is closed. If I go and search for our application which is the video player it’s asking again for permissions and this time I will allow permissions.
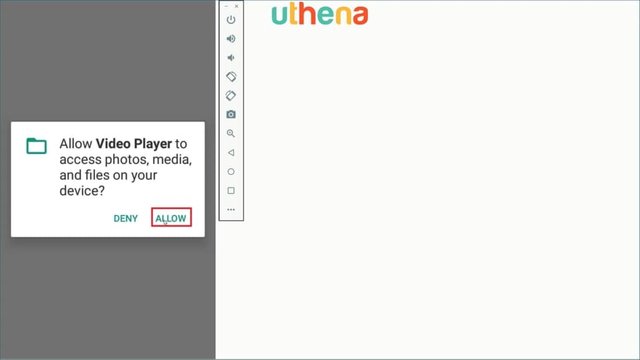
I click on allow as you can see we have our list view working great.
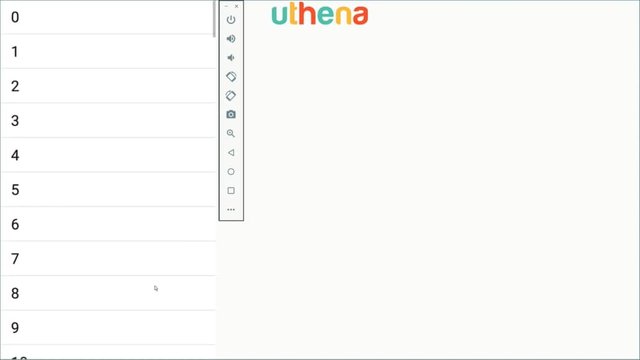
So we have numbered items. Every cell of these is set to the index of that. So it’s from 0 to 99 because it is a hundred elements as you can see. This is great. This is cool.
When we have the videos files here will appear for example myvideo.mp4, and when we click on that video then the video player will open, and will start playing the video. Then will maybe have a button that will close the video player and enable us to play another video, so we can maybe scroll down here and click on another video, and that video will be played again and so on and so forth.
GET MORE OF THIS COURSE
Would you like to continue learning about the Code Your Own Video Player App in Android Studio Course? If you are interested will you please buy the complete course, Code Your Own Video Player App in Android Studio, on the Uthena Education Platform..
You can also get the first hour and fifty-two minutes of the course completely for free on YouTube.
Thank you for reading the blog post or watching the course on YouTube.
You may like to read this post: Build an Android Studio Gallery App Like QuickPic Google Photos FOTO Piktures A+ and Camera Roll
I love you.
You’re awesome.
Thank you very much for checking out the Code Your Own Video Player App in Android Studio Course and I hope to see you again in the next blog post or video.
Love,
Jerry Banfield.
Posted from my blog with SteemPress : https://jerrybanfield.com/codeown-video-app-player-android-studio/
Hi @jerrybanfield i give you votes nice to post thanks for the support I am new user thanks thanks thanks very very thanks for support I'm Vetoing your post then please vote me! @timesoffitness