[DIY Game Series] Create a simple game with Javascript
[DIY Game Series with @zulman]
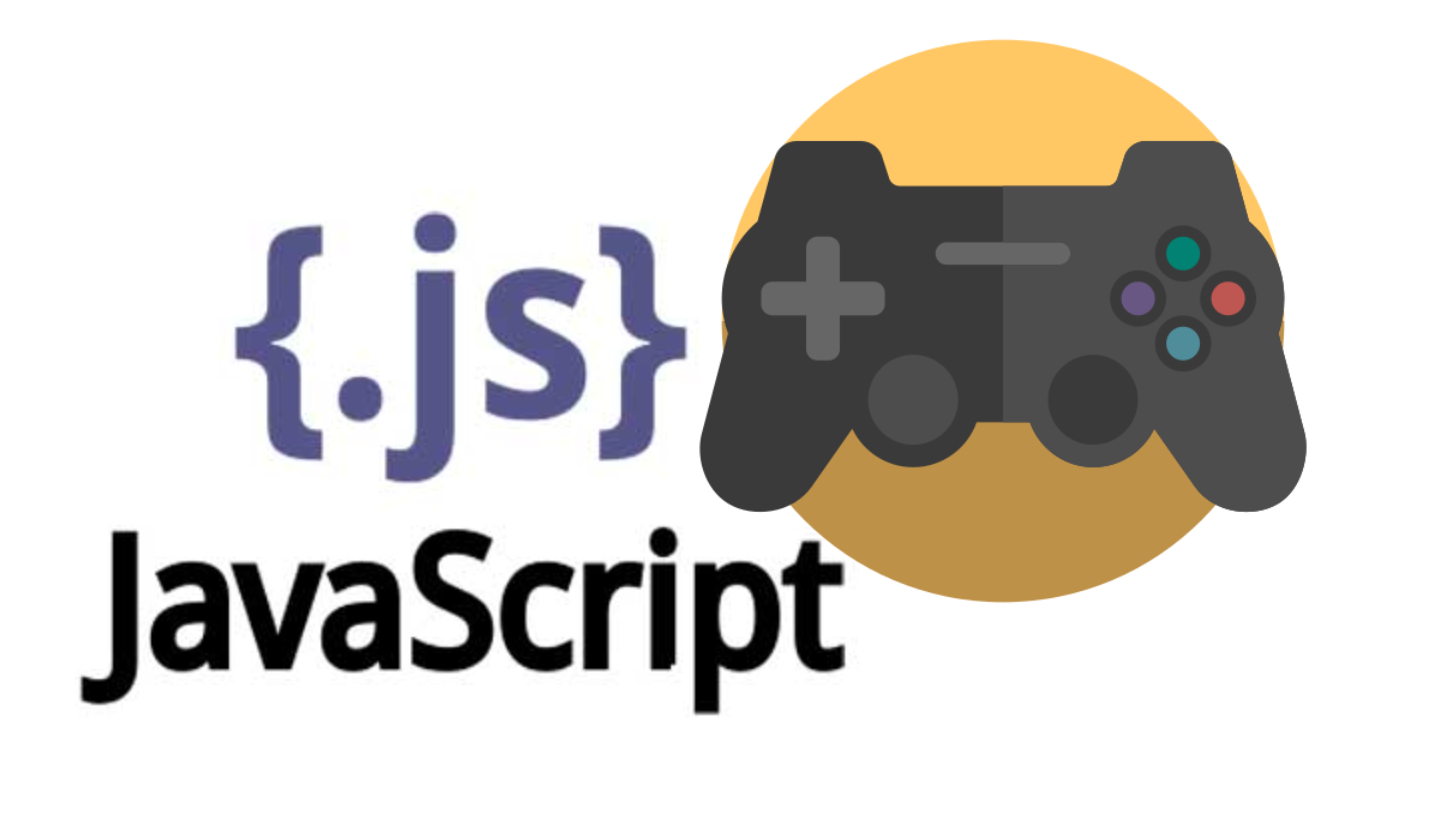
Hi guys. How are you all today? Welcome back again to my blog. It has been a week since I plan to write this post in this blog. However, I have been busy with my work lately. So, I focused to finish my work and now I have time and decide to update my post here on Steemit. If you have been following my article, you already know that in my blog so far, I only review some old or current games that available. But todays I am going to post something a little bit different. In today's post, I am gonna share my learning experience on how to build a simple game with only Javascript, CSS, and HTML.
So, here we are going to create a very simple game using html, css, and javascript. In this article you are not going to find the explanation about what is that html, css, and javascript. Here i only explain how you can create this simple game and share the code as well. And then, honestly I myself still learning about javascript, so the code might be not the best, but at least the game is working.
The game that we try to create is as follow
The game that we are trying to build is a very simple game, wit the following information:
- The game will have a rectangle as our controlled character.
- The rectangle going to be move to the right side endlessly.
- The rectangle need to avoid some obstacle, once it hit the obstacle, the game is over.
In order to follow the article. This is what you need.
- a computer off course.
- a browser. I suggest using google chrome.
- a text editor. You can use what do you like. My personal favorite is "Atom"
- a little bit knowledge about html, css, and javascript.
Notice:
This is could be not the best article about creating game with Javascript. I am currently starting to learn javascript and this article is something that I have learnt. Perhaps, you will find some similarities with the source in term of the code.
Alright, without further ado, let's start.
Step 1 : Create HTML page
To start writing the game, you need open your text or code editor and save the file as ".html" file. If you use something like "Atom code editor", by saving your file before hand, it will help you when you writing the code as Atom will highlight your code. Now, once you have your html file, we need to write some html code. Now, put this code in your code editor.
<!DOCTYPE html>
<html>
<head>
<title>[DIY Game Series]</title>
</head>
<body>
<script>
(html comment removed: Here is were we will put the javascript code for the game )
</script>
</body>
</html>
Step 2 : Execute function with onload event.
We will call the function when the page is loaded. In this case we will start the game when the html page is load. Let's call the function "startGame". So, to do this we add onload="startGame()"
in the body tag. Checkout the the code below. Now, our previous html file become like this:
<!DOCTYPE html>
<html>
<head>
<title>[DIY Game Series]</title>
</head>
<body onload="startGame()">
<script>
(html comment removed: Here is were we will put the javascript code for the game )
</script>
</body>
</html>
If you run the code above, there would be nothing happen because we do not do anything to our function yet. So now, let's create the function. To do that, we will put all of our javascript code inside the <script></script>
tag.
Step 3 : Writing the game.
There will be a couple function that we need here. First let's write our startGame()
function. For now, do not worry about anything else, just write the code as follow:
function startGame() {
windowArea.start();
}
Once you write the function above, now we need to add another function to create the area of the game. In this case, we need to create a canvas as the area of our game. To do that, we can add the following function.
var windowArea = {
canvas : document.createElement("canvas"),
start : function() {
this.canvas.width = 480;
this.canvas.height = 270;
this.context = this.canvas.getContext("2d");
document.body.insertBefore(this.canvas, document.body.childNodes[0]);
}
}
Once you run the code above, there are still nothing to display. This is happened because our canvas in still invisible but it is already there. So, let's try give it a style little bit to make it visible. We can do this by using CSS. In order to add the CSS, we need to add <style></style>
tag in our html file. We will add this between <head></head>
tag. Let's give the border and background color to our canvas.
canvas {
border: 1px solid black;
background-color: grey;
}
Now if you refresh or run the code, you will see something like this:
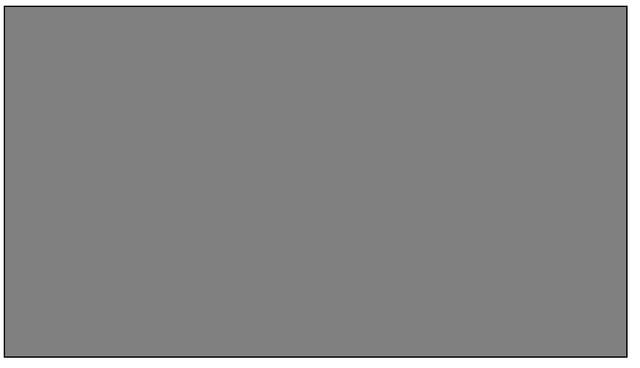
Now, we already have our game area. Next step is we need to add some character, let's add a rectangle that we will control later using keyboard. To add a rectangle image, we need to declare our rectangle, let's call it "myCharacter". We will also add a function to create this rectangle. Then, we will call this function in our "startGame()" function and pass some parameter as well about width, height, color and position of our character. So, we can do that as follow:
- Declare a variable
var myCharacter
- Add the following function
function character(width, height, color, x, y) {
this.width = width;
this.height = height;
this.x = x;
this.y = y;
ctx = windowArea.context;
ctx.fillStyle = color;
ctx.fillRect(this.x, this.y, this.width, this.height);
}
- Next, add the following code inside
startGame()
function.myCharacter = new character(40, 40, "yellow", 12, 122);
See that parameter, 40x40 is the size of our character, yellow is the color and 10, 120 is going to be the position.
Now after you add the function above, if you refresh or run the code, you will see something like this:
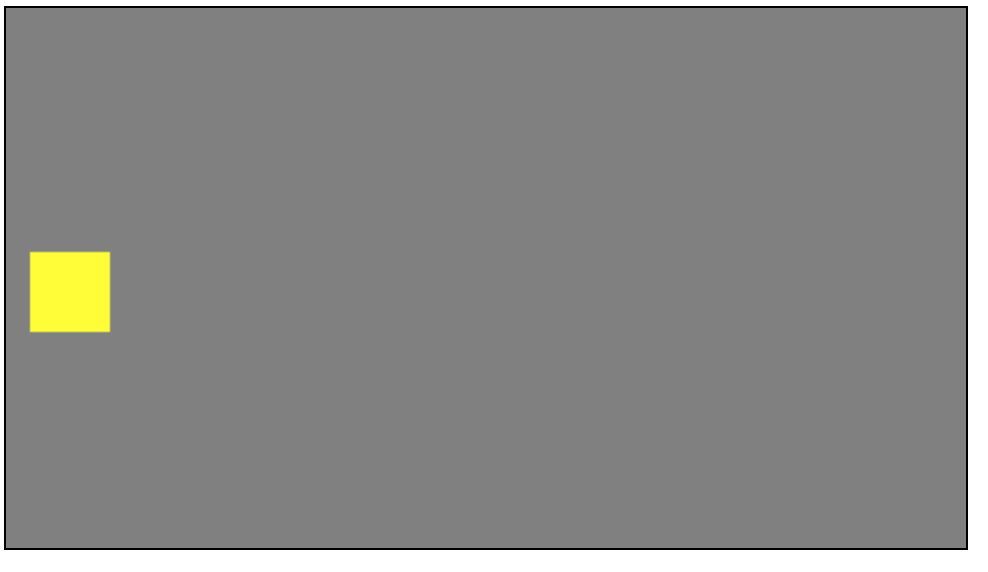
Next, we already have our character and window area. So, now let's make it more interesting by controlling our character with keyboard. When the key in the keyboard is pressed, our green character will move. To control our character, there are several thing we can use. Here I am going to show you to use keyboard as the controller. We are going to move our character up and down. So, we will use up and down arrow keys. To do that, we need to add the following function.
function updateGameArea() {
windowArea.clear();
myCharacter.speedX = 0;
myCharacter.speedY = 0;
if (windowArea.key && windowArea.key == 38) {myCharacter.speedY = -1; } //up
if (windowArea.key && windowArea.key == 40) {myCharacter.speedY = 1; } //down
myCharacter.newPos();
myCharacter.update();
}
Once we add the function above, we also need to update our code in windowArea
to add an event listener.
window.addEventListener('keydown', function (e) {
windowArea.key = e.keyCode;
})
window.addEventListener('keyup', function (e) {
windowArea.key = false;
})
},
Then we also need to add the code below in var windowArea
as well.
clear : function(){
this.context.clearRect(0, 0, this.canvas.width, this.canvas.height);
}
Now if you run the code you will able to control the the yellow rectangle up and down by pressing up and down arrow keys.
Alright, that is all for today, I will continue this article in part 2. In part 2, we will create another object that act as the obstacle to our character.
Now, here is the final code of our html file should be.
<!DOCTYPE html>
<html>
<head>
<title>[DIY Game Series]</title>
<style>
canvas {
border:1px solid black;
background-color: grey;
}
</style>
</head>
<body onload="startGame()">
<script>
var myCharacter;
function startGame() {
windowArea.start();
myCharacter = new character(40, 40, "yellow", 12, 122);
}
var windowArea = {
canvas : document.createElement("canvas"),
start : function() {
this.canvas.width = 480;
this.canvas.height = 270;
this.context = this.canvas.getContext("2d");
document.body.insertBefore(this.canvas, document.body.childNodes[0]);
this.interval = setInterval(updateGameArea, 20);
window.addEventListener('keydown', function (e) {
windowArea.key = e.keyCode;
})
window.addEventListener('keyup', function (e) {
windowArea.key = false;
})
},
clear : function(){
this.context.clearRect(0, 0, this.canvas.width, this.canvas.height);
}
}
function character(width, height, color, x, y) {
this.areaofthegame = windowArea;
this.width = width;
this.height = height;
this.speedX = 0;
this.speedY = 0;
this.x = x;
this.y = y;
this.update = function() {
ctx = windowArea.context;
ctx.fillStyle = color;
ctx.fillRect(this.x, this.y, this.width, this.height);
}
this.newPos = function() {
this.x += this.speedX;
this.y += this.speedY;
}
}
function updateGameArea() {
windowArea.clear();
myCharacter.speedX = 0;
myCharacter.speedY = 0;
if (windowArea.key && windowArea.key == 38) {myCharacter.speedY = -1; } //up
if (windowArea.key && windowArea.key == 40) {myCharacter.speedY = 1; } //down
myCharacter.newPos();
myCharacter.update();
}
</script>
</body>
</html>
Reference : Here
Remember that I write the article as my experience in learning javascript. If you want to learn more about javascript and html game, you can go to the original source as the link in the reference.
In next part, we will learn to add the gameplay to our game.
Please follow for me @zulman for my next article.
Ayyyy we got a gaming community goin, come for the support! Check us out at @peopleofthenight and join our Discord!
https://discord.gg/5sqeX95
This is spectacular work! Please keep making these.
Thankyou @reverbtank
Wow great post, thanks for sharing this!!
Thanks @ilhuna