Solution To Reggaemuffin's Coding Challenge - Python + Code Explanation and Logic
FizzBuzz
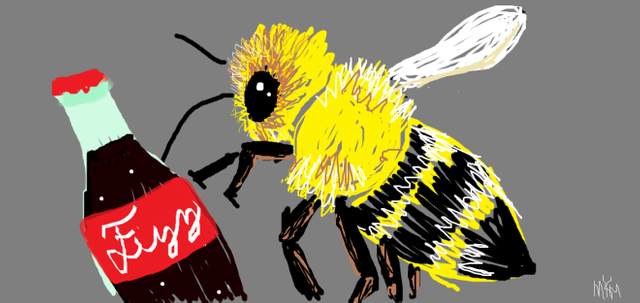
After coming across a post from @reggaemuffin where he hosted a coding challenge where I submitted a pretty amateur solution in Python he insisted that I make a separate post where I could explain my thinking process and the logic behind it. It's not a very difficult challenge but it's a pretty nice one to try for the sake of learning and potentially teaching others. So here's the rules to the challenge:
The fist thing that should pop up into your head when you hear the word 'multiples' is the modulo operator(%). So what exactly is the modulo operator I hear you ask? Well thanks for asking. We can use the modulo operator to return the remainder of a division. For example, 8%5 will give us an answer of 3 since 5 goes into 8 once and 3 is left which is the remainder. When one number is fully dividable by another a remainder of 0 is left. This way we can determine if a number is a multiple of another by dividing the two numbers using the modulo operator and getting a remainder of 0. Amazing right? Not really but it's perfect for this challenge so we'll be using it. Let's get to the the code.
For the sake of explaining I made my solution a little longer and more manageable than this gloriously ugly one line solution I found online.
Code Explanation
On line 1 we start by creating our function that will do all the heavy work for us. We add a parameter for 'n' which will be used as a variable storing the number that we want do some calculations with in order to determine if its a multiple of 3, 5 or both.
On line 3 we start our try-except which we'll use to handle value errors such as when a string is passed to our function since we can't divide a word by n dumber. duh
From lines 4 - 11 we have all of our conditional statements which will help us determine if we should either print 'Fizz', 'Buzz', 'FizzBuzz' or if you should just return the number. We check if n is a multiple of 5 and 3 first otherwise if we checked if n was a multiple of 3 or 5 first there's a definite change that we'll miss a FizzBuzz since if we find a multiple of 3 or 5 first the statement will result in true and the rest of the statements will be skipped. We store our word in a variable called test which we return on line 13.
On line 15 and 16 we return an error message in case someone tried to send a string or any other datatype with which we can not do calculations with.
On lines 18 - 20 we define our main function which will be called by the function on lines 22 and 23. In our main function we have a for loop which will call our fizzbuzz(n)
function 100 times passing it an integer from 0 - 100 as it loops through the integer range of 0 -100 and print out the returned text variable that contains our words or the integer in case it's not a multiple of 3 or 5.
Please Note: This is obviously not the shortest most efficient solution out there. I wrote it this way in order to help show people the thinking process as well as the logic behind solving a problem like this. I hope you learned something :)
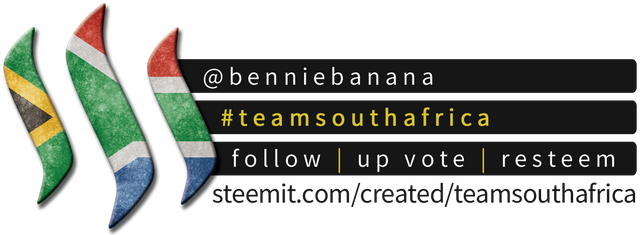
Team South Africa banner designed by @bearone
Nice clean solution. One improvement could be to concatenate fizz and/or buzz to an initially empty string. That way you only need the checks for % 3 and % 5, and the check for % 15 will come automatically as a result of those two.
Yes you're right, thanks for the feedback and the advice, appreciated. There's a few ways of doing this, even with one single line of code but I wrote it this way just to explain the underlying logic behind it for people who are trying to learn. I'm still pretty new to Python as well, trying to teach people as I learn the Python ways :)
This is an excellent post ! Absolutely brilliant.
I'm Upvoting this.