[#2] How to control Hue Lights | Philips Hue - C# Programming
How to control Hue Lights
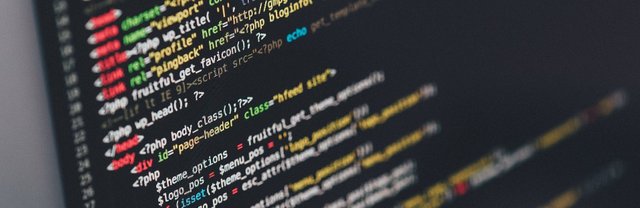
In the last tutorial I showed how to connect to the Philips Hue bridge.
[#1] How to connect to Hue Bridge
This time we will start the real fun! Controlling the Lights!
As we learned in the last tutorial everything is working with normal Get/Put/Post calls.
In the last tutorial I showed you the PostRequest. For Get and Put I created two basic requests:
private static string GetRequestToBridge(string fullUrl)
{
HttpWebRequest request = (HttpWebRequest)WebRequest.Create(fullUrl);
using (HttpWebResponse response = (HttpWebResponse)request.GetResponse())
using (Stream stream = response.GetResponseStream())
using (StreamReader reader = new StreamReader(stream))
return reader.ReadToEnd();
}
private static void PutRequestToBridge(string fullUri, string data, string contentType = "application/json", string method = "PUT")
{
using (var client = newSystem.Net.WebClient())
{
client.UploadData(fullUri, method, Encoding.UTF8.GetBytes(data));
}
}
Basic stuff 😉
Now, as we have a connection to the bridge, we can ask it for the connected lights with a Get Call.
First create a template for the url:
private const string LightsUrlTemplate = "http://{0}/api/{1}/{2}";
Call the basic get request with the created templated. You need the bridge url, Usercode (from Config, showed in the last tutorial) and the command - "lights"
string result = GetRequestToBridge(string.Format(LightsUrlTemplate, BridgeIP, Usercode, "lights"));
As result you will get the light list as string (in json format).
I created a LightHelper Class to serialize it (using this tool http://json2csharp.com/):
using System;
usingSystem.Net;
using System.Collections.Generic;
using Newtonsoft.Json;
namespace NAMESPACE
{
public partial class LightHelper
{
public string ID { get; set; }
[JsonProperty("state")]
public State State { get; set; }
[JsonProperty("swupdate")]
public Swupdate Swupdate { get; set; }
[JsonProperty("type")]
public string Type { get; set; }
[JsonProperty("name")]
public string Name { get; set; }
[JsonProperty("modelid")]
public string Modelid { get; set; }
[JsonProperty("manufacturername")]
public string Manufacturername { get; set; }
[JsonProperty("capabilities")]
public Capabilities Capabilities { get; set; }
[JsonProperty("uniqueid")]
public string Uniqueid { get; set; }
[JsonProperty("swversion")]
public string Swversion { get; set; }
[JsonProperty("swconfigid")]
public string Swconfigid { get; set; }
[JsonProperty("productid")]
public string Productid { get; set; }
}
public partial class Swupdate
{
[JsonProperty("state")]
public string State { get; set; }
[JsonProperty("lastinstall")]
public string Lastinstall { get; set; }
}
public partial class State
{
[JsonProperty("on")]
public bool On { get; set; }
[JsonProperty("bri")]
public long Bri { get; set; }
[JsonProperty("hue")]
public long Hue { get; set; }
[JsonProperty("sat")]
public long Sat { get; set; }
[JsonProperty("effect")]
public string Effect { get; set; }
[JsonProperty("xy")]
public List<double> Xy { get; set; }
[JsonProperty("ct")]
public long Ct { get; set; }
[JsonProperty("alert")]
public string Alert { get; set; }
[JsonProperty("colormode")]
public string Colormode { get; set; }
[JsonProperty("mode")]
public string Mode { get; set; }
[JsonProperty("reachable")]
public bool Reachable { get; set; }
}
public partial class Capabilities
{
[JsonProperty("streaming")]
public Streaming Streaming { get; set; }
}
public partial class Streaming
{
[JsonProperty("renderer")]
public bool Renderer { get; set; }
[JsonProperty("proxy")]
public bool Proxy { get; set; }
}
public partial class LightHelper
{
public static List<LightHelper> FromJson(string json)
{
var dict = JsonConvert.DeserializeObject<Dictionary<string, LightHelper>>(json, ConverterLight.Settings);
var result = new List<LightHelper>();
foreach (var item in dict)
{
item.Value.ID = item.Key;
result.Add(item.Value);
}
return result;
}
}
public static class SerializeLight
{
public static string ToJson(this LightHelper self) => JsonConvert.SerializeObject(self, ConverterLight.Settings);
}
public class ConverterLight
{
public static readonly JsonSerializerSettings Settings = new JsonSerializerSettings
{
MetadataPropertyHandling = MetadataPropertyHandling.Ignore,
DateParseHandling = DateParseHandling.None,
};
}
}
Now you can just call ".FromJson(result)" with the string result und you will get a beautiful List of Lights 😃
Before we make the Put call we have to create the data object for the call.
Here is the helper Class (created with http://json2csharp.com/):
using System;
usingSystem.Net;
using System.Collections.Generic;
using Newtonsoft.Json;
namespace HueTestForms
{
public partial class ColorLightHelper
{
[JsonProperty("transitiontime")]
public int Transitiontime { get; set; }
[JsonProperty("on")]
public bool On { get; set; }
[JsonProperty("sat")]
public long Sat { get; set; }
[JsonProperty("bri")]
public long Bri { get; set; }
[JsonProperty("hue")]
public long Hue { get; set; }
public ColorLightHelper(int transitiontime, bool on, long sat, long bri, long hue)
{
Transitiontime = transitiontime;
On = on;
Sat = sat;
Bri = bri;
Hue = hue;
}
}
public partial class ColorLightHelper
{
public static ColorLightHelper FromJson(string json) => JsonConvert.DeserializeObject<ColorLightHelper>(json, Converter.Settings);
}
public static class SerializeColorLightHelper
{
public static string ToJson(this ColorLightHelper self) => JsonConvert.SerializeObject(self, Converter.Settings);
}
public class ConverterColorLightHelper
{
public static readonly JsonSerializerSettings Settings = new JsonSerializerSettings
{
MetadataPropertyHandling = MetadataPropertyHandling.Ignore,
DateParseHandling = DateParseHandling.None,
};
}
}
Lets create the Data:
var data = new ColorLightHelper(0, true, 254, 254, 1000).ToJson());
0 = transaction time - how fast the color/state change should happen. smooth change = high value | fast change = low value
bool state
sat 254(max) = saturation
bri 254(max) = brightness
hue 1000 = measure of color - 1000 is red
Now we just have to call the PutRequestToBridge from the start of this tutorial.
First you will need a new url Template:
private const string ControlLightUrlTemplate = "http://{0}/api/{1}/{2}/{3}/{4}";
You need the bridge IP, the Usercode, the command "lights", the Id of the light you want to control (take it out of your LightHelper list) and the State "state". Add the data and go!
PutRequestToBridge(string.Format(ControlLightUrlTemplate, BridgeIP, Usercode, "lights", lightId, "state"), data);
If the light is red now everything is working!
if you have any questions do not hesitate to contact me per comment :-)
This post has received a 1.36 % upvote from @booster thanks to: @geggi632.
This post has received a 3.36% upvote from
thanks to: @geggi632.
For more information, click here!!!!
Send minimum 0.100 SBD to bid for votes.
Do you know, you can also earn daily passive income simply by delegating your Steem Power to @minnowhelper by clicking following links: 10SP, 100SP, 500SP, 1000SP or Another amount