Variables & Naming Them in Python
As the name indicates, a variable is something which can be changed later. Let’s suppose your teacher tells you a number containing 8 digits and asks you to remember for the next five days. If you don’t have a good memory like me, you can write those 8 digits on a piece of paper or may send a text to yourself. The main idea behind this is to save the number and retrieve it whenever your teacher asks for it. A variable in a computer program is just like that, you assign a value/data to a symbolic identifier that can be retrieved any time within a program. It’s just like a container, you can save values/data, retrieve them or even change them later in your program.
In Python, unlike the other programming languages like Java, C or C++, you don’t have to declare variable types before using them, it’s actually quite simple in Python. You just think of a name, start using it as a variable and change its data type whenever you want. Let’s create our first variable.
>>> num = 100
num is the name of our variable, you can name it whatever you want. The equal “=” is an assignment operator. Remember that in Python, it shouldn’t be read as “is equal to”, you can say “is set to”. This assignment operator helped us store 100 in our num variable. So in short, “the numvariable is set to 100” or you can say the variable named num was tied to the value 100. Let’s print our first variable and see the output.
>>> num = 100 >>> num 100
If you want to check the type of data we stored in the num variable, just pass your variable in the type function like this
>>> type(num) <class 'int'>
So we stored an integer in our variable, let’s change the type and assign a string “hundred” to our num variable
>>> num = 100 >>> num 100 >>> type(num) <class 'int'> >>> num = "Hundred" >>> num 'Hundred' >>> type(num) <class 'str'>
Now the type of our variable has changed to a string, let’s play again with our variable and now store a floating point number in our num variable.
>>> num = 100 >>> num 100 >>> type(num) <class 'int'> >>> num = "Hundred" >>> num 'Hundred' >>> type(num) <class 'str'> >>> num = 55.5 >>> num 55.5 >>> type(num) <class 'float'>
Object References
Let’s go into some technical details and see what happened above.
In Python, variables are references to objects and the actual value or data is stored in the objects. In our example, our variable num was a reference to an integer object. Later we changed the object to a string and then our num variable was referencing to a new object that was a string. Note that as soon as we changed the object, our variable num deleted the previous object (int) and started referencing to a new object, in our case that was a string. Same happened when we changed our object from string to a floating point number. Again, our num variable just removed the old object (string) and stored a new type of object (float).
Let’s create two variables, one is num1 and the other is num2, assign a value 100 to num1 and set num2 to num1 like this:
>>> num1 = 100 >>> num2 = num1 >>> num1 100 >>> num2 100
The output will be 100 & 100, in this case, both variables are referencing to the same int object. You can confirm it by passing both these variables to the identity function (id())
>>> num1 = 100 >>> num2 = num1 >>> num1 100 >>> num2 100 >>> id(num1) 140712306146144 >>> id(num2) 140712306146144
You can see that both these variables refer to the same object but once you change the value of any of these variables, a new object will be created.
>>> num1 = 100 >>> num2 = 101 >>> num1 100 >>> num2 101 >>> id(num1) 140712306146144 >>> id(num2) 140712306146176
Multiple Assignment
In Python, you can use a single value to multiple variables. This helps you to initialize several variables at a time which you can change later in your program.
>>> name = name1 = name2 = "Richard" >>> name 'Richard' >>> name1 'Richard' >>> name2 'Richard'
Or if you want to assign multiple values to multiple variables in one statement (using Tuple Assignment)
>>> name, num, my_float = "Richard", 24, 24.2 >>> name 'Richard' >>> num 24 >>> my_float 24.2
In the above example, the variable name was assigned to the string “Richard”, the num variable was assigned to the integer 24 and my_float variable was assigned to the float 24.2. This will only work if the number of elements on the left side of the assignment operator is equal to the values on the right side, otherwise, it will result in an error.
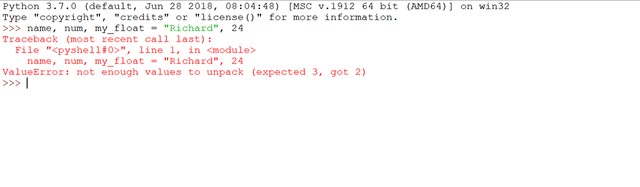
Deleting A Variable
You can delete a variable in Python using del keyword. del stands for delete and once you delete a variable, its value can’t be retrieved later in the program.
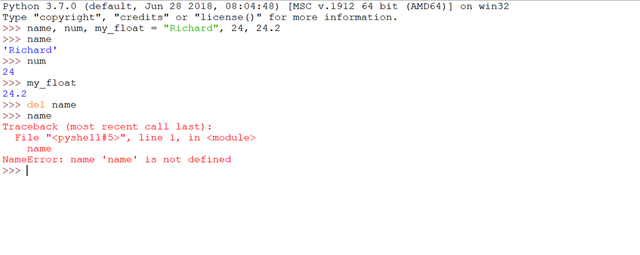
Naming Rules
Here are some of the guidelines that you should follow while naming a variable
- Your variable must only have one word with no spaced.
- You can use underscore (_) (my_name) or CamelCase notation (MyName) for variables having more than one word.
- You can use upper case letters “A” to “Z” or lower case letters “a” to “z”.
- Your variable must start with a letter or an underscore (_).
- You can’t use hyphens (-).
- Your variable shouldn’t start from a number, however, you can use numbers in the remainder of a variable.
- You can’t use symbols like %,$,#
- Variables in Python are case sensitive, the variable bob is different from the variable called Bob.
- Try to use descriptive names for your variables instead of using single characters.
- You can’t name your variable the same name as one of the Python keywords. Here is the list of all Python Keywords
False class from or None continue global pass True def if raise and del import return as elif in try assert else is while async except lambda with await finally nonlocal yield break for not
You will get an error if you use any of these keywords as a variable in your program.
>>> def = 100 SyntaxError: invalid syntax
Posted from Data Science With Python SteemPress : http://datasciencewithpython.info/variables-naming-them-in-python/
Hello! Your post has been resteemed and upvoted by @ilovecoding because we love coding! Keep up good work! Consider upvoting this comment to support the @ilovecoding and increase your future rewards! ^_^ Steem On!
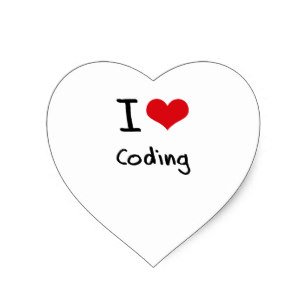
Reply !stop to disable the comment. Thanks!
@ghulammujtaba, go and place your daily vote for Steem on netcoins! http://contest.gonetcoins.com/
Congratulations @ghulammujtaba! You received a personal award!
You can view your badges on your Steem Board and compare to others on the Steem Ranking
Do not miss the last post from @steemitboard:
Vote for @Steemitboard as a witness to get one more award and increased upvotes!