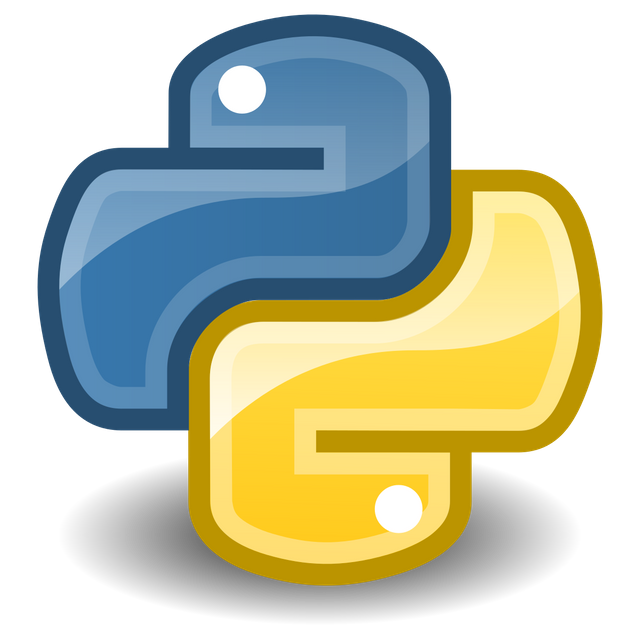
For Part 5 of my tutorial I will show you how to use IF, ELSE, and ELIF statements.
Same as last time, open up our preferred IDE (Integrated Development Environment)
We will also open up our previous program, "myprogram.py"
Your program should look like this:
def start(inputStr):
print("Hey from inside my function!")
print("Here is your input: " + inputStr)
print("Hello World!")
x = input("Input needed: ")
start(x)
IF and ELSE statements are very useful and fundamental.
They allow you to compare things, and run commands based on a user input.
If we are going to make a program with IF/ELSE then we need to have a function for our program.
I don't mean function like in the last tutorial, I mean what do we want our program to do.
Here's an idea, we make a basic password login.
The user will enter their password, and we will tell them if it is right or not.
If it is right, they will get let into the program, or whatever we want to do.
If it is wrong, give them three tries, and tell them how many tries they have left.
So let's clean up our program to make room for this function.
print("Please Login:")
x = input("Password: ")
We still haven't added the basic IF statement to check for the password.
First we go over the basic syntax of IF and ELSE:
if (something == somethingElse):
doSomething()
elif(something > somethingElse):
doSomethingElse()
else:
print("Nothing Happened?")
That is the basic syntax, here is a real example:
from random import randint
x = randint(1,3)
y = randint(1,3)
print("X is: ", x)
print("Y is: ", y)
if (x == y):
print("X is equal to Y")
elif(x > y):
print("X is greater")
elif(x < y):
print("Y is greater")
else:
print("Error: Please Try Again")
This generates a random number between 1 and 3 and assigns it to the variable x and y. Then it checks if they are greater, less than, or equal to each other.
If you run it a few times you get different outputs.
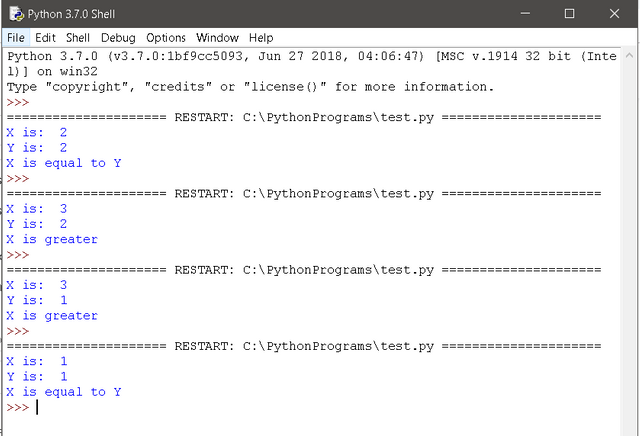
Here is a flow algorithm representing IF and ELSE
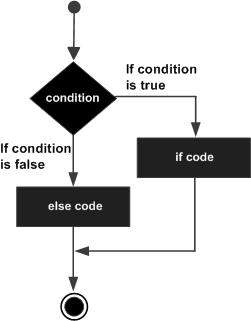
With all of this in mind we can start to create our program.
password = "Password123"
print("Please Login:")
x = input("Password: ")
if(inputStr == password):
print("Password Correct")

Now that that works, lets add a statement to check if the password is false.
password = "Password123"
print("Please Login:")
x = input("Password: ")
if(inputStr == password):
print("Password Correct")
elif(inputStr != password):
print("Password Incorrect")
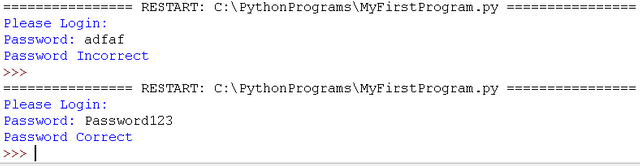
That works, we also wanted to implement a 3 try system right? We can acomplish this with a loop.
The while loop is like a repeating IF statment. Its name is what it does.
While x == true then iterate Something until x == false
So we can implement this into our code like this:
password = "Password123"
attempt = 0
while(attempt < 3):
print("Please Login:")
x = input("Password: ")
if(x == password):
print("Password Correct")
elif(x != password):
attempt = attempt + 1
print("Password Incorrect")
print("Attempt Number " + str(attempt))
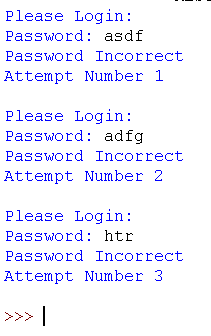
Now the program just ends even if we get the password right. We can fix this by adding some booleans that tell the program if the password is true or false
password = "Password123"
attempt = 0
while(attempt < 3):
print("Please Login:")
x = input("Password: ")
if(x == password):
passwordCorrect = "true"
print("Password Correct")
attempt = 3
elif(x != password):
passwordCorrect = "false"
attempt = attempt + 1
print("Password Incorrect")
print("Attempt Number " + str(attempt) + " \n")
if(passwordCorrect == "true"):
print("Welcome back!")
elif(passwordCorrect == "false"):
print("You have been locked out for too many tries. \n Contact your administrator.")
else:
print("Error: Please Try Again")
This will have 2 outputs.
Password Incorrect:
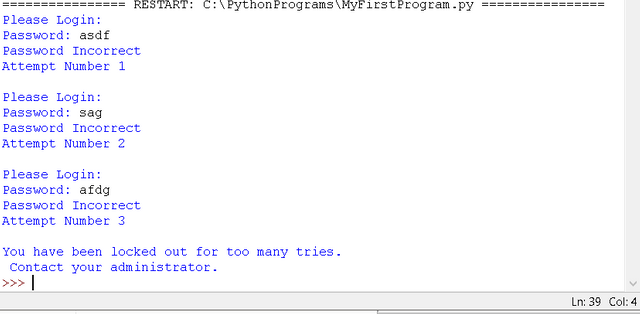
Or Password Correct:
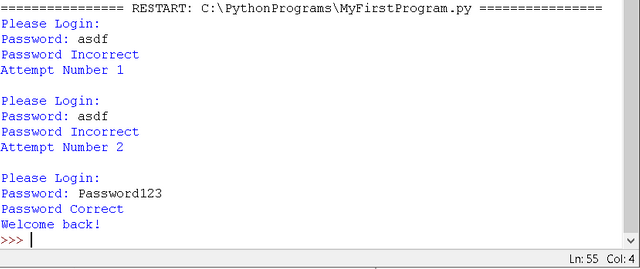
Today I showed you how to use IF and ELSE statements in python.
You also learned how to create a basic Password checker from scratch.
I hope you enjoyed this post, if you did please upvote and follow me (it helps!)