Simple Intellij Java GUI example/tutorial
Preface
GUI is important part of programming, so let's get into it. In this tutorial i'll show how to add buttons,dialogs, menu bar and make shortcuts to menu items. The final version of our GUI program would look something like this:
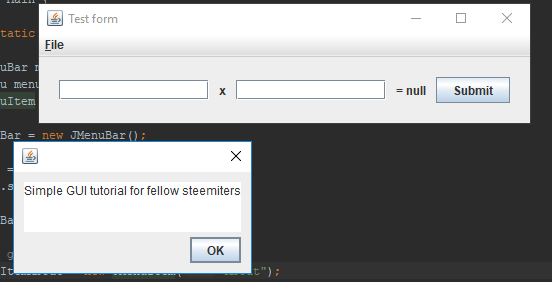
It will multiple two numbers and show an answer in the label.
Body
Create a new project in IntelliJ with Command Line app template , because it will give us a simple template with Main.java class. Then create GUI form for our project like this :
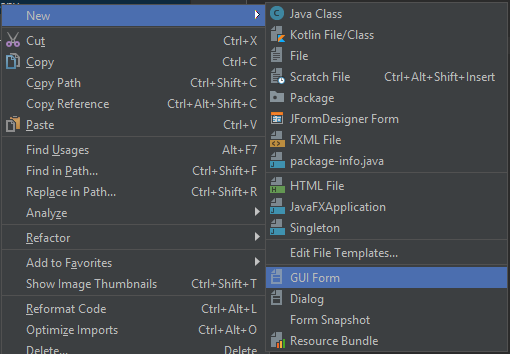
Give JPanel object a name, because we will need it for creating a JFrame object (a frame for our program).
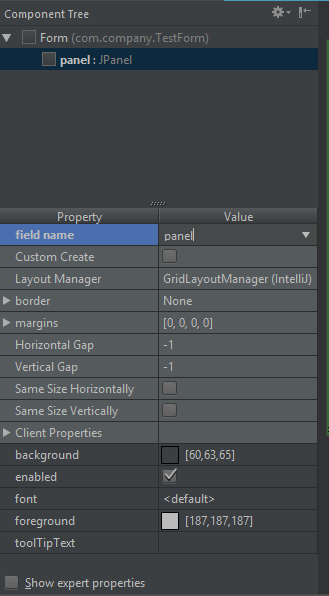
We should add a getter method for a panel we named, because in our Main class we will create a JFrame object, which requires a JPanel object to work. More information why use set and get methods you can find here : https://stackoverflow.com/questions/6638964/set-and-get-methods-in-java
So for now our GUIForm class which i named TestForm should look like this:
package com.company;
import javax.swing.*;
public class GUIForm {
private JPanel panel;
public JPanel getPanel(){
return panel;
}
}
Lets create JFrame object in our Main.java class.
//...
GUIForm form = new GUIForm();
JFrame frame = new JFrame("Your window name");
frame.setContentPane(new TestForm().getJPanel());
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.pack();
frame.setVisible(true);
//...
After that you should get something like this :
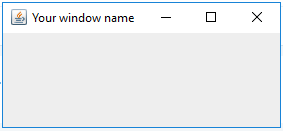
So, pretty much nothing ? Let's add some textfields, labels and a button from a pallete.
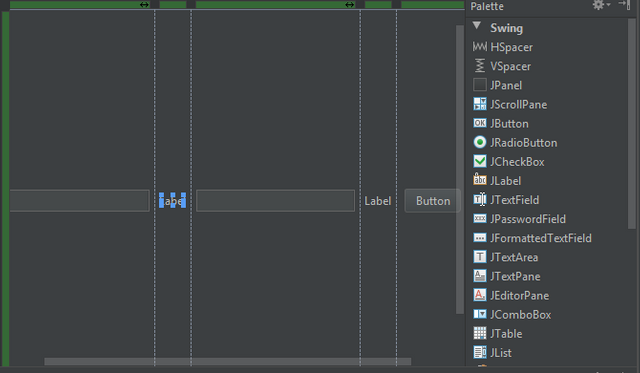
Don't forget to add margins to our panel, so it would be more visually pleasing. After running our program, we get this:

Looks cool right? Unfortunalety it does nothing, we need to add functionality to our GUI. Let's rename variables to more appropriate names like variable1, variable2, answerLabel and submitButton, do it in the GUI editor.
Let's add set and get methods. Information about and why use set and get methods : https://stackoverflow.com/questions/6638964/set-and-get-methods-in-java
Our GUIForm.java code now should look like this :
package com.company;
import javax.swing.*;
public class GUIForm {
private JTextField var1;
private JTextField var2;
private JButton submitButton;
private JPanel panel;
private JLabel answer;
public JTextField getVar1() {
return var1;
}
public JTextField getVar2() {
return var2;
}
public JButton getSubmitButton() {
return submitButton;
}
public void setAnswer(String answer) {
this.answer.setText(answer);
}
public JPanel getPanel() {
return panel;
}
}
It's time to add an action listener to our button, it's sounds like it is, action listener is basically an object which listens to occuring events in the program, in our instance it's pressing submit button.
Add this to Main.java:
form.getSubmitButton().addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
int variable1 = Integer.parseInt(form.getVariable1().
getText().toString());
int variable2 = Integer.parseInt(form.getVariable2().
getText().toString());
form.setAnswer(""+variable1*variable2);
}
});
It will add two variables from textfields in our GUI and set label's value to multiplication of those two variables.
Run your program and see if it works properly.
If everything works like intented, let's continue, we should add menu bar.
Initiation of menu and it's items in Main.java should look like this:
JMenuBar menuBar;
JMenu menu;
JMenuItem menuItemAbout, menuItemExit;
menuBar = new JMenuBar();
menu = new JMenu("File");
menu.setMnemonic(KeyEvent.VK_F);
menuBar.add(menu);
// a group of Menu items
menuItemAbout = new JMenuItem("About");
menuItemAbout.setMnemonic(KeyEvent.VK_A); // shortcut alt+A
menu.add(menuItemAbout);
menuItemExit = new JMenuItem("Exit");
menuItemExit.setMnemonic(KeyEvent.VK_X);
menu.add(menuItemExit);
It's pretty self explanatory.
Let's add dialog with textarea and action listeners to our project.
menuItemAbout.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
About dialog = new About();
dialog.pack();
dialog.setVisible(true);
}
});
menuItemExit.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
System.exit(0);
}
});
And that's it, you have a simple functional GUI, good luck creating way more difficult programs. ;)
Project code : https://github.com/Worll/SimpleGUIJavaTutorial.git