Python Tip #1: Line by line debugging in Python with ipdb module
Hello my steemian friends!
I want to share with you the first tip of this Python Tip series I'm beginning. This surely is the best way to debug code in Python without the use of any IDE, executing one by one each line in your code, been able to see or change the value of any variable in your code in runtime!
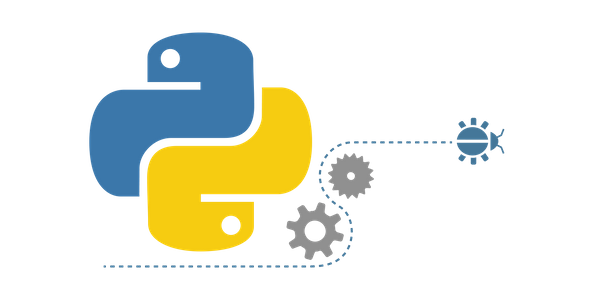
Well, here is how it works:
You just need to import the "pdb" module, an then call pdb.set_trace() in any line of your code you want to start debugging, and it's done. Yeah you read right that's all you need.
By example, let's suppose we have this little Python script to calculate the average age of :
age = [18, 20, 12, 37, 45]
age_number = len(age)
age_sum = sum(age)
average_age = age_sum / age_number
print("the average age is %s" % average_age)
If we want debug the previous code with pdb to see the result of each calculation, we could import "pdb" and call the "pdb.set_trace()" function in the third line by example:
import pdb
age = [18, 20, 12, 37, 45]
age_number = len(age)
pdb.set_trace()
age_sum = sum(age)
average_age = age_sum / age_number
print("the average age is %s" % average_age)
If we execute a script with this code, the execution will stop where we call the set_trace function, showing this:
-> age_sum = sum(age)
(Pdb)
Awesome!
then we could by example, print the value of age_number variable
-> age_sum = sum(age)
(Pdb) print(age_number)
5
We can even modify the value of the age_number, or create any new variable we want:
(Pdb) age_number = 6
(Pdb) new_var = "cat"
(Pdb) print(age_number)
6
(Pdb) print(new_var)
cat
(Pdb)
Now we can type "n" when we want to execute the next line of the script:
(Pdb) n
-> average_age = age_sum / age_number
(Pdb)
And type "c" if you want continue the execution of all the script:
(Pdb) c
the average age is 22
You could call also the Python function "exit()" if you instead want to permanently stop the execution of the script in that line and exit.
That's all, if you want extra commodity like autocompletion, get previous commands by typing the up arrow, nice coloring and be able to go back with the cursor in a statement, you can install "ipdb" module using pip:
pip install ipdb
and then import "ipdb" and call the set trace function in your code like this:
import ipdb
ipdb.set_trace()
pdb and ipdb has a list of useful commands and options you can use, you should also take a look of them ;)
I hope this could be helpful for you.
I will be posting more useful Python tips and tricks.
Cheers!
good post, continue to work, may your day please my friend. !!!!!
Thank you @jonel! I will :)
you are welcome @zerocoolrocker
Congratulations @zerocoolrocker! You have completed some achievement on Steemit and have been rewarded with new badge(s) :
Click on any badge to view your own Board of Honor on SteemitBoard.
To support your work, I also upvoted your post!
For more information about SteemitBoard, click here
If you no longer want to receive notifications, reply to this comment with the word
STOP
Do not miss the last announcement from @steemitboard!