Basic Python Exercises
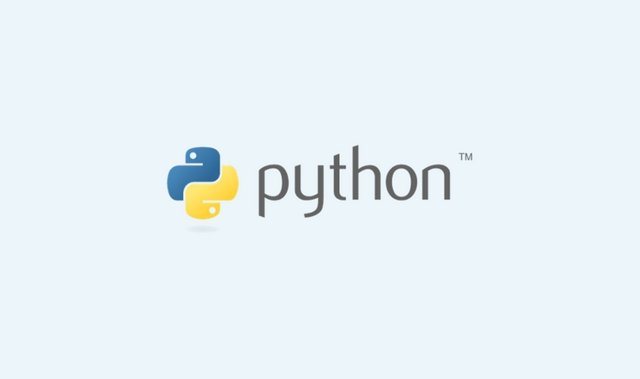
What is Python?
In my own words, Python is another Programming language that is very simple to understand and easy to code with, but the disadvantage is - it takes too long to compile the code. But yet it is easy, I will be showing you 9 Problems with Solutions you might get interested in.
So I was a little bit busy this day, I had to finish another course to be submitted this week, and I had a lot of spare time so why not solve the problems given from our instructor?
1st Python Exercise
Write a Python Program to check whether an alphabet is a vowel or consonant.
Solution
l = input("Input any letter: ")
if l in ('e'):
print("%s is a vowel." % l)
if l in ('i'):
print("%s is a vowel." % l)
if l in ('o'):
print("%s is a vowel." % l)
if l in ('u'):
print("%s is a vowel." % l)
else:
print("%s is a consonant." % l)
2nd Python Exercise
Write a Python program to read temperature in centigrade and display a suitable message according to temperatures.
Temp < 0 - Freezing Weather
Temp 0-10 - Very Cold Weather
Temp 10-20 - Cold Weather
Temp 20-30 - Normal Temperature
Temp 30-40 - Its Hot
Teno 40-50 - Vert Hot
Solution
i = input("What is the weather today?: ")
if i <= '0':
print("Its freezing!");
if (i <= '10') & (i >= '1'):
print("Very Cold Weather");
if (i <= '20') & (i >= '11'):
print("Just a Cold Weather");
if (i <= '30') & (i >= '21'):
print("Normal Temperature");
if (i <= '40') & (i >= '31'):
print("Its Hot");
if (i >= '40'):
print("Very Hot");
3rd Python Exercise
Write a Python Program to check whether a triangle is Equilateral, isosceles or scalene..
Solution
print("Triangle");
a = input("Enter the 1st side of the Triangle: ")
b = input("Enter the 2nd side of the Triangle: ")
`c = input("Enter the 3rd side of the Triangle: ")
if a == b == c:
print("Equilateral triangle")
elif a != b != c:
print("Scalene triangle")
else:
print("isosceles triangle")
4th Python Exercise
Write a Python Program to read the age of a candidate and determine whether it is eligible for casting his/her own vote.
Solution
print("Your Age");
age = input("Hi welcome to VALIDITY of Age for Voters, Enter your Age: ")
if (age >= '18') :
print("You are above 18 Years Old, You are eligible to Vote")
if (age <= '17') & (age >= '1'):
print("You are Underaged, you are not eligible to Vote")
if (age <= '0'):
print("That is totally invalid, your age is equal or below 0.")
5th Python Exercise
Write a Python Program to read the value of an integer m and display the value of n. n is 1 when m is larger than 0, 0 when m is 0 and -1 when m is less than 0.
Solution
print("Integer m display the value of n");
m = input("Enter the Value of M: ")
if (m>'0'):
print("The value of n = 1")
if (m=='0'):
print("The value of n = 0")
if (m<'0'):
print("The value of n = -1")
6th Python Exercise
In a barrio to make a long distance call you have to be charged according to some rates that differ according to the duration of your call. Develop a running Python program that computes the cellular phone bill with the following conditions: Rate = 20.0php per minute for the first 0 - 3 mins, succeeding minutes will be charged 4.00 minute. The program will ask the duration of the call in minutes and displays the bill on the screen.
Solution
print("Welcome to Call Calculator")
print("First 3 Mins will be charged 20.00php per minute")
print("The succeeding minutes will be charged 4.00 per minute")
mins = int(input("How many minutes did you call? "))
if mins <= 3:
total = (20 * mins);
print("You need to pay = ", total)
if mins >=4:
totalextend = 60 + (4 * (mins - 3));
print("You need to pay = " , totalextend)
7th Python Exercise
XYZ Telegram Company charges 18.5 pesos for telegrams that do not exceed 12 words and 1.50 pesos for every succeeding word, write a program that accepts the number of words in a telegram, compute and display the charge.
Solution
string=input("Enter string:")
char=0
word=1
comment: case and space sensitive
for i in string:
if(i==' '):
word=word+1
print("Number of words in the message:")
print(word)
comment: word exceeded to 12
if word >= 13:
newword = 18.5 + (1.5 * (word - 12));
print("Exceeded above 12 words on your message, you need to pay")
print(newword,"PHP")
#word pay not exceeded
else:
print("Pay 18.50PHP")
8th Python Excercise
Write a program that determines the quadrant, given a user-input angle. If the angle is exactly 0, it is not in the quadrant but lies on the positive x-axis, if it is exactly 90 it lies in the postive Y-axis and so on.
Solution
print("Welcome to Quadrant Pointer")
angle = int(input("Input Angle: "))
if (angle >=1) & (angle <= 89):
print(angle,"degrees lie in Quadrant I.")
if (angle >=91) & (angle <= 179):
print(angle,"degrees lie in Quadrant II.")
if (angle >=181) & (angle <=269):
print(angle,"degrees lie in Quadrant III.")
if (angle >=271) & (angle <=359):
print(angle,"degrees lie in Quadrant IV.")
if (angle == 0) | (angle == 360):
print(angle,"lie in the x + axis.")
if (angle == 90):
print(angle,"lie in the y + axis.")
if (angle == 180):
print(angle,"lie in the x - axis.")
if (angle == 270):
print(angle,"lie in the y - axis.")
9th Python Exercise
Write a Python program to read integers from 0-5 then display it in words. (take note that only 0-5)
Solution
print("Welcome to Word to Number Python Program")
num = input("Input Number: ")
if num == '0':
print("Zero")
if num == '1':
print("One")
if num == '2':
print("Two")
if num == '3':
print("Three")
if num == '4':
print("Four")
if num == '5':
print("Five")
if (num < '0') | (num > '5'):
print("Number not included, only 0-5.")
Sorry for this post, I posted it using wordpress so it means it is pretty messed up. Thanks for reading.
Posted from my blog with SteemPress : https://chuckunlimited.net/index.php/2018/10/14/basic-python-exercises/
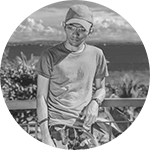
even i don't understand it XD i will try to read again @chuuuckie, nice hunt btw LOL
This aint hunt dude. xD
Hello! Your post has been resteemed and upvoted by @ilovecoding because we love coding! Keep up good work! Consider upvoting this comment to support the @ilovecoding and increase your future rewards! ^_^ Steem On!
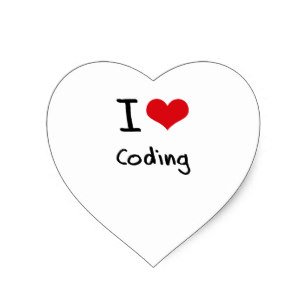
Reply !stop to disable the comment. Thanks!
Congratulations @chuuuckie! You have completed the following achievement on the Steem blockchain and have been rewarded with new badge(s) :
Click on the badge to view your Board of Honor.
If you no longer want to receive notifications, reply to this comment with the word
STOP
Do not miss the last post from @steemitboard:
@chuuuckie admired the great knowledge you possess dear friend congratulations
I wish you a prosperous week
Thanks @jlufer I appreciate your compliments :D
Congratulations @chuuuckie! You have completed the following achievement on the Steem blockchain and have been rewarded with new badge(s) :
Click on the badge to view your Board of Honor.
If you no longer want to receive notifications, reply to this comment with the word
STOP
Do not miss the last post from @steemitboard:
Hi @chuuuckie!
Your post was upvoted by @steem-ua, new Steem dApp, using UserAuthority for algorithmic post curation!
Your UA account score is currently 2.205 which ranks you at #20741 across all Steem accounts.
Your rank has improved 209 places in the last three days (old rank 20950).
In our last Algorithmic Curation Round, consisting of 279 contributions, your post is ranked at #202.
Evaluation of your UA score:
Feel free to join our @steem-ua Discord server