Pagination with PHP OOP system #3 Create a dynamic search system, Page between
Repository
https://github.com/php/php-src
What Will I Learn?
- Basic OOP Class
- Create page between
- Create a dynamic search system
Requirements
- Basic HTML
- Basic OOP
- Basic Sql Query
- Localhost (xampp, wampp, or etc)
- PHP >= 5.6
Difficulty
- Intermediate
Tutorial Contents
This tutorial is a continuation of the OOP pagination tutorial series, I suggest you follow the previous tutorial to follow this tutorial well. We will also create a page between for page number that looks better.
Create a dynamic search system
in the previous tutorial, We have created a pagination search system, but the search system we created is not dynamic because we are directly looking at the table 'username'. but in the database we have several columns:
Name | Type | Length/value | Indeks |
---|---|---|---|
id | INT | 11 | Primary |
username | Varchar | 100 | -- |
city | Varchar | 100 | -- |
Varchar | 100 | -- |
So the idea we will make the query parameter search ?search
to be dynamic, of course, we will make changes to the functions we have created before.
- Create a new variable to store column name
Because we will make the column name to be dynamic we have to store it in a variable. we will create a variable named$col
.
Example:
private $db, $table, $total_records, $limit = 5, $col;
- Create function
set_search_col()
In this function we will set the value of the variable$col
. The value is obtained from the query parameter?col
in the browser URL.
Example:
public function set_search_col(){
$this->col = $_GET['col'];
}
- to retrieve the query parameter value
?col
we can use$ _GET ['key']
. The key is col and We can access the variables inside the function with$this
.
- Run the function
set_search_col()
in __construct()
We need to run the functionset_search_col ()
the first time the class is called, therefore we will perform the function in__construct()
.
Example:
public function __construct($table){
$this->table = $table;
$this->db = new PDO("mysql:host=localhost; dbname=faker", "root", "root");
if($this->is_search()) $this->set_search_col();
$this->set_total_records();
}
- Before we run the function set_search_col () we will check with
if()
whether the query parameter?search
exists. We can know it through theis_search()
function we have created in the previous tutorial. - If the result of query parameter
?search
exists, then the result is true and automatically we will runset_search_col()
.
Noted: We have to run the function if($ this-> is_search ()) $ this-> set_search_col ();
first before running $this-> set_total_records ();
, because this affects the page number to be displayed.
- Change the query in function
We have created a function to assign a value to the $ col variable, now we can use it in the function to change the query dynamic based on the parameter query?col='colomnName'
.
1. Change the query in function set_total_records()
Example:
public function set_total_records(){
$query = "SELECT id FROM $this->table";
if($this->is_search()){
$val = $this->is_search();
// $query = "SELECT id FROM $this->table WHERE username LIKE '%$val%'";
$query = "SELECT id FROM $this->table WHERE $this->col LIKE '%$val%'";
}
$stmt = $this->db->prepare($query);
$stmt->execute();
$this->total_records = $stmt->rowCount();
}
I have given the comment syntax //
to the query that we have changed. Now we can use variable $this->col
to replace username column that we hardcode before.
2. Change the query in function get_data()
Example:
public function get_data(){
$start = 0;
if($this->current_page() > 1){
$start = ($this->current_page() * $this->limit) - $this->limit;
}
$query = "SELECT * FROM $this->table LIMIT $start, $this->limit";
if($this->is_search()){
$val = $this->is_search();
// $query = "SELECT id FROM $this->table WHERE username LIKE '%$val%' $start, $this->limit";
$query = "SELECT id FROM $this->table WHERE $this->col LIKE '%$val%' $start, $this->limit";
}
$stmt = $this->db->prepare($query);
$stmt->execute();
return $stmt->fetchAll(PDO::FETCH_OBJ);
}
I have given the comment syntax //
to the query that we have changed. Now we can use variable $this->col
to replace username column that we hardcode before.
3. Add a parameter query in the check_search()
function
We will add a new parameter query that is ?col
, we need to add the return value on the check_search()
function so that the page number, next and prev link corresponds to the new query parameter .
Example:
public function check_search(){
if($this->is_search()){
return '&search='.$this->is_search().'&col='.$this->col;
}
return '';
}
We can add a new query parameter by combining the string &col=
and the value of the variable $this->col
like this: .'&col='.$this->col;
- The Result
If there is no error, we can see the result like this.
Search by email
Looking for data that has a g character in the email field in the databasesearch=g&col=email
.
Search by city
Looking for data that has a g character in the city field in the database search=g&col=city
.
Create page between
So far our pagination system goes well. but there are certain cases if our data has reached 1000 we rather see a lot of page number that will appear of course it is not good. for that, we will create page between. So the idea we will hide some page number.I will only show 2 next pages and 2 pages previous from the current page. to handle that problem, I will create a function is_showable ()
.
- Create function
is_showable()
In this function will accept the current page parameters$num
and in this function, there will be two statements that we will check.
Example:
public function is_showable($num){
// The first statements
if($this->get_pagination_number() < 4 || $this->current_page() == $num)
return true
// The second statements
if(
($this->current_page()-2) <= $num && ($this->current_page()+2) >= $num
)
return true
}
- The first statements
1. $this->get_pagination_number() < 4 : Because we will display 2 pages next and 2 previous after then the minimum amount we will hide is 4 pages from (2 next + 2 prev = 4). We can get the total page number through the get_pagination_number()
function.
2. $this->current_page() == $num: then we create a logic operator ||
(or) for the second condition of this statement. This condition is useful for the current page the same as the $num
value will result in true. We can get the current page through the current_page()
function.
Note: This statement uses the logic ||
(or), so if one qualifies then we will return true. The true value we will use in a section of frontend to check the certain condition.
- The second statements
1. ($this->current_page()-2) <= $num: This condition will check whether the current page reduced by 2 results is smaller than(<=) the page that passed in the parameters $num
.
2. ($this->current_page()+2) >= $num: This condition will check whether the current page plus 2 results is greater than the page that is passed in the parameters $num
.
Note: This statement uses the logic &&
(and), so both conditions in this statement must be true, then the result of this statement is true. if one does not qualify then it will be false.
- Use function
is_showable ()
We have finished making the logic of this function. now we can use in the looping variable $pages. $pages = $pagination->get_pagination_numbers();
.
Example:
<? for($=i; $i<=$pages; $i++): ?>
<? if($pagination->is_showable($i)):?>
<a class="<? echo $pagination->is_active_class($i) ?>"
href="?page=<? echo $i.''.$pagination->check_search(); ?>"><? echo $i;</a>
<? endif; ?>
<? endfor; ?>
Not all pages will be displayed, so we will check first with
if(){}
, We can use the function is_showable() like this$pagination->is_showable($i)
.we pass the page number contained in the$i
variable of the loop result.
The Result
We have seen in the picture. we only show 2 next page and 2 previous page. we have done create page between. thank you for following this tutorial, may be useful for you.
Curriculum
Basic OOP, Fetch Data with PDO database
Previous page, Next page, and Active Class in PHP
Search system and dynamic query
@therealwolf 's created platform smartsteem scammed my post this morning (mothersday) that was supposed to be for an Abused Childrens Charity. Dude literally stole from abused children that don't have mothers ... on mothersday.
https://steemit.com/steemit/@prometheusrisen/beware-of-smartsteem-scam
And the reason why is because @therealwolf is disgusting slimy pedophile that enjoys abusing kids. Here's proof of him upvoting child porn on the steemit blockchain. bigbadwolf indeed.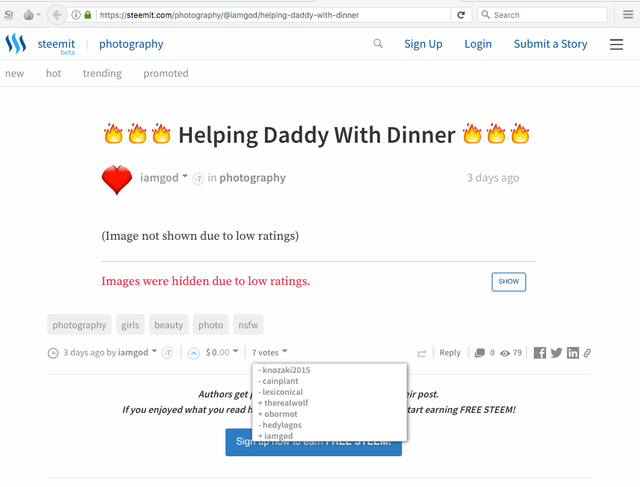
And the reason why is because @therealwolf is disgusting slimy pedophile that enjoys abusing kids. Here's proof of him upvoting child porn on the steemit blockchain. bigbadwolf indeed.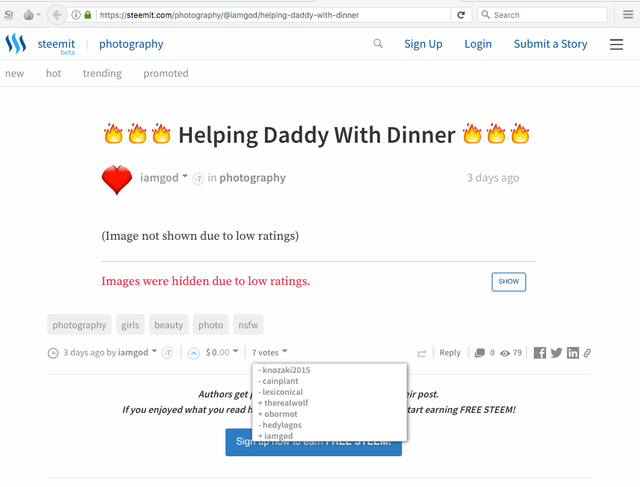
Your content is unfortunately taken from elsewhere on the web and hence falls under plagiarism. This applies to your recent contributions as well.
Your tutorials are actually taken from belajar php, which can be found under https://www.duniailkom.com/tutorial-belajar-php-dasar-untuk-pemula/
and
https://belajarphp.net/belajar-tutorial-php-mysql/
Plagiarism is a serious offense, and due to your several attempts at doing so you have now been banned from using utopian services.
Need help? Write a ticket on https://support.utopian.io/.
Chat with us on Discord.
[utopian-moderator]
Hai @mcfarhat I do not do plagiarism in my contributions, which you show absolutely no example of plagiarism in my contribution. I want further explanation. content I created myself.
https://steemit.com/coding/@gaottantacinque/rasmus-lerdorf-php-inventor-in-milan
Thanks :)
Congratulations @alfarisi94! You have completed the following achievement on the Steem blockchain and have been rewarded with new badge(s) :
Click on the badge to view your Board of Honor.
If you no longer want to receive notifications, reply to this comment with the word
STOP
Do not miss the last post from @steemitboard: