Learning Basics Of PyGame To Create A Game
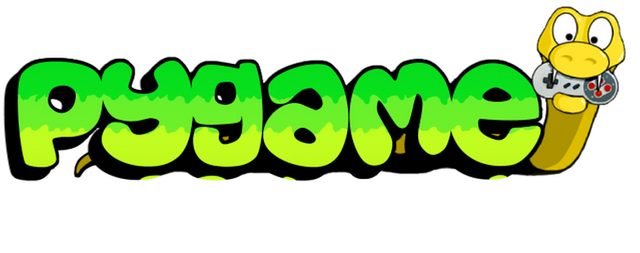
Welcome to another tutorial and in this tutorial I will be teaching you can create simple games using a module known as PyGame. I won't be going all over the PyGame in just article because If I will do that then this will become just another tiresome article to read. The first thing to understand is that what is PyGame, if we take a look at wikipedia then it says there.
Pygame is a cross-platform set of Python modules designed for writing video games. It includes computer graphics and sound libraries designed to be used with the Python programming language.
If you are familiar with coding in Python then you will absolutely love this series and let's just jump right into it!
Repository
https://github.com/pygame/pygame
What Will I Learn?
In this tutorial you will learn the following things:
- Importing PyGame and initializing it
- Learning about Surface and creating it
- Updating the display and learning about different methods of doing it
- Setting the title or caption
- Creating game loop
- Understanding events
- Learning about different kinds of events
Requirements
To find this tutorial useful you should atleast have some knowledge about Python. I will try to explain everything as clear as I can but the subjects will go all over your head if you don't have basic knowledge about Python. You will need following things installed to follow along with this tutorial
- Python Installed (Python 3.6)
- A Good Code Editor (I will use PyCharm)
- PyGame Installed (You can download it from here)
Difficulty
- Intermediate
Tutorial
Basic Set Up
Importing PyGame And Initializing The PyGame
First thing should be opening code editor and making a new new python file (.py). First line of code that we will write is import pygame
. So what this code does is that it imports the pygame module and this will also allow us to use the things from the module, such as functions inside it. The next thing that you will find yourself doing every time you make a game in Python is initializing the pygame and we can do this by typing following code in the new line pygame.init()
and as you can understand by yourself that init is a function and it is short form for "initialize".
What Is Surface In PyGame And Creating It
Now you will need to create a surface, now what surface is that it acts like a canvas. All the images and things first get drawn and then we can show those things. In the programming everything first get drawn in the back end first whether you are using Pygame, Pillow or PIL and then you can display them on your screen when ever you want. So to create a surface we will need to type game_surface = pygame.display.set_mode((800, 600))
. Let me explain it to you, first we are creating a object known as game_surface and assigning it to our pygame and we are passing a tuple which is width and height to set_mode. It is really easy to mess this up and some times people easily forgot to pass tuple instead what they did was pygame.display.set_mode (800, 600)
and you will never want to do it because we don't pass in tuple then the function will treat the 800 and 600 as two different parameters which will result in errors.
Updating The Display
Now we will need to update the display which we can do by typing pygame.display.update()
there is another very interchangeable function that you can use is pygame.display.flip()
. You can think of most games made by pygame like flipbooks they are just frames flipping/updating, The main difference between both is that .flip will update the entire surface but the .update will only update certain parts of surface that we want to. If we want we can pass in the parameters to .update() function to update only specific parts but if we will not pass any argument then it will cause it to update the entire surface. So the use of both are very same but because many people use .update() we are gonna do it too.
Setting The Title (Or Caption)
We can also change the title of the window of the game. The game which I will be building is really basic game and I am sure you might of heard about it too, it is the snake game. Do you remember the snake game in which snake eats food and it gets longer and longer and your objective it to not eat yourself or hit the edges of the window. So I will like to add title something like "Snake Game Returns" simple. I don't know why exactly but the title is known as caption in pygame. So to change title we will type pygame.display.set_caption('Snake Game Returns')
. Make sure the line of code is before updating the game so it will change the title and update the window which will result in new title. So that's the basic setup of PyGame and now under the basic setup we will have code, we will uninitialized the pygame and all sorts of stuff. Here's all the code and image how much we have done so far.
Code
import pygame
pygame.init()
game_surface = pygame.display.set_mode((800, 600))
pygame.display.set_caption('Snake Game Returns')
pygame.display.update()
Image Of Usage Of Code
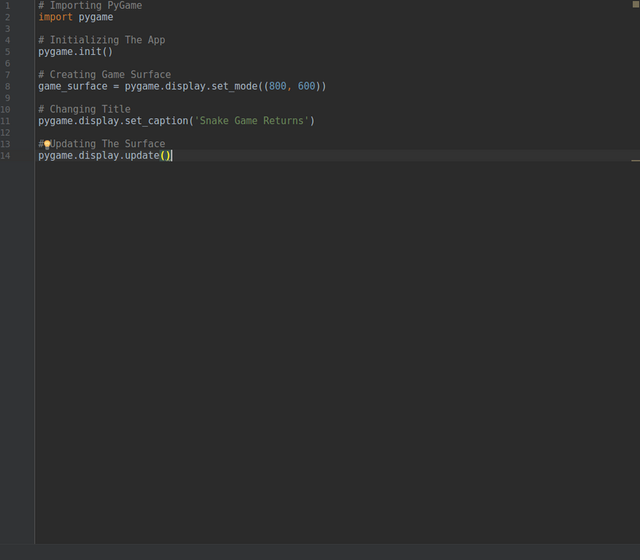
Results Of Code
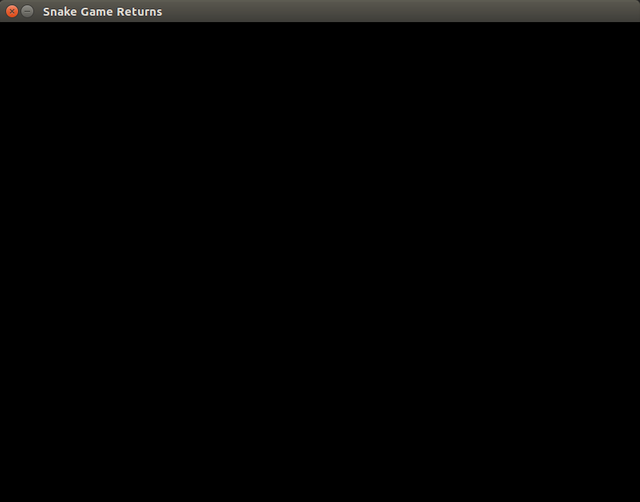
You can see it's just a big black window at the moment that is because we haven't done anything fancy yet.
Getting Into It
Creating The Game Loop
Now that we have all the basic set up let's move on to the real deal. Now we are gonna slowly move towards building the logic for the game and this is where PyGame really comes in handy. First we are gonna make a simple game loop which will handle simple functions. First we will create a variable known as game_exit and set it to False. Now we will make a so called game loop which will while game_exit is set to False. so to do this you will need to type or copy the code given below.
while not game_exit:
for event in pygame.event.get():
print(event)
Ok, what is actually happening in this while is that while game_exit is not true it prints every even that is happening in the game.
Understanding The Events
Events are very important for making a game in PyGame. We can use Events to give information to use about what the game player is doing in the window or what to do when player does certain things. To give you better idea of what events are I have uploaded a image below that is what above while loop actually, it prints everything that a player is doing or you can also say what events are trigerring. Take a look at this image.
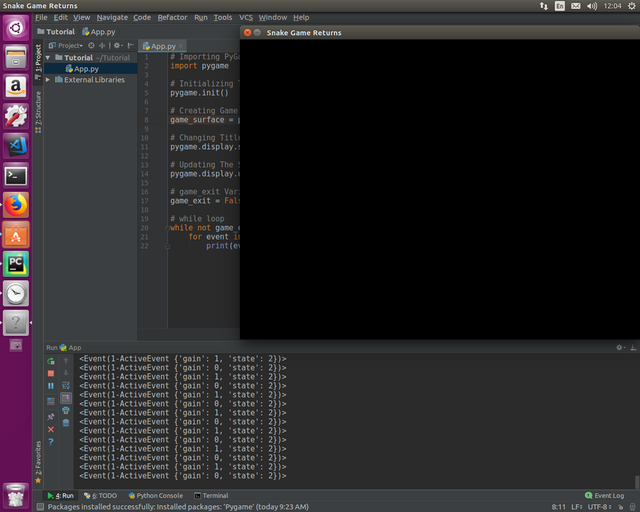
You can see our script is running and at the bottom you can see a list of events and they all are related to what I am doing. If I move my mouse inside the window then it updates, if I press any button on the keyboard then it updates. It also updates when I move the window. Basically what I am trying to say is that all the information are in event of what we are doing, how we are interacting with the window.
All The Events
Now you know what events are in PyGame but you probably don't know all the events that can happen in PyGame so we will take a look into it now. Here's the list of all the events after the list I am gonna give you a idea how those events can be helpful to us.
- Quit - This event tells us if user want to quite
- Active Event - This event tells us if the window of game is active or inactive
- Key Down - This event tells us if player has hold down any key
- Key Up - This event tells us if user has released any key
- Mouse Motion - This event tells us if mouse is moving or where our cursor is
- Mouse Button Up - This event tells us if player has released the mouse button
- Mouse Button Down - This event tells us if player is holding the mouse key
- Joy Axis Motion - This event tells us if Joy Stick's Axis is moving
- Joy Ball Motion - This event tells us if Joy Stick's Ball is moving
- Joy Button Up - This event tells us if Joy Stick's button is released
- Joy Button Down - This event tells us if Joy Stick's button is pressed
- Video Resize - This event tells us if player is resizing the window
- Video Expose - This event tells us if window is exposed to the user or not.
- User Event - Not Used
With above event you can see that PyGame also has support for Joy Stick but I won't be covering anything related to it as I want to keep my tutorial as simple as I can.
Handling The Quit Event
Now that we know what events are and we have learned about different kinds, let's actually do something with event. If you run the script that we have until now you will notice that we can't really exit the game even if we click red x at the top right if you are using Windows and at top left if you are using Linux (which I am). Now we will create a script for every time user tries to exit game, they can. Instead of printing the event now we want to check if the type of event is quit, so to do that type the following code.
if event.type == pygame.QUIT:
game_exit == True
Ok, so what above code is really doing is that whenever the user clicks on red x at top right/left, it sets game_exit to True which will cause while loop to shutdown and it will not allow us to exit the game yet because we will need to add two more lines of code but before it make sure you have placed above code under the for loop of for event in pygame.event.get(). After the while loop and outside the while loop type.
pygame.quit()
quit()
The first line uninitializes the pygame and the last quit() closes the game. So now we have a blank game that we can close. Hopefully you may have learned many useful stuff in this tutorial and stay tuned for the second one because in that tutorial we will actually build the game rather then learning about how it works.
Code
All Code Use In This Can Be Found Here
amazing @anonfiles
looking for your next contribution
Thanks for your support @funb!
Thank you for your contribution.
See this tutorial as a good example Link
Need help? Write a ticket on https://support.utopian.io/.
Chat with us on Discord.
[utopian-moderator]
Congratulations! Your post has been selected as a daily Steemit truffle! It is listed on rank 12 of all contributions awarded today. You can find the TOP DAILY TRUFFLE PICKS HERE.
I upvoted your contribution because to my mind your post is at least 34 SBD worth and should receive 104 votes. It's now up to the lovely Steemit community to make this come true.
I am
TrufflePig
, an Artificial Intelligence Bot that helps minnows and content curators using Machine Learning. If you are curious how I select content, you can find an explanation here!Have a nice day and sincerely yours,
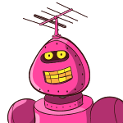
TrufflePig