Artisan Update: Change Password and Account Closure
GitHub Repository: https://github.com/chrix95/artisan
What is the project about?
Artisan tends to link clients who require the services of an artisan (example electrician, plumber, tailor e.t.c) for their various needs. We stand in the gap to provide the best of the best in the various fields to meet your expected result.
How we operate?
Artisans register on the platform to get their information displayed on the search area for clients to view and request for their services.
On the other hand, the clients
- Select the time and date that's convenient enough for him/her and let us know (via the request form provided on the landing page)
- We confirm the appointment with our very best artisan for the job
- Upon job completion, we confirm that our clients are satisfied with the job offered
New Features
- User account closure
- Users can now update their password from settings page
How I implemented this features
USER ACCOUNT CLOSURE: Artisans that are registered on the platform can actually delete their account anytime they deem it necessary. I implemented this by first designing the delete button which on click provides a form for users email to be inserted
// gets a confirmation of account closure before actually deleting the account $('#apply .delBtn').click(function(){ $('#apply').hide(); $('#delAcct').show(); }); // terminates the closure of account process on button click $('.cancel').click(function(){ $('#delAcct').hide(); $('.email').val(''); $('#apply').show(); });
Then once the email is entered, the information is sent to the php script using jquery validation. The snippet first verify that the form fields are correctly entered as required
// validates the form with this rules rules: { email: { required: true, email: true } },
After validation is done, the information is sent to a script through the submit handler and response is sent back to the JS script for necessary actions.
submitHandler: function() { //gets the form fields details var that = $('#delAcct'), url = that.attr('action'), type = that.attr('method'), data = {}; that.find('[name]').each(function(index, value){ var that = $(this), name = that.attr('name'), value = that.val(); data[name] = value; // stores the data as an array }); // sends the data to the script using ajax $.ajax({ url: '../resources/script.php', type: 'POST', data: data }) // on completion, the necessary actions are taken .done(function(data){ if (data == 'Account closure confirmed') { $('p#delmessage').css({ "color":"#9C27B0", "font-size": "15px" }); $('p#delmessage').text(data); setTimeout("window.location = 'logout.php'", 3000); } else if (data == 'Failed to close account') { $('p#delmessage').css({ "color":"#9C27B0", "font-size": "15px" }); $('p#delmessage').text(data); setTimeout("$('p#delmessage').text('')", 3000); } else if (data == 'Incorrect email provided') { $('p#delmessage').css({ "color":"#9C27B0", "font-size": "15px" }); $('p#delmessage').text(data); setTimeout("$('p#delmessage').text('')", 3000); } }) // on failure the error uis displayed in the console .fail(function(data){ console.log("error encountered"); }); }
The snippet of the script that performs the account closure is shown below. The script confirms that the email provided matches the account email and then deletes the record from the database and then logs out the user
if (isset($_POST['delAcct'])) { // get details from form $email = htmlentities(strip_tags(trim($_POST['email']))); $cmail = htmlentities(strip_tags(trim($_POST['cmail']))); // confirms if email provided matches the accounts email if ($email == $cmail) { // if the email matches // send details for account closure $delAcct = $conn->prepare("DELETE FROM registration WHERE email=:email"); $delAcct->bindParam(":email", $email); $success = $delAcct->execute(); // once the deletion process has been carried out if ($success) { echo "Account closure confirmed"; } else { echo "Failed to close account"; } } else { // if email doesn't matches echo "Incorrect email provided"; } }
PASSWORD UPDATE: The necessity may arise for a change or update of password. The artisan selects settings on his navigation section and fills the change password form and then clicks on the provided button. The button uses the jquery validation to confirm that all fields are entered correctly and the new password and confirm new password matches before sending to the PHP script.
// validates the form with this rules rules: { curpass: { required: true }, newpass: { required: true }, conpass: { required: true, equalTo: '#newpass' } }, submitHandler: function() { //gets the form fields details var that = $('#passUpdate'), url = that.attr('action'), type = that.attr('method'), data = {}; that.find('[name]').each(function(index, value){ var that = $(this), name = that.attr('name'), value = that.val(); data[name] = value; // stores the data as an array }); // sends the data to the script using ajax $.ajax({ url: '../resources/script.php', // script responsible for processing the information type: 'POST', data: data }) // on completion, the necessary actions are taken .done(function(data){ if (data == 'Password changed successfully') { $('p#message').css({ "color":"#9C27B0", "font-size": "15px" }); $('p#message').text(data); setTimeout("$('p#message').text('')", 3000); } else if (data == 'Password change failed') { $('p#message').css({ "color":"#fff", "font-size": "15px" }); $('p#message').text(data); setTimeout("$('p#message').text('')", 3000); } else if (data == 'Current password incorrect') { $('p#message').css("color", "red"); $('p#message').text(data); console.log(data); setTimeout("$('p#message').text('')", 3000); } }) // on failure the error uis displayed in the console .fail(function(data){ console.log("error encountered"); }); }
The php script gets the form details from the JS script and then verifies if the current password matches the one in the database before calling the password hash function
password_hash()
and then updating the record with the new passwordif (isset($_POST['passupdate'])) { // get details from form $email = htmlentities(strip_tags(trim($_POST['email']))); $curpassword = htmlentities(strip_tags(trim($_POST['curpass']))); $newpassword = htmlentities(strip_tags(trim($_POST['newpass']))); // confirm if the email exist $query_email = $conn->prepare("SELECT * FROM registration WHERE email=:email"); $query_email->bindParam(":email", $email); $query_email->execute(); // fetch password $get_details = $query_email->fetch(PDO::FETCH_OBJ); $retrieved_password = $get_details->password; $check_password = password_verify($curpassword, $retrieved_password); // once current password has been confirmed if ($check_password) { // hash new password and update record $hashPassord = password_hash($newpassword, PASSWORD_DEFAULT); $update_password = $conn->prepare("UPDATE registration SET password=:password WHERE email=:email"); $update_password->bindParam(":password", $hashPassord); $update_password->bindParam(":email", $email); $success = $update_password->execute(); // on success of password hashing and upadting if ($success) { echo "Password changed successfully"; } else { echo "Password change failed"; } } else { echo "Current password incorrect"; } }
Sample Video of implementation
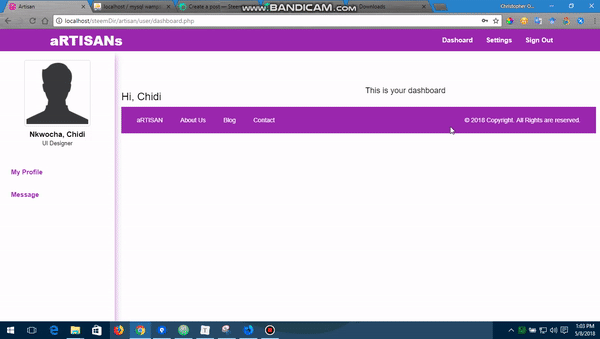
Commits on GitHub
- https://github.com/chrix95/artisan/commit/2b26c842aa6603d348d7ad532e8800f365866761
- https://github.com/chrix95/artisan/commit/a6dedd338287a7f0895c5a1b69acdb4ece9e67ff
Previous Update
- https://utopian.io/utopian-io/@chri5h/55dga9-artisans-bridging-the-gap-between-clients-and-artisans
- https://steemit.com/utopian-io/@chri5h/artisan-update-readme-contact-page-login-and-dashboard
How to contribute
- Fork the repo https://github.com/chrix95/artisan
- Create your feature branch
- Commit your changes
- Push to the branch:
- Submit a pull request.
Congratulations @chri5h! You have completed some achievement on Steemit and have been rewarded with new badge(s) :
Click on any badge to view your own Board of Honor on SteemitBoard.
To support your work, I also upvoted your post!
For more information about SteemitBoard, click here
If you no longer want to receive notifications, reply to this comment with the word
STOP
Thank you for your contribution. I feel the development effort is less on this contribution and would love to see more features in a single contribution. Also its better to write the logic rather than writing whole code in the post.
Need help? Write a ticket on https://support.utopian.io.
Chat with us on Discord.
[utopian-moderator]
Thanks for the approval. I will effect the corrections in my next contribution. But I do hope I get upvoted for this contribution.