Heisenberg 0.1.1: Rest API wrapper and update on "attack" command
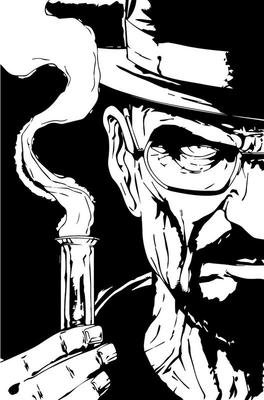
Heisenberg is a Python command line application and a library to play drugwars without using the website.
Drugwars is a blockchain based game. That makes it possible for the blockchain accounts to play the game using off-site transactions. Heisenberg is a set of tools to make that easier in Python environments.
Before going into the details of new features, make sure you updated the library in your environment. All changes are backward compatible.
$ (sudo) pip install heisenberg_drugwars --upgrade
Rest Client
This is a new REST API from the drugwars team which serves handful endpoints about the game.
Get the RestClient
instance:
from heisenberg.rest_client import RestClient
rest_client = RestClient()
Globals
globals = rest_client.dynamic_global_properties())
print(globals)
Example output:
{
'daily_percent': 3,
'heist_percent': 3,
'last_prod_update': '2019-03-04T13:37:09.151Z',
'drug_production_rate': 3675.5369999999757,
'heist_pool': 86728017,
'balance': '66467.641 STEEM',
'steemprice': 0.3736408799
}
Fights
Return the recent fights of a player.
fights = rest_client.fights('<player_name>'))
print(fights)
Users
List players by production rate.
users = rest_client.users(max_production_rate=420))
print(users)
User data
Return the game data of a player/
player_data = rest_client.info('<player_bame>'))
print(player_data)
calculate_balance
helper
Game doesn't update the balances on resources every second.
Example account data:
'drugs_balance': 420,
'drug_production_rate': 4.200,
'drug_storage': 420000,
'last_update': '2019-03-04T13:37:03.000Z',
That information means that the drug balance was 420 at the 2019-03-04T13:37:03.000Z
. It's client's responsibility to calculate regeneration from that balance to calculate the actual, uptodate balance.
heisenberg.utils
module has a helper to make that calculation. For example, this snippet will print the actual balance:
rest_client = RestClient()
account_data = rest_client.info('<player_name>')
print(calculate_balance('drug', account_data))
Auto Invest to Heist script in the repository is also updated to use the available drug balance instead of a fixed -estimated- balance.
Related pull requests:
Updating Attack functionality
The first version of the battle system was designed like this:
With the recent changes on the game, it has changed into a new data structure:
Related changes are implemented to Heisenberg with the new version.
As a side note,the custom_json ID for the battles changed from dw-attack
to drugwars
. I think the development team plans to get all drugwars related actions into drugwars
namespace.
Related Pull Request:
https://github.com/emre/heisenberg/pull/1
Disclaimer
Drugwars is on constant development progress. Custom JSON definitions on the game may change. I am planning to maintain and update the library as long as I play the game, however, make sure you always audit the code and use it on your own risk.
I've been playing this game on and off, but couldn't really see the point and was quickly getting bored of it. Hopefully with them reintroducing the battles it might become a bit more interesting, but I will have to wait and see. Imo if they add something that you can make your own mafia with others then I would definitely get on board.
As for the code:
drugwars_heisenberg
-heisenberg_drugwars
does exist, but it's only version 0.1.0, and with this post it's meant to be 0.1.1.shell.py
do_exit
can be a function sinceself
is never used, and in the filetest.py
the variableheist
is never used.0.99
and0.005
which could be made into constants.Great work making sure it stays up to date, and I am sure you are up to the challenge of continuing to do this! I've seen a lot of posts from people complaining about the fact that so much is changing constantly, which must be a headache for you, haha.
Your contribution has been evaluated according to Utopian policies and guidelines, as well as a predefined set of questions pertaining to the category.
To view those questions and the relevant answers related to your post, click here.
Need help? Chat with us on Discord.
[utopian-moderator]
Thanks for the perfect review @amosbastian. Package naming issues are fixed. Will consider the other changes in the next iteration. 👍
Thank you for your review, @amosbastian! Keep up the good work!
Damn you just stole 20k/20k/20k from my alt @smartasscards due to the lag. How dare you pay attention to my troop movements :D
Regulators
always watch :DGreat work! Thank you so much!
Sadly the drugwars.io REST-API seems to be gone at the moment.
Getting {"error":"This page doesn't exist"} for about an hour now.
Hope it's only a temporary issue.
yeah, it seems there are some problems on the traffic they get into their API. It's been worked on, I am sure it will be up, soon. :)
Interesting stuff !
On a different note, The "proposals" on the Bitshares chain could have been a great addition to Steem to pull of games like Drugwars. ... ie http://docs.bitshares.org/bitshares/user/transactions-proposed.html
Hi @emrebeyler!
Your post was upvoted by @steem-ua, new Steem dApp, using UserAuthority for algorithmic post curation!
Your post is eligible for our upvote, thanks to our collaboration with @utopian-io!
Feel free to join our @steem-ua Discord server
I have problem to run included example. h.heist(1000) works but client=RestClient() is not working with error ModuleNotFoundError: No module named 'heisenberg.rest_client'
I have it in my imports - just like in example
RestClient is shipped with the new version. Can you try upgrading w/
should return 1.1.0 if you have successfully upgraded.
I have 0.1 version. This might be the problem. Conda don't see heisenberg at all, pycharm has 0.1.0 version and when I try to install using pip then I get plenty Visual Studio errors and fail.
So I copied source to my folder then now I have ModuleNotFoundError: No module named 'dateutil'
And when I want to install it I can't since it is colliding with steem ... I need to take a break from this.
I gave it another try with ubuntu this time, and after installing using pip I still have 0.1 version. Guess I'm total noob.
It's not your fault, the latest version on PyPI is 0.1.0, we just have to wait for @emrebeyler to update it. Of course, you can also clone his repository and install it locally!
lol, I wasn't aware of that. pypi is updated, you can try again @steelman! :-)
I am getting an error:
Returns:
It works fine when I just ask it to deposit to heist so I am a bit confused here. Other than that, great job :)
This post has been included in the latest edition of SoS Daily News - a digest of all the latest news on the Steem blockchain.
Amazing idea!
Hey, @emrebeyler!
Thanks for contributing on Utopian.
We’re already looking forward to your next contribution!
Get higher incentives and support Utopian.io!
Simply set @utopian.pay as a 5% (or higher) payout beneficiary on your contribution post (via SteemPlus or Steeditor).
Want to chat? Join us on Discord https://discord.gg/h52nFrV.
Vote for Utopian Witness!