Implementing Syde An online Forum in Flutter (Update : Authenticate With Google)
Repository
https://github.com/enyason/Syde
History
New Features
Authenticate User
A platform such as this should be able to identify it's users. To achieve this, I implemented Google sign in.
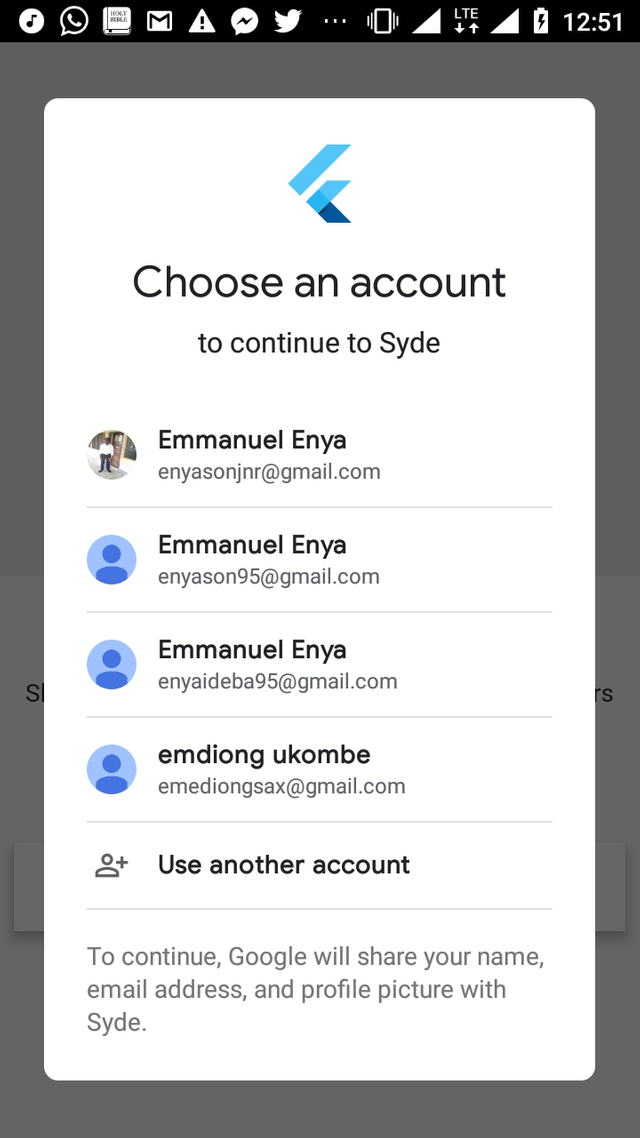
Implementation
Implementing the authentication was a bit straight forward with the flutter plug-in. To make things modular I used the bloc pattern to handle click events from the user and then respond to the click event by calling the sign in method on an abstract class I created. This abstract class handles everything concerning authentication.
- The code block below is the auth bloc where we listen for data on the stream and react accordingly
class AuthBloc {
final BaseAuth baseAuth;
//stream controller to handle streams
StreamController<ClickEvents> _controller = StreamController<ClickEvents>();
AuthBloc(this.baseAuth) {
//listen for data on the stream
_inputStream().listen((event) {
if (event is ClickEvents) {
baseAuth.signInWithCredentials(baseAuth.getFireBAseAuth());
}
});
}
//create a stream
Stream<ClickEvents> _inputStream() {
return _controller.stream;
}
//create a sink- with a sink, data can be added unto the stream
Sink<ClickEvents> get eventSink => _controller.sink;
//close the stream
void dispose() {
_controller.close();
}
}
abstract class ClickEvents {}
class LogInClick extends ClickEvents {}
//make a global instance of AuthBloc
final authBloc = AuthBloc(Auth());
- The code snippet below shows abstract methods used for implementing the authentication. for full details check source code
abstract class BaseAuth {
FirebaseAuth getFireBAseAuth();
GoogleSignIn getGoogleLogin();
Future<FacebookLoginResult> initFaceBookLogIn(
FacebookLogin fbLogIn, List<String> permission);
Future<GoogleSignInAuthentication> getGoogleSignInAuth();
AuthCredential getAuthCredential(GoogleSignInAuthentication auth);
Future<void> logUserOut(FirebaseAuth mAuth);
Future<void> storeUser(FirebaseUser user);
Future<FirebaseUser> signInWithCredentials(FirebaseAuth mAuth);
}
- The code block stores user's details to fire-store if user details do not exist
Future<void> storeUser(FirebaseUser user) async {
if (user != null) {
// Check is already sign up
final QuerySnapshot result = await Firestore.instance
.collection('users')
.where('id', isEqualTo: user.uid)
.getDocuments();
final List<DocumentSnapshot> documents = result.documents;
documents.forEach((val) {
print("Data = ${val.data}");
});
if (documents.length == 0) {
// Update data to server if new user
Firestore.instance.collection('users').document(user.uid).setData({
'name': user.displayName,
'photoUrl': user.photoUrl,
'id': user.uid,
'created': DateTime.now().millisecondsSinceEpoch.toString()
});
}
}
}
- From the Login Screen, all we need is a line of code in the
onTap
function to begin authenticating a user
onTap: () {
authBloc.eventSink.add(LogInClick());
}
Technology Stack
- Android Studio
- Flutter: Flutter is google mobile framework for building beautiful cross-platform apps
- FireBase: Firebase will provide us with online backend functionality such database, cloud functions, authentication, real-time functionalities etc
- SQFlite : this is flutter version of SQLite. This will enable local storage on the phone
- RxDart : RxDart is used for reactive programming
- BLOc pattern is used as the architectural pattern for layering the app.
IMPORTANT RESOURCES
*Github *: https://github.com/enyason/Syde
Roadmap
The screenshots above show the current state of the application. I will work towards implementing all the features as described above. More attention would be given to the layouts and presentation of the app, to improve the user's experience. Some of the updates to expect are:
Authenticate User- Persisting user post
- Searching all posts
- Providing different layouts
- ToDo for users to track their daily progress
- Push Notifications
- Posts sharing
- Users dashboard
- Searching all users
- Direct Messaging with Users
- ChatGroup
- Bookmarking post
Demo Video
Github - https://github.com/enyason/Syde
How to contribute?
You can reach me by commenting on this post or send a message via [email protected] you want to make this application better, you can make a Pull Request. Github
Your contribution has been evaluated according to Utopian policies and guidelines, as well as a predefined set of questions pertaining to the category.
To view those questions and the relevant answers related to your post, click here.
Need help? Chat with us on Discord.
[utopian-moderator]
Thank you for your review, @helo! Keep up the good work!
Hi, @ideba!
You just got a 0.67% upvote from SteemPlus!
To get higher upvotes, earn more SteemPlus Points (SPP). On your Steemit wallet, check your SPP balance and click on "How to earn SPP?" to find out all the ways to earn.
If you're not using SteemPlus yet, please check our last posts in here to see the many ways in which SteemPlus can improve your Steem experience on Steemit and Busy.
Hi @ideba!
Your post was upvoted by @steem-ua, new Steem dApp, using UserAuthority for algorithmic post curation!
Your post is eligible for our upvote, thanks to our collaboration with @utopian-io!
Feel free to join our @steem-ua Discord server
Hey, @ideba!
Thanks for contributing on Utopian.
We’re already looking forward to your next contribution!
Get higher incentives and support Utopian.io!
Simply set @utopian.pay as a 5% (or higher) payout beneficiary on your contribution post (via SteemPlus or Steeditor).
Want to chat? Join us on Discord https://discord.gg/h52nFrV.
Vote for Utopian Witness!