[PHP | MySQL ] User Registration & User login System | Lotto web application building tutorial part-3
Repository
https://github.com/kibriakk/lotto
What Will You Learn?
After complete the full tutorial you will learn
- How to create a simple lotto web application
- How to create online payment methood
- How to create user and admin system in a site
- How to secure a site
- How to build a respinsive backend template
What Will You Learn from This part
From this part of my tutorial you will learn -
- How to create a login page
- How to create a registration page
- How to connect login & registration page with database
Requirements
- Php server
- MySQL database
- Knowledge about PHP, HTML, CSS and MySQL
Difficulty
- Intermediate
Tutorial Contents
Hello steemians,
This is my third part of the tutorial. Today I will teach everyone how we can create a login, registration & forget password page. It is nessesary for every website / applications.
This part is so large. So we work partically. The theory is not nessesary for this part. I will describe shortly every function. I am really sorry that my English speech is not so good.
So lets start the tutorial-
Previous part I write the tutorial - how to create database for user managememt.
- At first go to the 'root' folder of your web application.
- create 2 new file and named them 'login.php', 'registration.php' ,
- Open the 'login.php' with any text editor. Then write the following code
require_once('function.php');
connectdb();
session_start(); // for database connection
if (is_user()) {
redirect("$baseurl/dashboard");
}
?>
Here first three line is for connecting database. last two line is for redirect logged user to dashboard. So, the logged user can not access login page.
- Then write this code
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0, maximum-scale=1.0">
<meta name="description" content="">
<meta name="author" content="">
<link rel="shortcut icon" href="<?php echo $baseurl; ?>/images/fav.png" type="image/png"> // (site icon) Change it to your favicon url
<title>Sign In</title>
<link href="<?php echo $baseurl; ?>/css/style.default.css" rel="stylesheet"> // (css stylesheet) change it to your stylesheet
</head>
This is the head tag of your login page
- Then write these html code in
<body></body>
tag
<form method="post" action="">
<h4 class="nomargin">Sign In</h4>
<p class="mt5 mb20">Don't have an Account? <a href="<?php echo $baseurl; ?>/signup"><strong>Sign Up</strong></a></p>
<input type="text" class="form-control uname" name="username" placeholder="Username" />
<input type="password" class="form-control pword" name="password" placeholder="Password" />
<button class="btn btn-success btn-block">Sign In</button>
</form>
now visit this page with any browser you can see that there is a login form.
But the form will not working. So now we write php code after the <form>
tag.
<?php
if ($_POST){
$username = $_POST['username'];
$password = md5($_POST['password']);
$count = mysql_fetch_array(mysql_unbuffered_query("SELECT COUNT(*) FROM users WHERE username='".$username."' AND password='".$password."'"));
if ($count[0]==1) {
$uname = $_POST['username'];
$time = time();
$si = "$uname$time";
$sid = md5($si);
$_SESSION['sid'] = $sid;
$_SESSION['username'] = $username;
mysql_query("UPDATE users SET sid='".$sid."' WHERE username='".$uname."'");
redirect("$baseurl/dashboard");
}else{
echo '
<div class="alert alert-danger alert-dismissable">
<button type="button" class="close" data-dismiss="alert" aria-hidden="true">×</button>
Wrong username or password
</div>';
}
}
?>
<?php if (!empty($_GET['error'])): ?>
<?php endif ?>
I’ve quoted every code with commenting that which line doing what & which function is using for what. As we can see first of all we’ve included the db connection so that we can have the connection while calling the db connection through a object in constructor.
So the full code of login page is
<?php
require_once('function.php');
connectdb();
session_start();
if (is_user()) {
redirect("$baseurl/dashboard");
}
?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0, maximum-scale=1.0">
<meta name="description" content="">
<meta name="author" content="">
<link rel="shortcut icon" href="<?php echo $baseurl; ?>/images/fav.png" type="image/png">
<title>Sign In</title>
<link href="<?php echo $baseurl; ?>/css/style.default.css" rel="stylesheet">
</head>
<form method="post" action="">
<h4 class="nomargin">Sign In</h4>
<p class="mt5 mb20">Don't have an Account? <a href="<?php echo $baseurl; ?>/signup"><strong>Sign Up</strong></a></p>
<?php
if ($_POST){
$username = $_POST['username'];
$password = md5($_POST['password']);
$count = mysql_fetch_array(mysql_unbuffered_query("SELECT COUNT(*) FROM users WHERE username='".$username."' AND password='".$password."'"));
if ($count[0]==1) {
$uu = $_POST['username'];
$tm = time();
$si = "$uu$tm";
$sid = md5($si);
$_SESSION['sid'] = $sid;
$_SESSION['username'] = $username;
mysql_query("UPDATE users SET sid='".$sid."' WHERE username='".$uu."'");
redirect("$baseurl/dashboard");
}else{
echo '
<div class="alert alert-danger alert-dismissable">
<button type="button" class="close" data-dismiss="alert" aria-hidden="true">×</button>
Wrong username or password
</div>';
}
}
?>
<?php if (!empty($_GET['error'])): ?>
<?php endif ?>
<input type="text" class="form-control uname" name="username" placeholder="Username" /> // username field
<input type="password" class="form-control pword" name="password" placeholder="Password" /> //password field
<button class="btn btn-success btn-block">Sign In</button> //sign in button
</form>
</body>
You should add css, stylesheet and design the page.
I will add full design with script after finishing full tutorial.
The registrations and forget password page is same of this. so I just write only the code.
For register.php file
<?php
require_once('function.php');
connectdb();
session_start();
if (is_user()) {
redirect("$baseurl/dashboard");
}
?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0, maximum-scale=1.0">
<meta name="description" content="">
<meta name="author" content="">
<link rel="shortcut icon" href="<?php echo $baseurl; ?>/images/fav.png" type="image/png">
<title>Sign Up</title>
<link href="<?php echo $baseurl; ?>/css/style.default.css" rel="stylesheet">
</head>
<body class="signin">
<form method="post" action="">
<h3 class="nomargin">Sign Up</h3>
<p class="mt5 mb20">Already have an Account? <a href="<?php echo $baseurl; ?>/signin"><strong>Sign In</strong></a></p>
<?php
if(isset($_GET['ref'])) {
$ref = $_GET['ref'];
$rrr = mysql_fetch_array(mysql_query("SELECT id FROM users WHERE username='".$ref."'"));
}else{
$rrr[0] = 0;
}
//echo "$rrr[0] ----------------- ";
if($_POST)
{
$username = $_POST["username"];
$pass1 = $_POST["password1"];
$pass2 = $_POST["password2"];
$email = $_POST["email"];
$country = $_POST["country"];
$phone = $_POST["phone"];
$err1=0;
$err2=0;
$err3=0;
$err4=0;
$err5=0;
$err6=0;
$err7=0;
$err8=0;
if(trim($username)=="")
{
$err1=1;
}
if(trim($email)=="")
{
$err2=1;
}
if($pass1!=$pass2)
{
$err3=1;
}
if(strlen($pass1)<="3")
{
$err4=1;
}
$nnn = mysql_fetch_array(mysql_query("SELECT COUNT(*) FROM users WHERE username='".$username."'"));
if($nnn[0]>="1")
{
$err5=1;
}
$eee = mysql_fetch_array(mysql_query("SELECT COUNT(*) FROM users WHERE email='".$email."'"));
if($eee[0]>="1")
{
$err6=1;
}
$ppp = mysql_fetch_array(mysql_query("SELECT COUNT(*) FROM users WHERE phone='".$phone."'"));
if($ppp[0]>="1")
{
$err7=1;
}
$error = $err1+$err2+$err3+$err4+$err5+$err6+$err7;
if ($error == 0){
$passmd = md5($pass1);
if($rrr[0]=="0" || $rrr[0]==""){
$r = 1;
}else{
$r = $rrr[0];
}
$res = mysql_query("INSERT INTO users SET username='".$username."', email='".$email."', password='".$passmd."', phone='".$phone."', country='".$country."', ref='".$r."'");
if($res){
echo "<div class=\"alert alert-success alert-dismissable\">
<button type=\"button\" class=\"close\" data-dismiss=\"alert\" aria-hidden=\"true\">×</button>
Register Completed Successfully!
</div>";
///////////////////------------------------------------->>>>>>>>>Send Email
$to = "$email";
$subject = 'Welcome to lotto';
$message = 'Hi,'."\r\n Thanks For us.";
$headers = 'From: ' . "$sender \r\n" .
'Reply-To: ' . "$sender \r\n" .
'X-Mailer: PHP/' . phpversion();
mail($to, $subject, $message, $headers);
///////////////////------------------------------------->>>>>>>>>Send Email
///////////////////------------------------------------->>>>>>>>>Send SMS
/*
$msg = "Thanks For Joining O";
$sendsms = "http://bulksms.hostrecline.com/gateway/?user=*************************&pass=**********&to=$phone&sender=lotto&message=$msg";
$getsmsstatus = file_get_contents($sendsms);
*/
///////////////////------------------------------------->>>>>>>>>Send SMS
}else{
echo "<div class=\"alert alert-danger alert-dismissable\">
<button type=\"button\" class=\"close\" data-dismiss=\"alert\" aria-hidden=\"true\">×</button>
Some Problem Occurs, Please Try Again.
</div>";
}
} else {
if ($err1 == 1){
echo "<div class=\"alert alert-danger alert-dismissable\">
<button type=\"button\" class=\"close\" data-dismiss=\"alert\" aria-hidden=\"true\">×</button>
Username Can Not be Empty!!!
</div>";
}
if ($err2 == 1){
echo "<div class=\"alert alert-danger alert-dismissable\">
<button type=\"button\" class=\"close\" data-dismiss=\"alert\" aria-hidden=\"true\">×</button>
Email Can Not be Empty!!!
</div>";
}
if ($err3 == 1){
echo "<div class=\"alert alert-danger alert-dismissable\">
<button type=\"button\" class=\"close\" data-dismiss=\"alert\" aria-hidden=\"true\">×</button>
Passwords Don't match!!
</div>";
}
if ($err4 == 1){
echo "<div class=\"alert alert-danger alert-dismissable\">
<button type=\"button\" class=\"close\" data-dismiss=\"alert\" aria-hidden=\"true\">×</button>
Password Can not be less than 4 Letter
</div>";
}
if ($err5 == 1){
echo "<div class=\"alert alert-danger alert-dismissable\">
<button type=\"button\" class=\"close\" data-dismiss=\"alert\" aria-hidden=\"true\">×</button>
Username Already Exist !
</div>";
}
if ($err6 == 1){
echo "<div class=\"alert alert-danger alert-dismissable\">
<button type=\"button\" class=\"close\" data-dismiss=\"alert\" aria-hidden=\"true\">×</button>
Email Already Exist !
</div>";
}
if ($err7 == 1){
echo "<div class=\"alert alert-danger alert-dismissable\">
<button type=\"button\" class=\"close\" data-dismiss=\"alert\" aria-hidden=\"true\">×</button>
Phone Number Already Exist !
</div>";
}
}
}
?>
<input type="text" class="form-control uname" name="username" placeholder="Username" /> //username field
<input type="text" class="form-control email" name="email" placeholder="Email" /> // email field
<input type="text" class="form-control phn" name="phone" placeholder="Mobile eg: 8801XXXXXXXXX" /> //phone number field
<select class="form-control input-lg" name="country">
<option Value="Bangladesh">Bangladesh</option>
<option Value="India">India</option>
<option Value="Pakistan">Pakistan</option>
<option Value="USA">USA</option>
<option Value="Canada">Canada</option>
<option Value="UK">UK</option>
</select> // country option. you can add more country.
<input type="password" class="form-control pword" name="password1" placeholder="Password" /> //password field
<input type="password" class="form-control pword" name="password2" placeholder="Retype Password" /> // confirm pass field
<button class="btn btn-success btn-block">Sign Up</button> //signup button
</form> // form end tag
</body>
</html>
All codes are too easy to realize. No need to change anything. You can change variables for security. If you have any question, please put a comment here. Note that here sid and password will store with md5 encryption.
Curriculum
- Building a Online Lotto Web Application. Tutorial -1 : basic function of the lotto web application.(connecting mysql in website by function.php)
- Building a Online Lotto Web Application. Tutorial -2 : Creating User data storing and management database by SQL.
Hi @kibria365!
Your post has been upvoted by @bdcommunity.
You can support us by following our curation trail or by delegating SP to us.
20 SP, 50 SP, 100 SP, 300 SP, 500 SP, 1000 SP.
If you are not actively voting for Steem Witnesses, please set us as your voting proxy.
Feel free to join BDCommunity Discord Server.
Hello! Your post has been resteemed and upvoted by @ilovecoding because we love coding! Keep up good work! Consider upvoting this comment to support the @ilovecoding and increase your future rewards! ^_^ Steem On!
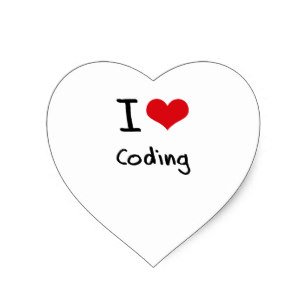
Reply !stop to disable the comment. Thanks!
Hi @kibria365!
Your post was upvoted by @steem-ua, new Steem dApp, using UserAuthority for algorithmic post curation!
Your post is eligible for our upvote, thanks to our collaboration with @utopian-io!
Feel free to join our @steem-ua Discord server
Congratulations @kibria365! You have completed the following achievement on the Steem blockchain and have been rewarded with new badge(s) :
Click on the badge to view your Board of Honor.
If you no longer want to receive notifications, reply to this comment with the word
STOP
Do not miss the last post from @steemitboard:
Congratulations @kibria365! You have completed the following achievement on the Steem blockchain and have been rewarded with new badge(s) :
Click on the badge to view your Board of Honor.
If you no longer want to receive notifications, reply to this comment with the word
STOP
Do not miss the last post from @steemitboard:
Hey, @kibria365!
Thanks for contributing on Utopian.
We’re already looking forward to your next contribution!
Get higher incentives and support Utopian.io!
Simply set @utopian.pay as a 5% (or higher) payout beneficiary on your contribution post (via SteemPlus or Steeditor).
Want to chat? Join us on Discord https://discord.gg/h52nFrV.
Vote for Utopian Witness!