Bot Build #10 - Simple Automatic Curation Bot
Repository
e.g. https://github.com/steemit/steem-js
What Will I Learn?
In brief, write details of what the user is going to learn in a bullet list.
- You will learn how to create simple automatic curation bot
Requirements
- Node.JS, Here
- Steem Package (Install: npm install steem --save)
Difficulty
- Intermediate
Tutorial Contents
You will learn how to create simple automatic curation bot with SteemJS.
Curriculum
- Bot Build #1
- Bot Build #2
- Bot Build #3
- Bot Build #4
- Bot Build #5
- Bot Build #6
- Bot Build #7
- Bot Build #8
- Bot Build #9
- SteemJS Tutorials #1
- NodeJS Tutorials #1
The Tutorial
Step 1 - Setup Variables & Functions
we need 7 variables, account name & key(WIF), VOTE WEIGHT, TIMER, Next Round Timer (When The Next Round Starts In Hours), TAG (Category), LIMIT (Limit Of Loaded Posts).
Note
: if you want to change the limit know that 80% of the times only one-two votes will stream to the blockchain.
const TAG = 'photography',
LIMIT = 1; // if you want to change the limit know that 80% of the times only one-two votes will stream to the blockchain.
const ACC_NAME = 'guest123',
ACC_KEY = '5JRaypasxMx1L97ZUX7YuC5Psb5EAbF821kkAGtBj7xCJFQcbLg',
WEIGHT = 20*1000, //20% - 20*5 = 100, 100% per round.
TIMER = 12, // NUMBER = MINUTES. [Normally = 12m]
NEXTROUND = 0.5; //0.5 = Half Hour, NUMBER = HOUR.
Account Name & WIF Key = SteemJS Public User, everybody can use it.
now we need two functions, one for the vote and one for the bot.
first because at my previous tutorials I already used the streamVote function I'll just paste it and I'm not going to explain it.
function streamVote(author, permalink, weight) {
steem.broadcast.vote(ACC_KEY, ACC_NAME, author, permalink, weight, function(err, result) {
if(err)
throw err;
console.log('Voted Succesfully, permalink: ' + permalink + ', author: ' + author + ', weight: ' + weight / 1000 + '%.');
});
}
and we need a function called StartCuration
function StartCuration(){
}
Step 2 - Create The Bot
- everything in this section works inside the function!
now we need to get the last posts that created at the blockchain with our TAG and LIMIT.
//Getting Latest Discussions Created by tag (1 post, current limit)
steem.api.getDiscussionsByCreated({tag: TAG, limit: LIMIT}, function(err, result){
console.log(LIMIT + " Post(s) Loaded, Waiting "+TIMER+" minutes to vote.");
}
this function gets the latest discussions
, posts that created in the steem blockchain.
and at the start, we send a comment to the console so we can know that the curation bot start.
Note
: if you want to use Limit higher than 1 use a loop
result.forEach(function(res){
console.log(res);
});
and use res
instead of result[0]
.
now we need a timer with our TIMER variable (minutes) and when it ends it will send the vote.
setTimeout(function(){streamVote(result[0].author, result[0].permlink, WEIGHT);}, TIMER*60*1000);
if(TIMER < 1){
console.log(TIMER*60+"s Timer Start For The Post(Permalink): " + result[0].permlink);
}else{
console.log(TIMER+"m Timer Start For The Post(Permalink): " + result[0].permlink);
}
so first we use setTimeout
function, this function creates a timer and uses function and milliseconds(time).
at the function, we use the streamVote function with the author name, permalink and the voting weight.
at the time, 1000 milliseconds = 1 second
60 seconds = 60k ms
and TIMER = Minutes, 12 Minutes is really good time to get full curation, if you want to be sure change the timer to 20 minutes.
after the timer, we check if the TIMER variable is seconds or minutes, higher than one is minutes, less than one is seconds.
so for example 0.5 = 30 seconds.
and then we send a comment to the console so we can know that the timer started successfully.
now we just need to know when we start the next round.
if(NEXTROUND < 1){
setTimeout(function(){console.log("Next Round In "+NEXTROUND*60+" Minutes...");}, (TIMER*60*1000) + 5*1000);
setTimeout(function(){StartCuration();}, NEXTROUND*60*60*1000);
}else{
setTimeout(function(){console.log("Next Round In "+NEXTROUND+" Hours...");}, (TIMER*60*1000) + 5*1000);
setTimeout(function(){StartCuration();}, NEXTROUND*60*60*1000);
}
we check if the next round timer is higher than a full hour, if it is we start a timer that equals to the time of the TIMER variable (when the vote streaming to the blockchain) and we take more 5 seconds and send comment to the bot and start new timer and at the function start the curation bot again.
and here we done!
results:
1 Post(s) Loaded, Waiting 0.1 minutes to vote.
6s Timer Start For The Post(Permalink): the-meaning-of-a-sunset-in-life-6226c0d21700d
Voted Succesfully, permalink: the-meaning-of-a-sunset-in-life-6226c0d21700d, author: nurulhusna1, weight: 2%.
Next Round In 30 Minutes...
and here we're done, I used 6 seconds timer to not wait too long.
Please! for the next tutorial I will be really happy if you can give me a suggestion for the next script/tutorial!
have a great day!
You can check the full work at my repl.it account and other bots that I create!
Proof of Work Done
the work made in Repl.it, https://repl.it/@lonelywolf22/Steem-Bots-Simple-Curation-Bot-V1-Done
user: https://repl.it/@lonelywolf22
GitHub: https://github.com/lonelywolf1
Utopian Tutorials GitHub Repository: https://github.com/lonelywolf1/Bot-Projects-SteemJS (This tutorial will add to the GitHub repository in the next few days!)
Thank you for your contribution.
While I liked the content of your contribution, I would still like to extend one advice for your upcoming contributions:
Looking forward to your upcoming tutorials.
Your contribution has been evaluated according to Utopian policies and guidelines, as well as a predefined set of questions pertaining to the category.
To view those questions and the relevant answers related to your post, click here.
Need help? Write a ticket on https://support.utopian.io/.
Chat with us on Discord.
[utopian-moderator]
Powerful
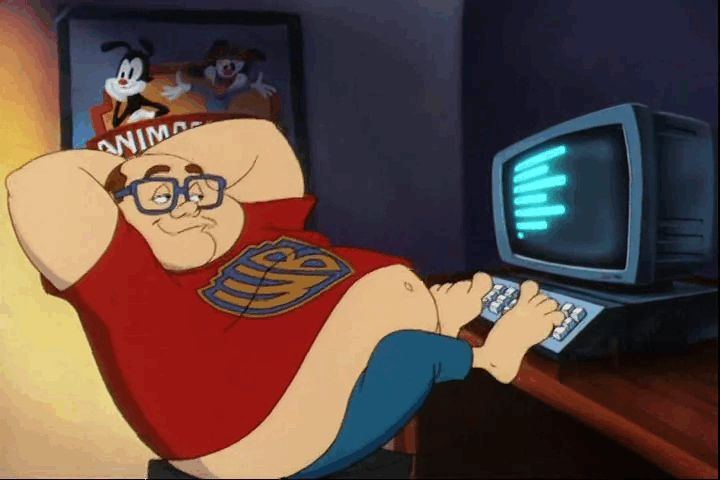
Hey @lonelywolf
Thanks for contributing on Utopian.
We’re already looking forward to your next contribution!
Contributing on Utopian
Learn how to contribute on our website or by watching this tutorial on Youtube.
Want to chat? Join us on Discord https://discord.gg/h52nFrV.
Vote for Utopian Witness!
Hi @lonelywolf ,
First of all I would like to thank you for all the good work you are doing for the community.
Now, Let me explain what I am looking for:
I am the owner of a community account called @runningproject . It is an initiative to promote running posts in steemit.
We provide upvotes to the members as well as an automatic resteem of their Running posts, leaving a comment every time we resteem.
Now we are doing this "work" manually but we would like to implement an automatic system in order to both, resteem and leave a comment on those posts of the members using a particular tag (#running).
I read an old post from you https://steemit.com/utopian-io/@lonelywolf/bot-build-4-resteem-bot-with-friend-list
on which you explain the first function.
My question is: Would you like to contribute with us in order to provide us a "Resteem bot with friends + leave comment" solution?
Thanks in advance for your help.
If you want to know more about @runningproject please, have a look to the following post:
https://steemit.com/runningproject/@runningproject/the-steem-running-project-9th-status-report-runningproject-has-reached-300-sp
Hi Bro! you can check my new tutorial, https://steemit.com/utopian-io/@lonelywolf/bot-build-11-advanced-resteem-by-friend-list-bot
thanks for your suggestion!
This is A Test Text!
What happen when block contains no transactions?