A Beginners Guide to Python Programming - Part 1: If statements, Loops and Functions
Dear future Python programmers, welcome to the first real part of our Beginners guide to Python Programming!
Repository
https://github.com/python/cpython
What Will I Learn?
In this first part we will learn:
- Different python object types and some important operations tied to them,
- The structure and use of if statements,
- Structure of two main loops - while and for loop,
- Structure and uses of functions
Requirements
The user must have installed at least Python 2.7 or later.
Difficulty
Basic
Curriculum
Why python?
First lets start with the pure basics. What is Python? It is a high-level programming language which is strongly object oriented that is very good at general programming stuff. This means it is very intuitive to a non-programmer but it does mean that is quite slow compared to other programming languages like C and Fortran. The mentioned languages are much quicker in their computations meaning they are usually used in Mathematical and Physics settings, but because they lack objects they are bit more cumbersome when trying to do some simpler programming, which we will do. On the other hand. On the other hand there exist languages like Ruby or Javascript which rely heavily on objects, but focus more on web applications.
So why Python? Python is in the perfect middle ground where you have a very basic understanding of programming which allows you to more easily adopt web-developing languages through your skill at using objects or you can go into more low level but computational programming through the knowledge of some of the scientific libraries in Python like NumPy and SciPy.
This is not even near of a complete lists of pros and cons for the mentioned programming languages as I derive most of these facts from my personal experience.
Basic python object types
So let us start! Everything in Python is considered an object belonging to a certain object type called a class. There are at least 10 different in built classes in Python. These classes have so-called attributes that are associated with them and also some methods that can be used on them. Attributes are properties of the class like their the length of a word (string in python) or a list. We can check a specific attribute belonging to a object through the following action:
object.attribute
As an example we can input a complex number into python and check its imaginary and real part by checking its attributes:
In(1): z = 1 + 2j
In(2): z.imag
Out(2): 2.0
In(3): z.real
Out(3): 1.0
Classes also have certain methods that work on them. The methods are basically functions designed specifically for a certain class. In general methods are called like:
object.method(args*)
Where args is an arbitrary amount of parameters you can input into the method. Lets again take an example from the class of complex numbers:
In(1): z = 1 + 2j
In(2): z.conjugate()
Out(2): 1-2j
Another interesting example can be taken from the object class of lists or strings. In python they are considered very similarly as one can be converted into the other quite simply. Lets look at at some methods for lists. Let us first attempt to join two lists together, so one goes to the end of the other. This can be done in several ways:
In(1): list1 = [1, "a", 2, 3.377377, "asdsa"]
In(2): list2 = [15, "fsaf", None, 3.377377, "a"]
In(3): list1.append(list2)
In(4): list1
Out(4): [1, "a", 2, 3.377377, "asdsa",15, "fsaf", None, 3.377377, "a"]
So why did nothing happen when we input list1.append(list2). This is because this is a method called on list1 that appends list2 to list1, but in this processes it changes what list1 is. It is no longer the original input. If we want to conserve the identity we can use a syntax workaround:
In(1): list1 = [1, "a", 2, 3.377377, "asdsa"]
In(2): list2 = [15, "fsaf", None, 3.377377, "a"]
In(3): list 3 = list1 + list2
In(4): list3
Out(4): [1, "a", 2, 3.377377, "asdsa",15, "fsaf", None, 3.377377, "a"]
In this way we now have 3 different lists! In summary one has to be careful with methods as they change the object that they were called on! One important thing that must still be said is how you can check to which class does the object you are working with belong. It can be done using the function type(object). Example:
In(1): type(1)
Out(1): int
In(2): type([15, "fsaf", None, 3.377377, "a"])
Out(2): list
In(3): type(3.123131)
Out(3): float
In(4): type("3.213132")
Out(4): str
There is a whole multitude of different in-built python classes which you can find in their documentation. One can also build their own classes with their own attributes and methods. We will cover that in a later lesson.
If statement
If statements have a simple syntax form:
if (cond1):
expr1
elif(cond2):
expr2
.
.
.
else:
exprN
The logic behind it is simple. If cond1 returns the value of True then expr1 is executed, if cond1 is False then we go to check cond2 and so on. If no conditions return True, then the else exprN is executed. If no conditions return True and you want nothing to happen you can set exprN = None or you can simply leave out the else entirely. The indentations are important in Python as Python has a nested structure. Everything that happens if cond1 return True must have the same indentation. Of course it is possible that the code in expr1 requires its own indentations. Lets look at a working example of an If statement that you can use in your homework:
print("Please choose your favourite color!")
favColor = input("You can choose between: Red, Blue, Green or Yellow!")
if (favColor == "Red"):
print("My favorite color is " + favColor + " too!")
elif (favColor == "Blue"):
print("My favorite color is " + favColor + " too!")
elif (favColor == "Greeny"):
print("My favorite color is " + favColor + " too!")
else:
print("My favorite color is " + favColor + " too!")
It is a simple example but it shows the entire capability of the if statement! We have also shown how one can print things to the console or how one can read input from the console. You can see that string objects like the one we want to print into the console also have the same "+" syntax to .append() as did lists.
You can find various logical operators in python listed here
For and while loop
Both loops have very similar structures and uses. I would say that 99 % of the time the for loop is used.
While
The while loop is only used if we dont really know how long the loop will be. Its structure is very similar to the if statements:
While cond:
expr
A simple use is when we are trying to guess a number "GuessNum" that another user has choosen:
GuessNum = 12
OurGuess = int(input("Guess the number!"))
while True:
if OurGuess != GuessNum:
OurGuess = int(input("You guessed wrong. Guess again!"))
elif OurGuess == GuessNum:
print("Finally!")
break
In this case you always have to end the loop with a break or your loop will run indefinitely. You can also see that we have to use the int() function that converts a string (the input is always a string) to a corresponding int type.
For
For loops are used when we know how long we will do something. The structure of the for loop is:
for element in iterable_object:
expr
The loop takes an iterable_object like a string a list and goes over all of its elements. If you know what is the length of the iterable_object you know how long the loop will run for. Lets look at the previous game implementation with the for loop. Lets say we only have three attempts to guess the number. After that the game is over. In principle we would the range(begin, end, step) function. it creates an iterable_object that begins with the element begin ends with end and between both limits it goes with a step of *step. You can imagine it as almost being a list. Lets show some example how it would look like a list. It is not a list though. Keep that in mind.
range(3) = [0, 1, 2]
range(end) = [0, 1, 2, 3,.... end - 1]
range(begin, end) = [begin, begin + 1, ..., end - 1]
range(2, 5) = [2, 3, 4]
range(0, 12, 3) = [0, 3, 6, 9]
range(0, 12, 2) = [0, 2, 4, 6, 8, 10]
range(0, 12, 1) = [0, 1, 2, 3, 4, 5, ..., 10, 11]
I have purposefully not written it in code form, because if you write range(3) into the python console the output is not the list I wrote. It is only a learning aid. You might have also noticed that if nothing is specified it begins with 0. This is because the first element of a list or a string in python has an index "0". The index of an element tells us on which position it is found in the list/string.
Now we can look at the example of the guessing game where you get n tries:
n = 4
GuessNum = 12
k = 0 #Amount of tries already used
for i in range(n):
OurGuess = int(input("Guess my number. You have " + str(n - k) + " tries"))
if GuessNum == OurGuess:
print("Correct!")
break
k += 1 #If you fail to guess the amount of tries used go up by one. it is a simple counter!
if k == n:
print("You failed miserably!")
This has some extra used friendliness that you can try to understand yourselves.
Functions
Now onto our last topic of todays post. Functions are probably the most useful tool at the beginning level of programming. If you truly try to understand and master functions in python you are pretty much golden. The general syntax form is:
def function(arg1, arg2, ..., argN):
body of function
return result
One inputs the name of the function himself and sets it to accept a well defined number of arguments with strict meanings. The thing you input as arg1 when you are using the function will be internally (inside the function) used in all places arg1. The definitions of things inside the function do not have any impact outside of the function. The only thing the user gets out of the function is the result. The result does not have to be just 1. It can be a list of lists. Whatever you wish. Lets illustrate how functions work with some examples:
First lets make a function that returns the sum of all the elements in the list of numbers.
def length1(lst):
sum_of_list = 0
for i in range(len(lst)):
sum_of_list = sum_of_list + lst[i]
return sum_of_list
def length2(lst):
sum_of_list = 0
for elem in lst:
sum_of_list = sum_of_list + elem
return sum_of_list
I have written two seperate functions that do the same thing. The first utilizes a counter i and constructs a iterable_object with the length of the input list that starts with 0 and goes with a step of 1. This is done by range(len(lst)). The second utilizes that lists are inherently iterative objects. The second iterates directly over the list and picks out elements one after the other.
Now lets write a function that goes over a list of numbers and finds the index of an input element we are searching for. If it does not find it, it simply warns the user.
def find(lst, x):
for i in range(len(lst)):
if lst[i] == x:
return i
print("The element is not in the list!")
return None
This function again creates an iterable_object just like the first function in the earlier example. Here this is better than using the list as an iterable object itself as we are looking for the index i. Here we also use the fact that x either is in the list and we will surely find it, or it will not be found at all. That is why we only have on if statement and no elif or even else statement. Because we must return something from the function in any case we simply return the None object if we dont find x, which is the standard practice.
Homework and Summary
So in summary what have we learned. We have learned some essentials stuff about how Python works like the fact that everything in Python is a class with its own methods and attributes. We have also learned some basic functionality that will let you do a whole lot of various things like logic and data crunching by learning if statements, loops and functions.
You can copy all of the code written in this post into your text editor and run it in a console and it should work. All of the example will also be in the GitHub repository I have made for this Guide. The link is in the Proof of Work section. There you will also upload your work done for the homework as is instructed in the repository. The descriptions of your homework can also be found in the repository.
The rewarding system was changed a little to be more objective. People who turn in their completed work until the 25. 11. will get a share of 25% of the authors rewards this post gets. The share will be divided equally among all who complete the tasks.
I am more than willing to answer any question you might have regarding this post, so please dont hesitate to ask or comment down bellow if something is unclear or if you have any questions regarding the homework.
Until next time!
hey @maticpecovnik,
Thank you for this cool tutorial! Below is our review:
z.imag
or
list1.append(list2)
, with the user knowing nothing about Python yet, those would appear a bit too weird to digest just yet.Overall, nice work !
Your contribution has been evaluated according to Utopian policies and guidelines, as well as a predefined set of questions pertaining to the category.
To view those questions and the relevant answers related to your post, click here.
Need help? Write a ticket on https://support.utopian.io/.
Chat with us on Discord.
[utopian-moderator]
Thank you very much for your input. If you dont mind I would like to respond to you bullet by bullet to explain my thinking.
In summary, thank you very much for your feedback. This has been my first contribution in @utopian-io and I am very happy about the positive feedback and constructive criticism I have received from you.
Thank you for your review, @mcfarhat! Keep up the good work!
Hello! Your post has been resteemed and upvoted by @ilovecoding because we love coding! Keep up good work! Consider upvoting this comment to support the @ilovecoding and increase your future rewards! ^_^ Steem On!
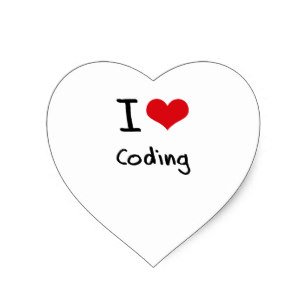
Reply !stop to disable the comment. Thanks!
Nooo, nooo no homework for weekend !!!😂 Good old days in school 🤘
Hehehehe I thought that might evoke some reactions from the people but I thought it would be quite fun anyway. Hopefully I get at least one participant. :)
Wonderful idea 👌 For sure, me and my wife will try it out tomorrow ✌️
It will be fun, because we dont know anything about it... will se how we will do :)
This post has been voted on by the SteemSTEM curation team and voting trail in collaboration with @curie.
If you appreciate the work we are doing then consider voting both projects for witness by selecting stem.witness and curie!
For additional information please join us on the SteemSTEM discord and to get to know the rest of the community!
Hi @maticpecovnik!
Your post was upvoted by @steem-ua, new Steem dApp, using UserAuthority for algorithmic post curation!
Your post is eligible for our upvote, thanks to our collaboration with @utopian-io!
Feel free to join our @steem-ua Discord server
Congratulations @maticpecovnik! You have completed the following achievement on the Steem blockchain and have been rewarded with new badge(s) :
Click here to view your Board of Honor
If you no longer want to receive notifications, reply to this comment with the word
STOP
To support your work, I also upvoted your post!
Do not miss the last post from @steemitboard:
Hey, @maticpecovnik!
Thanks for contributing on Utopian.
We’re already looking forward to your next contribution!
Get higher incentives and support Utopian.io!
Simply set @utopian.pay as a 5% (or higher) payout beneficiary on your contribution post (via SteemPlus or Steeditor).
Want to chat? Join us on Discord https://discord.gg/h52nFrV.
Vote for Utopian Witness!
Hi, @maticpecovnik!
You just got a 0.33% upvote from SteemPlus!
To get higher upvotes, earn more SteemPlus Points (SPP). On your Steemit wallet, check your SPP balance and click on "How to earn SPP?" to find out all the ways to earn.
If you're not using SteemPlus yet, please check our last posts in here to see the many ways in which SteemPlus can improve your Steem experience on Steemit and Busy.