Coding FreeCodeCamp's Wikipedia viewer project using Jquery and the Wikipedia API
Repo: https://github.com/jquery/jquery
Using Jquery and the Wikipedia API to create a Wikipedia search web app
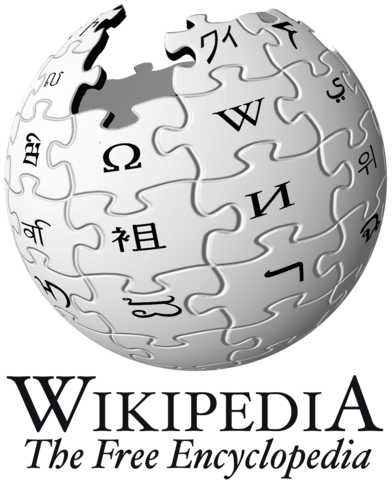
In this tutorial I will walk you through coding FreeCodeCamp’s Wikipedia viewer project. The project consists of creating a search bar that allows the user to search for a term which is sent to the Wikipedia API and returns relevant Wikipedia articles for the search. The project also includes a link that will direct the user to a random Wikipedia article.
This project will teach you the basics of sending an AJAX request to an API and displaying the data returned.
What you will learn:
- How to work with the Wikipedia API
- Making an AJAX request with Jquery
- Displaying Wikipedia articles from the API
Requirements:
Difficulty:
- Easy to Intermediate
The Project:
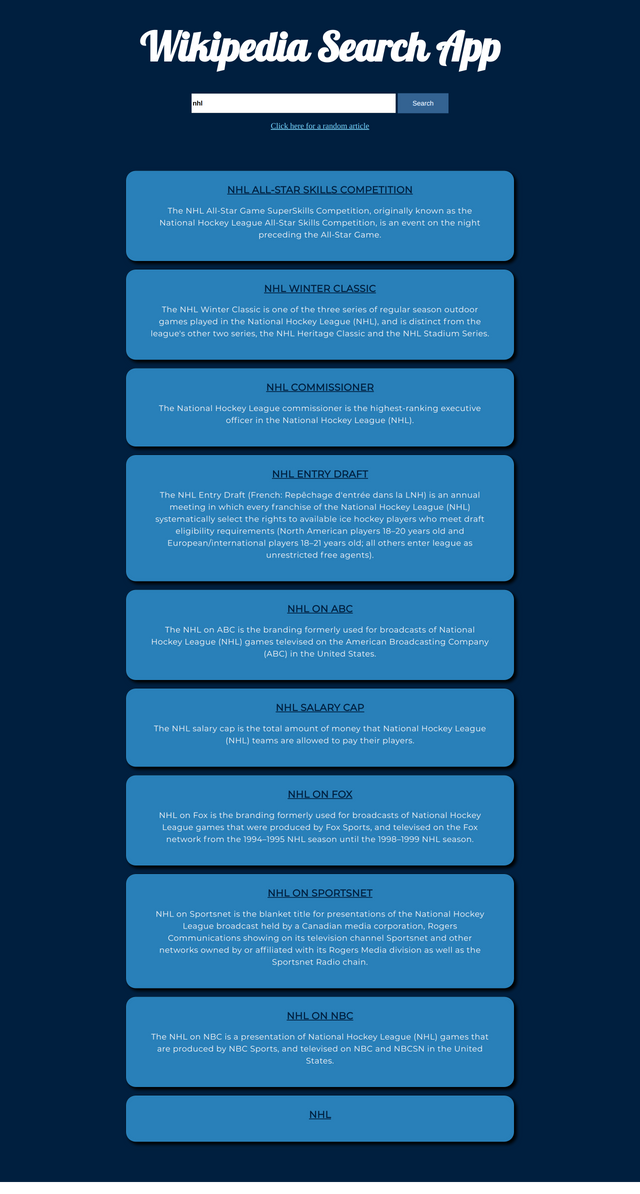
The code in it’s entirety:
HTML file
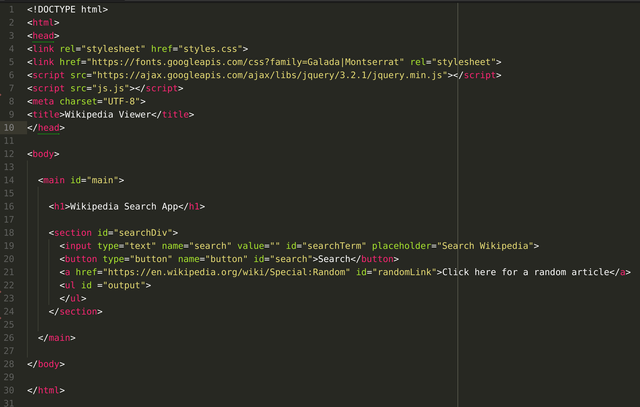
Javascript file
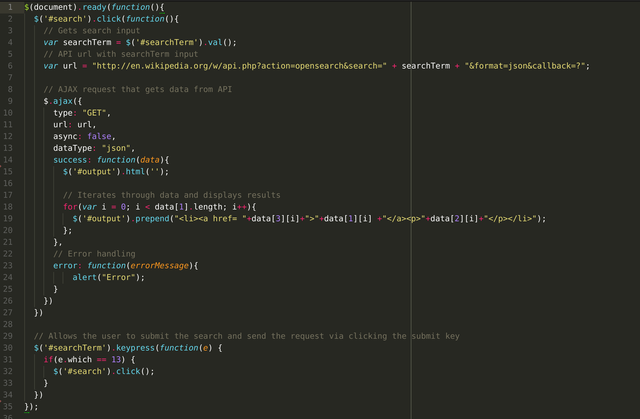
Let's get started:
In our Javascript file we start our Jquery with :
$(document).ready(function(){
});
This ensures that our Jquery code doesn’t execute until the Document Object Model (DOM) is properly loaded. This helps us avoid any potential issues with the DOM and Jquery. The rest of our code in our Javascript file will be nested in this function.
The next function we call within our document.ready function is :
$('#search').click(function(){
})
This function uses the Jquery selector to select the HTML search element we created in our HTML file. We call the Jquery click function on this so that this function is called when the search button is clicked.
The next step is to create a variable with the Wikipedia API URL stored in it:
var url = "http://en.wikipedia.org/w/api.php?action=opensearch&search=" + searchTerm + "&format=json&callback=?";
Query | What it does | Our Value |
---|---|---|
action | Tells the API what action we want | opensearch |
search | Gives the API the term we are searching for | searchTerm variable we created |
format | Tells the API what format the data should be in | json |
After creating the url variable with our Wikipedia API endpoint stored in it the next step is to call the API via an AJAX call:
$.ajax({
type: "GET",
url: url,
async: false,
dataType: "json",
success: function(data){
$('#output').html('');
for(var i = 0; i < data[1].length; i++){
$('#output').prepend("<li><a href= "+data[3][i]+">"+data[1][i] +"</a><p>"+data[2][i]+"</p></li>");
};
},
error: function(errorMessage){
alert("Error");
}
})
The AJAX request consists of several parts:
Parameter | What it does | Our Value |
---|---|---|
type | The type of request (GET or POST) | "GET" |
url | The url we are sending the request to | url |
async | Whether the request is asynchronous or not | false |
dataType | The data type of the request | "json" |
success | Function that handles what to do if the request is successful | success function |
error | Function that handles what to do if the request is unsuccessful | error function |
The success function that handles what to do in the event that the AJAX request is successful looks like this :
success: function(data){
$('#output').html('');
for(var i = 0; i < data[1].length; i++){
$('#output').prepend("<li><a href= "+data[3][i]+">"+data[1][i] +"</a><p>"+data[2][i]+"</p></li>");
};
},
First the unordered list HTML element with the ID output is selected and we use the html method to set it to empty (‘’). Next a for loop is used to iterate through the JSON data we are retrieving from the API and then we use the prepend method to prepend the data we want and display it in the output unordered list in our HTML.
data[3] selects the links for each result, data[1] selects the title for each article, and data[2] selects the text content for each article.
The error function handles what we want to do in the event there is an error in our AJAX response :
error: function(errorMessage){
alert("Error");
}
For the sake of simplicity we will launch a very basic alert message that lets us know there was an error.
At this stage in our code the AJAX request is able to send a request to the API and properly handle how to display the data. However, our search bar only works if we use the mouse to click on the search button. If we attempt to do a search and submit the search term by hitting the enter key on a keyboard nothing happens.
Let’s fix this with the keypress method:
$('#searchTerm').keypress(function(e) {
if(e.which == 13) {
$('#search').click();
}
})
First we select the search bar by selecting searchTerm and then we call the keypress method on it. Next the which method is used to to determine which key on the keyboard is being pressed. The number that represents the enter key on a keyboard is 13, so we use an if statement to determine if the enter key is being pressed. If the enter key is in fact being pressed then we call the click method on the search element and this submits the search the same way it would if we clicked the search button.
Our Javascript file is now finished and we have a fully functional search bar that allows the user to pull results from the Wikipedia API and properly displays them in an unordered list.
Wrapping up:
This concludes my tutorial on FreecodeCamp’s Wikipedia viewer project. I hope you learned how to work with the Wikipedia API and how to send out an AJAX request and display the retrieved JSON data with Jquery.
I did not include the CSS file for the styling of this project due to the fact that I wanted to focus primarily on the AJAX request and API. The full project, including the stylesheet, can be found here.
@nicnyr Great job
Plz remember to put your github account at the end.
And a little advise from me that at the above portion you have used some coding which isin the form of pics, try to type the code in the post, so it will make more easier for user if they want that code.
Thank you for your contribution.
Few suggestions for your future work:
Your contribution has been evaluated according to Utopian policies and guidelines, as well as a predefined set of questions pertaining to the category.
To view those questions and the relevant answers related to your post, click here.
Need help? Write a ticket on https://support.utopian.io/.
Chat with us on Discord.
[utopian-moderator]
Hey @nicknyr
Thanks for contributing on Utopian.
We’re already looking forward to your next contribution!
Contributing on Utopian
Learn how to contribute on our website or by watching this tutorial on Youtube.
Want to chat? Join us on Discord https://discord.gg/h52nFrV.
Vote for Utopian Witness!
Congratulations @nicknyr! You have completed some achievement on Steemit and have been rewarded with new badge(s) :
Click on the badge to view your Board of Honor.
If you no longer want to receive notifications, reply to this comment with the word
STOP