Build A Blog Site With React, Redux Firebase And MaterializeCSS (Part 12)
Repository
React
https://github.com/facebook/react
Material-ui
https://github.com/mui-org/material-ui
My Github Address
This Project Github Address
https://github.com/pckurdu/Build-A-Blog-Site-With-React-Redux-Firebase-And-MaterializeCSS-Part-12
What Will I Learn?
- You will learn how to access the desired collection using
firebaseReducer
. - You will learn
useFirestoreForProfile
in firebaseReducer. - You will learn
userProfile
in firebaseReducer. - You will learn how to format the date with the
moment
packages. - You will learn
calendar()
in moment. - You will learn about
getState()
in redux.
Requirements
- text editor (I used visual studio code editor)
- Basic javascript information
- Basic react information
Difficulty
- Basic
Tutorial Contents
In this tutorial we will consider how to access the profile property in firebaseReducer
.
We can access many features in firebase with firebaseReducer. One of these features is the profile
feature. We can export the collection we want in firebase to the profile feature in firebaseReducer. So that we can access collections very easily using the profile feature within the application.
We did not take profile properties in some places in application. In this tutorial, we will fill the fixed places with their counterparts in the firebase and we will make the necessary arrangements.
Separating this tutorial into the following sections will make it easier to follow:
- Activate The Profile Property
- Edit the projectActions File
- Editing Projects By Profile Property
- Use Moment Package
1- Activate The Profile Property
In this section, we will learn the process of transferring users collection to the profile feature in firebaseReducer.
We use the SignedInLinks
component in the Navbar
component. We need the Navbar component's users collection because we need the information of the logged in user here.
Let's examine firebaseReducer in the Navbar component.
In Navbar.js
//to be used in connect.
const mapStateToProps=(state)=>{
console.log(state);
//have to be return
return {
auth:state.firebase.auth
}
}
The isEmpty
and isLoaded
properties in the firebase profile come true
when the user is not logged in.
When the user logs in, we observe that the isLoaded is false. We can find that the user is logged in even from the change of this feature.
The profile feature is not limited to these features only. By activating the useFirestoreForProfile
property, we can transfer collections to the profile. After activating this feature, we can use the collection we want to define the userProfile
property.
We need to do this where we define the reactReduxFirebase
module. We need to send these properties to the reactReduxFirebase module as objects.
In index.js
//import the users collection into the profile property.
reactReduxFirebase(firebaseConfig,{attachAuthIsReady:true,useFirestoreForProfile:true,userProfile:'users'})
Users colection will now have profile features in the profile.
Now that we have access to the user's features, we can now use them in the required fields.
First, we create an object called profile within the mapStateToProps in Navbar component. We transfer the profile object in the firebase to this object.
In Navbar.js
//to be used in connect.
const mapStateToProps=(state)=>{
console.log(state);
//have to be return
return {
auth:state.firebase.auth,
//We are accessing the profile object in firebaseReducer.
profile:state.firebase.profile
}
}
Now the profile object will be contained within the component's props then we will send the props to the SignedInLinks component.
const {auth,profile}=props
//We send the profile to the SignedInLinks component.
const links=auth.uid?<SignedInLinks profile={profile}/>:<SignedOutLinks/>
Within the SignedInLinks component we can use the user's monogram
properties.
<li><NavLink to='/' className="btn btn-floating red">
{props.profile.monogram}
</NavLink></li>
Let's test the change when we add a new user.
2- Edit the projectActions File
In this section, we'll edit the properties we've added as a constant when adding projects.
Since we keep the user's name and surname in the users collection, let us store the surname of the user who added the project in the projects collection.
We will bring the user's name and surname from the firebase.profile
field. We will bring the user id property firebase.auth property.
To do this, we have defined getState
in the projectActions file. With the getState feature, we can access rootReducer, where we can access the reducers with the prefix names of the reducer.
In projectActions.js
//We get the profile and uid values.
const profile=getState().firebase.profile;
const authorId=getState().firebase.auth.uid;
Let us use these variables in the project addition stage.
//We are adding data to the projects collection.
firestore.collection('projects').add({
...project,
authorName:profile.firstName,
authorLastName:profile.lastName,
authorId:authorId,
createdAt:new Date()
Thus, we are able to capture the information of the user who entered the project.
Let's test by adding a project.
We have implemented the project with a steemit user, but when we examine the project we added, it is seen as adding pckurdu. This is because we haven't corrected the places where the project was displayed in more applications.
When we check the firebase, we see that the project has been added correctly.
3- Editing Projects By Profile Property
In this section, we display the projects on the pages of the user name of the firebase will perform the process.
We can make the necessary corrections in the ProjectSummary
file. We had previously transferred these component project properties. The only thing we do is to edit the data that comes with the project object.
In ProjectSummary.js
<div>Posted by {project.authorName} {project.authorLastName}</div>
We can do the same in the ProjectDetails page.
In ProjectDetails.js
<p>Posted by The {project.authorName} {project.authorLastName}</p>
Thus we have edited the user name and in this section, let's perform the page orientation when adding the project.
In CreateProject.js
//for routing
this.props.history.push('/');
Thus, when the project is added, it will be directed to the main page.
4- Use Moment Package
When creating the project we were taking the current date and registering it in the firebase. In this section we will access the date in the firebase and show it on the project detail page.
We can show the date using the toDate()
function. In the ProjectSummary
component, let's access and show the date with createdAt
in the project object.
In ProjectSummary.js
{/* get date */}
<p className="grey-text">{project.createdAt.toDate().toString()}</p>
The date created, users will not be understood. We need to format for users to understand.
We can create more understandable dates using moment
packages. First, we have to download the moment packages with the code we will write on the command line.
yarn add moment
Then show the time since the date created using the calendar()
function.
import moment from 'moment'
…
{/* get date */}
<p className="greytext">
{moment(project.createdAt.toDate().toString()).calendar()}
</p>
Since we need to format date in ProjectDetails.js
, let's do the same in there.
Thus we have created a more clear date.
Curriculum
Part 1 - Part 2 - Part 3 - Part 4 - Part 5 - Part 6 - Part 7 - Part 8 - Part 9 - Part 10 - Part 11
Proof of Work Done
https://github.com/pckurdu/Build-A-Blog-Site-With-React-Redux-Firebase-And-MaterializeCSS-Part-12
Thank you for your contribution @pckurdu.
After reviewing your tutorial we suggest the following points listed below:
Your tutorial is very well explained and structured.
The GIF where you show what is in the inspector we can not see well what is in the console. We suggest that when you want to demonstrate the inspector only put images.
The forum tutorial would look interesting if you had a password recovery section and a user profile area to put your account details.
Thank you for your work in developing this tutorial.
Looking forward to your upcoming tutorials.
Your contribution has been evaluated according to Utopian policies and guidelines, as well as a predefined set of questions pertaining to the category.
To view those questions and the relevant answers related to your post, click here.
Need help? Chat with us on Discord.
[utopian-moderator]
Thank you for your review, @portugalcoin! Keep up the good work!
Congratulations! Your post has been selected as a daily Steemit truffle! It is listed on rank 3 of all contributions awarded today. You can find the TOP DAILY TRUFFLE PICKS HERE.
I upvoted your contribution because to my mind your post is at least 16 SBD worth and should receive 239 votes. It's now up to the lovely Steemit community to make this come true.
I am
TrufflePig
, an Artificial Intelligence Bot that helps minnows and content curators using Machine Learning. If you are curious how I select content, you can find an explanation here!Have a nice day and sincerely yours,
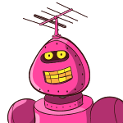
TrufflePig
Hi @pckurdu!
Your post was upvoted by @steem-ua, new Steem dApp, using UserAuthority for algorithmic post curation!
Your post is eligible for our upvote, thanks to our collaboration with @utopian-io!
Feel free to join our @steem-ua Discord server
Hey, @pckurdu!
Thanks for contributing on Utopian.
We’re already looking forward to your next contribution!
Get higher incentives and support Utopian.io!
Simply set @utopian.pay as a 5% (or higher) payout beneficiary on your contribution post (via SteemPlus or Steeditor).
Want to chat? Join us on Discord https://discord.gg/h52nFrV.
Vote for Utopian Witness!