Creating a Website With Django Using Python 3.6 (Part 3) | Setting Up The Database And Models
Creating a Website With Django Using Python 3.6 (Part 3)
Welcome to another tutorial of my series and in the last tutorial, we learned about lots of things. In this tutorial, we will be setting up our database so we can use it on the website in the future. Some of the uses of the database include the addition of albums and songs by different artists. We need to learn a couple of core concepts of Django first before setting up the database and we'll learn about them in this tutorial.
Repository
https://github.com/django/django
In the following tutorial, you will learn these things.
- Learning about default database that comes with Django
- Creating Models
- Learning about Primary Key and Foreign Key
- Activating Database
- Fixing the migration issue
Requirements
To fully understand the concepts you must have basic knowledge of Python. I am going to be using Python 3.6 in this tutorial. Here are few things that you will need installed.
- Python 3.6 (Link at the end of the tutorial)
- Pip (Link at the end of the tutorial)
- Django
- Code Editor
- Ubuntu 16.04 LTS
The code editor is not necessary but it can make your life a lot easier. Some of the good code editors include Atom and PyCharm. I will be using PyCharm for this series because it is designed for mostly Python and it is good for beginners.
Difficulty Level
- Intermediate
Tutorial
Learning about default database that comes with Django
We remember that when we set up our project to use Django, it came with few core files but may not be clear at first but they all are working together at back end. The Django comes with default database which is SQLite3 which can be found in main folder which contains all the files. If you open the settings file of the WebApp and go to line 76 or 77 you can see the default database is set to be SQLite3.
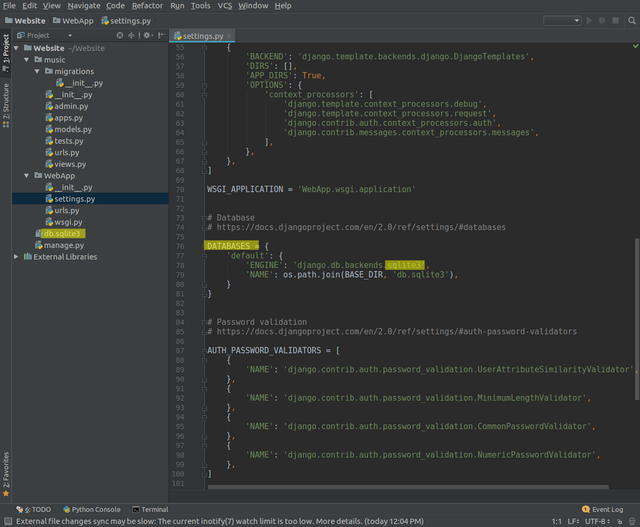
The good thing about Django is that it can use any database like MySQL and those type of databases are something that you will use in production but just for testing, sqlite3 is the best choice as of now. It's really cool that it comes with it by default and this saves us a lot of time because we don't have to do a lot of work but by not to do lot of work I don't mean we have to do any work because as of right now the database is not connected to our apps and it is outside of our project basically but Django know that it exists, so still we need to configure to work with our code. When we install Django it comes with a bunch of default apps that can be seen in line 33 of the settings file.
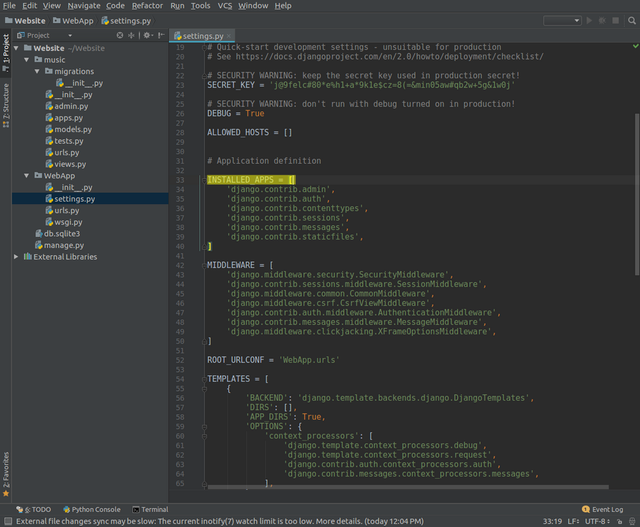
Some of them are
- Admin
- Authentication
- Content Types
- Session
- Messages
- Static Files
We'll work with them later but behind the scenes, Django is already to work with bunch of default apps. Some of these apps need to access database and most work is done for us.
Recap of what models are
A model is like a blueprint for your database. It stores all the information like how you want to store data for this app in the database. All the tables, columns and rows that we will use in the database for this app will be found here and you obviously we'll be adding more settings in the future. There isn't much code in the file as well. In the future we will create two classes in this file, one will be for the albums and another one will be for the songs so that's the blueprint of how we are gonna set up our database.
Creating Models
The first question is that what kind of data are we going to be saving. We are going to be saving songs and albums and then each album will have an artist, genres and all sorts of stuff. Some of you will be now thinking are we going to be creating the blueprint for the database or our code. Here's a cool thing about Django, whenever you create the models in Django, it's the same thing.
You don't have to make one for your database and one for your code. You just make a class in the models.py file of music and then automatically Django will get each variable from those class and convert it to a column in your database. So let's just open the models.py file from music app directory and create our first model. Type or copy the code given below and paste it under the comment that is present in the file.
Code
class Album(models.Model):
artist = models.CharField(max_length=250)
album_title = models.CharField(max_length=500)
genre = models.CharField(max_length=100)
album_logo = models.CharField(max_length=1000)
Explanation of the code
As I said earlier we need to create a class and we are gonna name our class "Album" which obviously means that we are going to create this for albums and it is gonna inherit from models. Model. Every class for the model will be inherited from it. An album surely has an artist who has sung the song. Let's create a variable artist and assign it to something. There is one thing you need note is that we need to tell the Django whether our column is going to be text, float and integer and I think you all must know that the name of a person is in text or characters, so we typed models.CharField(). Inside the parameter we have written max length for the text, it is not necessary but I will recommend you to set it up for each variable.
The max length for the artist's name can be 250 characters, I am sure no artist's name is that long. We have done same things for other variables so it is self-explanatory. We have also added album logo and logo for the album (for now) will be links that we can find on the web but in the future, I will show you how you can set up to upload the files to the database. Here's our first model and again each variable in this class like "artist" will represent a column in the database. Let's create another model for Song. Just type or copy the below code underneath the last block of code but before it here's a screenshot of the code placed inside the code editor in case you get indentation errors or don't know how to place the code.
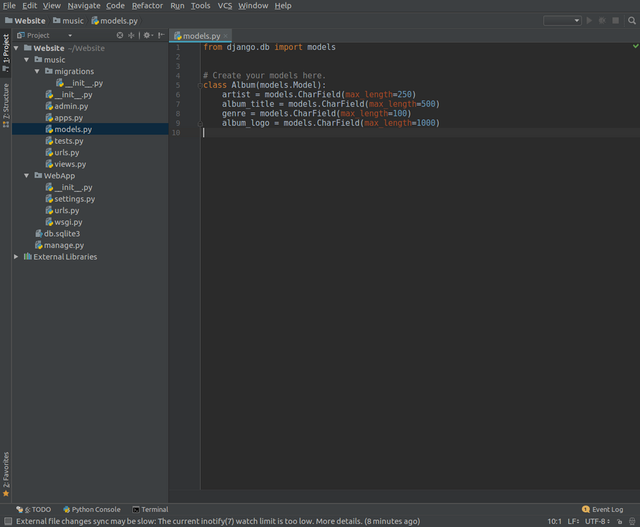
Code
class Song(models.Model):
album = models.ForeignKey(Album, on_delete=models.CASCADE)
file_type = models.CharField(max_length=10)
song_title = models.CharField(max_length=250)
Explanation of the code
We needed to associate both classes in some kind of way. We can actually create as many albums as we want but for songs, the case is not going to be same. For songs, each song must be a part of an album or in other words, each song will be associated with an album. We have will have up to 13 - 15 songs per album. You'll be thinking how can we associate songs with an album in the way we want. We have done this in the second line of code. To understand what he has done we first need to explain the concept of primary key and foreign key.
Learning about Primary Key
You should remember that I told you that each variable in this models represents a column but in the backend, there is another column being made and that column is a unique id number. In our first class or model, the id will be of 1 because it is the first model. In the computer language, the counting starts from 0 but that is not the case in this, the first model or class will have the id of 1 and yes the second model will have an id of 2. You may be wondering why does it do that, we can pick out an album by typing the name of the album. Let's use album Ed Sheeran album "X" for this example, what if we want information for the album of Ed Sheeran but there is another band who also have an album named "X", this can create confusion. This will become if you have all these pieces of information but you don't know which one to specify. That id is known as Unique or Primary Key.
Learning about Foreign Key
Let's say album "X" has a primary key of 1 and Ed Sheeran has a song called Photograph of that album but we need to link it to an album. The foreign key for this song is just gonna be 1 and that way we know that this song is linked with an album that has a primary key which is 1 as well. Again, a primary key is needed whenever you have a bunch of elements and each needs a unique id number and on the other side, a foreign key is used whenever you have something like a song that's part of something else like an album in our case.
Moving on with the explanation
In the first line of the code, we are setting the variable "album" to a foreign key. There's another thing you might not know and that thing is "on_delete". Since we have a lot of songs and they need to be a part of an album, so what happens whenever we delete an album. We can't have a bunch of songs just lying around because that doesn't make sense. So to fix this issue we have put a code "on_delete=models.CASCADE" which means whenever the album is deleted the songs are deleted with it as well so we won't have to delete each song one by one. In the next lines, we have just created two variables that are going to be strings, texts or whatever you want it to call.
The max length of the file format should be 10 characters, I don't think there's any file format even close to that. Now our basic models are completed and they are looking good. I want to paste the screenshot also below in case you are stuck with indentation errors or anything.
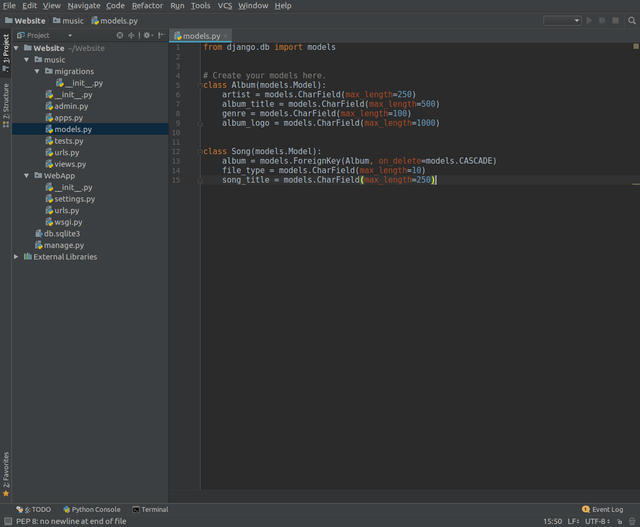
Activating Database
You should remember that I said that Django checks all the apps from the settings.py file which can be found in WebApp folder. Let's place our app inside there so Django can check if our app is synchronized with the database. To do this type this in the list of installed apps.
'music.apps.MusicConfig',
What it actually is checking is the apps file in music directory and if you want to be more specific, it is checking MusicConfig class inside it but you can basically just say that it means that music app. Here's the screenshot if you are stuck.
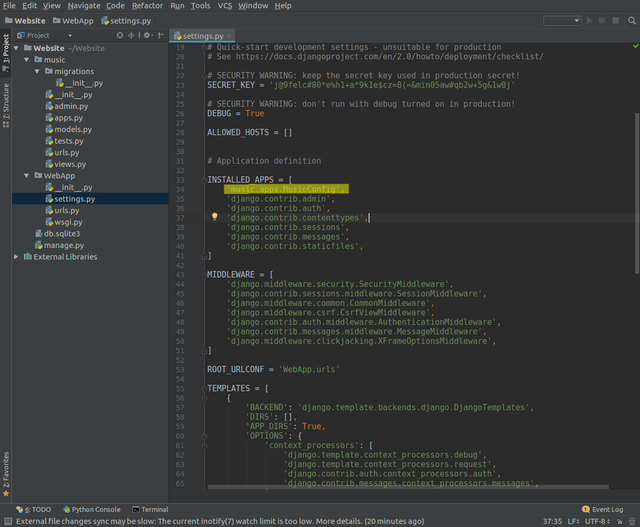
Fixing the migration issue
You must be thinking now that everything looks good, we have our database ready and we have added our app. The reality is if we try to run the server now it will show a migration issue. The Django checks all the apps that can be found in installed apps and then goes to models.py of that app. In the models of that app, it checks the models and compares it to the database to check if the database is synchronized with the models. Before fixing the issue, let's actually take a look at the issue. You can do this by trying to run the server which we can by typing python manage.py runserver
we need to make sure that we are in the directory of the website. After seeing the error you can fix it by typing python manage.py makemigrations music
.
Most we are doing right now is telling Django that we have made changes to the music app and again make sure you are in the directory of the file before running the code. If you still not clear about what migrations are then here's a quick explanation. Migrations are like a commit in which Django converts our models into table in the database and keeps it synchronized. There is still one last thing that we need to do is to apply the migrations. We created or made the migrations before now let's apply them by typing python manage.py migrate
like done in the screenshot below.
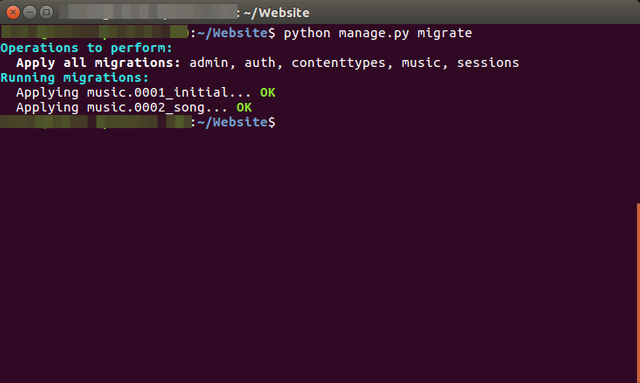
Running the server
Now that we have everything set up, we can actually run the server by typing python manage.py runserver
and it will be running without any error like in the image provided below.
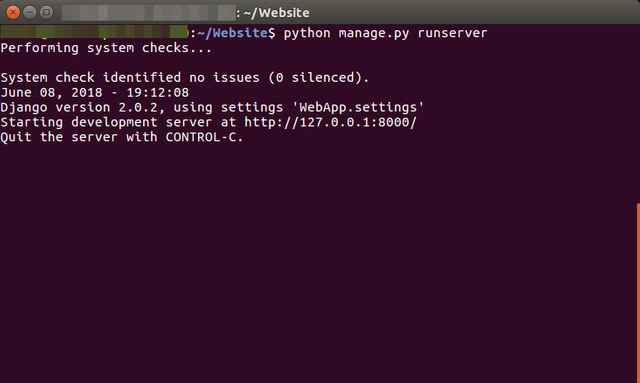
Curriculum
- Creating a Website With Django Using Python 3.6 (Part 2)
- Creating a Website With Django Using Python 3.6 (Part 1)
Go here https://steemit.com/@a-a-a to get your post resteemed to over 72,000 followers.
Uncomplicated article. I learned a lot of interesting and cognitive. I'm screwed up with you, I'll be glad to reciprocal subscription))
Congratulations @reinhardtation! You received a personal award!
You can view your badges on your Steem Board and compare to others on the Steem Ranking
Vote for @Steemitboard as a witness to get one more award and increased upvotes!