[Java Tutorial #3] - How to create GUI java aplication for displaying Steemit account data using Netbeans
Repository
https://github.com/dmlloyd/openjdk
What Will I Learn?
- You will learn How to create GUI form for this aplication
- You will learn how to get data from Steemit URL API
- You will learn how to split data
- You will learn how to display data on GUI aplication
Requirements
- Basic knowledges about java programing
- Basic Knowledges about API
- Need to Install Netbeans for easy pratice
Difficulty
- Basic
Tutorial Contents
In the previous tutorial, I've explained how to create GUI java aplication to display LIVE SBD price. And in this tutorial I will try to create GUI aplication to display steemit accout data. When we fill name of the steemit account in textfield and press the search button will exit the account details such as reputation, sbd number, vest number, number of voting power and date created.
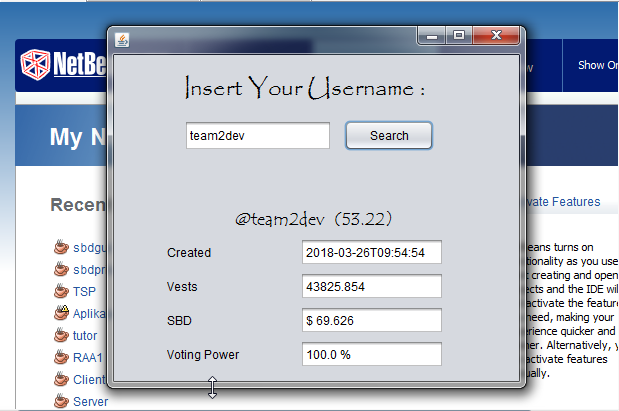
Let's start the tutorial :
Open Netbeans IDE software and create new project. Choose Java Aplication and click next. Then Rename the project and click finish.
Add new jFrameForm in your Source Package
Rename the form
Click on panel component and drag it to the form
Add Label, TextField and Button on Panel, just follow picture bellow :
We already have a form, Now open
source
and start to edit the code.
To get data from steemit API we need curl an url API. Here I use URL API from https://helloacm.com/tools/steemit/. and I use get Accout Report API. This the URL :
We already have an URL api, Now create a method to get data from that url. Open the method under
initComponents();
public void getData()throws Exception{
}
- get Text from url username
public void getData()throws Exception{
String Username=userName.getText();
}
- Now, add the username to URL. and start to curl the url.
public void getData()throws Exception{
String Username=userName.getText();
URL oracle = new URL("https://uploadbeta.com/api/steemit/account/profile/?cached&id="+Username);
HttpURLConnection httpcon = (HttpURLConnection) oracle.openConnection();
httpcon.addRequestProperty("User-Agent", "Mozilla/5.0");
BufferedReader in = new BufferedReader(new InputStreamReader(httpcon.getInputStream()));
Code | Explanation |
---|---|
getData() | Method Name |
throws JSONException | This code to handle the error from JSON |
String Username=userName.getText(); | Get data from user input. |
="+Username | combine username with url |
HttpURLConnection httpcon = (HttpURLConnection) oracle.openConnection(); | class initiation to get URL connection. |
httpcon.addRequestProperty("User-Agent", "Mozilla/5.0"); | Add browser property, Here I add Mozilla 5.-0 |
' BufferedReader in = new BufferedReader(new InputStreamReader(httpcon.getInputStream()));` | method Read input from http connection. |
- To add httpconnnection class and buffared reader you need to import following package :
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
import java.util.logging.Level;
import java.util.logging.Logger;
- Add a string variable to receive the result data, and to dispay it we need open
while
looping.
while ((inputLine = in.readLine()) != null){
System.out.println(inputLine);
}
in.close();
- and try to run aplication. Input some user and press search button.
{"steem": 18.469, "value": 124.48064683007745, "vp": 100, "esp": {"vesting_sp": 21.562002306883915, "received_sp": 0.0, "esp": 21.562002306883915, "delegated_vesting_shares": 0.0, "received_vesting_shares": 0.0, "delegated_sp": 0.0, "vesting_shares": 43825.854103, "effective_vesting_shares": 43825.854103}, "payout7": [{"steem": 0.4, "sbd": 12.51, "sp": 5.658246214390056, "name": "team2dev", "vests": 11500.67}], "sbd": 69.626, "created": "2018-03-26T09:54:54", "online": 73, "rep": 53.22, "id": "team2dev", "last_vote_time": "2018-06-04T18:24:57", "vests": 43825.854, "ticker": {"latest": "1.37033861980282889", "sbd_volume": "5343.396 SBD", "steem_volume": "3867.142 STEEM", "lowest_ask": "1.37030479322119647", "highest_bid": "1.37030357679591219", "percent_change": "-2.13153221250492519"}}
- We already get data, Now we need to display it on GUI form. Here we will just display name, reputation, created date, amout of SBD, vest and voting power. To split this data we need convert it to JSON object. Before that import the json package.
import org.json.JSONObject;
- TO use JSONObject here we need to add java-json Library. Dowload it here : http://www.java2s.com/Code/Jar/j/Downloadjavajsonjar.htm . Extract and add to project Libraries. Then import it to your file.
- Convert data that we get in string to Object
JSONObject obj = new JSONObject(inputLine);
- Then Display it to GUI form
userNrep.setText("@"+obj.getString("id")+" ("+obj.getDouble("rep")+")");
created.setText(obj.getString("created"));
Vests.setText(""+obj.getDouble("vests"));
SBD.setText("$ "+obj.getDouble("sbd"));
VP.setText(obj.getDouble("vp")+" %");
Code | Explanation |
---|---|
setText | Method to setText on GUI |
getString("created")); | get String from created object, why we use getString() ? because the value is a string |
`getDouble() | get double value from the object |
in.close(); | close connection. |
- Finish. Now try to run aplication.
Curriculum
[Java Tutorial] - How to create some java aplication to display Live SBD price from bittrex API using Netbeans
[Java Tutorial #2] - How to create GUI aplication for displaying Live SBD price using Netbeans
Proof of Work Done
https://gist.github.com/team2dev/e11ae7a2af174114c1fa847f1acf4cd5
Get your post resteemed to 72,000 followers. Go here https://steemit.com/@a-a-a
Thank you for your contribution.
While I liked the content of your contribution, I would still like to extend few advices for your upcoming contributions:
Looking forward to your upcoming tutorials.
Your contribution has been evaluated according to Utopian policies and guidelines, as well as a predefined set of questions pertaining to the category.
To view those questions and the relevant answers related to your post, click here.
Need help? Write a ticket on https://support.utopian.io/.
Chat with us on Discord.
[utopian-moderator]
Hey @team2dev
Thanks for contributing on Utopian.
We’re already looking forward to your next contribution!
Contributing on Utopian
Learn how to contribute on our website or by watching this tutorial on Youtube.
Want to chat? Join us on Discord https://discord.gg/h52nFrV.
Vote for Utopian Witness!