[Java Tutorial #5] - How to create GUI java aplication for checking The Estimated Reward in Last Week using Netbeans
Repository
https://github.com/dmlloyd/openjdk
What Will I Learn?
- You will learn How to create GUI form for this aplication
- You will learn API to get Estimated reward Data
- You will learn how to split data or take the important data from API
- You will learn how to dislay data to the form
Requirements
- Basic knowledges about java programing
- Basic Knowledges about API
- Need to Install Netbeans for easy pratice
Difficulty
- Basic
Tutorial Contents
In this tutorial I will try to explain how to create a java application to check the amount of rewards received by some author in last week. This reward consists of SBD, STEEM, STEEM POWER and VEST that get by some author in a week ago. To create this aplication we need to grab the data from steemit, and in this tutorial I use API from https://helloacm.com/tools/steemit/ . You can see the aplication on image bellow :
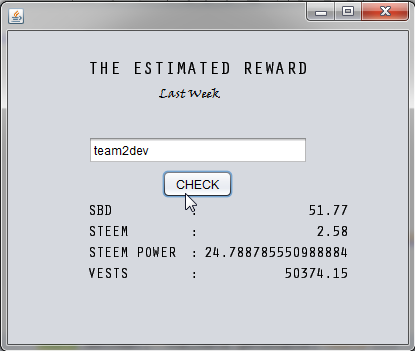
Let's start the tutorial :
Open Netbeans IDE software and create new project. Choose Java Aplication and click next. Then Rename the project and click finish.
Add new jFrameForm in your Source Package
Rename the form
Click on panel component and drag it to the form
Add Label, TextField and Button on Panel, just follow picture bellow :
here the component detail:
We already have a form, Now open
source
and start to edit the code.Create The method to get Data from URL. This method in
java.net.*
method. So before your start use this method import the package first
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
import java.util.logging.Level;
import java.util.logging.Logger;
- Then Create
getApi()
method.
public String getApi(String url) throws Exception{
URL oracle=new URL(url);
HttpURLConnection httpcon = (HttpURLConnection) oracle.openConnection();
httpcon.addRequestProperty("User-Agent", "Mozilla/5.0");
BufferedReader in = new BufferedReader(new InputStreamReader(httpcon.getInputStream()));
String inputLine;
while ((inputLine = in.readLine()) != null){
return inputLine;
}
in.close();
return null;
}
Code | Explanation |
---|---|
getApi() | Method Name |
String url | The Parameter |
throws Exception | Some method to catch error |
URL oracle=new URL(url); | Decrlarating new URL |
HttpURLConnection httpcon = (HttpURLConnection) oracle.openConnection(); | class initiation to get URL connection. |
httpcon.addRequestProperty("User-Agent", "Mozilla/5.0"); | Add browser property, Here I add Mozilla 5.-0 |
BufferedReader in = new BufferedReader(new InputStreamReader(httpcon.getInputStream())); | method Read input from http connection. |
return inputLine; | return the output |
in.close(); | closie input stream reader |
- Now create the method to get Data from url and count the price. Here I rename with
getData()
method.
public void getData() throws Exception{
}
- Get The author name from user input.
String Author=UserName.getText();
- Call get Data method with parameter is the API URL. The API URL is from https://helloacm.com/tools/steemit/ , you can get more API there :
String Data = getApi("https://uploadbeta.com/api/steemit/payout7/?cached&id="+Author);
- To get the important data from result we need split it. Here we need use java-json library. Dowload it here : http://www.java2s.com/Code/Jar/j/Downloadjavajsonjar.htm . Extract and add to project Libraries. Then import it to your file.
import org.json.JSONArray;
import org.json.JSONObject;
- Because the Data is in Array form(you see on image bellow) so we need to convert it to JSONArray
JSONArray ArrData=new JSONArray(Data);
- Now, Convert it to JSONObject
JSONObject ObjData=ArrData.getJSONObject(0);
- Get Some element of object and display it on the form
sbd.setText(""+ObjData.getDouble("sbd"));
steem.setText(""+ObjData.getDouble("steem"));
sp.setText(""+ObjData.getDouble("sp"));
vests.setText(""+ObjData.getDouble("vests"));
Code | Explanation |
---|---|
ObjData.getDouble("sbd") | we use get Double because the value of sbd element in data is in double type. |
""+ | we combine the text with double to convert double to string because the setText method need parameter in string type. |
- Call the
getData()
method in button. Open the aplication design form and double click on button. then add this code.
try {
getData();
} catch (Exception ex) {
Logger.getLogger(Reward.class.getName()).log(Level.SEVERE, null, ex);
}
Now try to run the aplication, and input some author name then press
check
button
Finish, the aplication is running rightly. You can get the full code on my gist. The link is bellow
Curriculum
- [Java Tutorial] - How to create some java aplication to display Live SBD price from bittrex API using Netbeans
- [Java Tutorial #2] - How to create GUI aplication for displaying Live SBD price using Netbeans
- [Java Tutorial #3] - How to create GUI java aplication for displaying Steemit account data using Netbeans
- [Java Tutorial #4] - How to create Calculator java aplication to convert STEEM to IDR using Netbeans
Proof of Work Done
https://gist.github.com/team2dev/242a87b4bf2b9f7d7c5d3653bb3c9e6d
Go here https://steemit.com/@a-a-a to get your post resteemed to over 72,000 followers.
Thank you for your contribution.
Your contribution has been evaluated according to Utopian policies and guidelines, as well as a predefined set of questions pertaining to the category.
To view those questions and the relevant answers related to your post, click here.
Need help? Write a ticket on https://support.utopian.io/.
Chat with us on Discord.
[utopian-moderator]
Hey @team2dev
Thanks for contributing on Utopian.
We’re already looking forward to your next contribution!
Contributing on Utopian
Learn how to contribute on our website or by watching this tutorial on Youtube.
Want to chat? Join us on Discord https://discord.gg/h52nFrV.
Vote for Utopian Witness!