A Beginners Guide to Dart - Scope, Iterators, Functional Programming and Collections - Part Six
Repository
https://github.com/dart-lang/sdk
What Will I Learn?
- You will learn about Lexical Scoping
- You will learn about Iterators in Dart
- You will learn about Collection Types
- You will learn about Iterator and Collection Methods
- You will learn about Functional Programming Concepts
Requirements
System Requirements:
- IDEA intellij or Visual Studio Code with the Dart Plugins
- The Dart SDK
OS Support for Dart:
- Windows
- macOS
- Linux
Required Knowledge
- The Dart SDK
- A Dart supported text editor (Dart Pad can be used)
- A little time to sit and watch a video and some patience to learn the language
Resources for Dart:
- Dart Website: https://www.dartlang.org/
- Dart Official Documentation: https://www.dartlang.org/guides/language/language-tour
- Dart GitHub repository: https://github.com/dart-lang/sdk
- Awesome Dart GitHub Repository: https://github.com/yissachar/awesome-dart
- Pub website: https://pub.dartlang.org
Sources:
Dart Logo (Google): https://www.dartlang.org/
Difficulty
- Beginner
Description
In this Dart Tutorial, we take a look at some different concepts. We look at Lexical Scoping, Collections such as Lists and Maps, Iterators and Functional Methods for traversing through iterators and collections. This includes the inheritance and class structure for these different collection types and how the iterator type relates to them. We also look at the for-in
syntactic sugar.
Lexical Scoping and Block Scope in Dart
Dart as a language has a very straight forward scoping model. It embraces the concept of Lexical Scoping or Block Scoping. When a variable is defined, it is automatically exposed to the block that it is defined in. This also includes any blocks that are inside of that original block. The variable is not directly exposed to outside blocks unless the developer specifies otherwise. Also, variables can be passed to objects and functions via parameters and through other methods in this way. Dart also features a Global Scope which allows a variable or resource to be exposed to all of the code in a file and project.
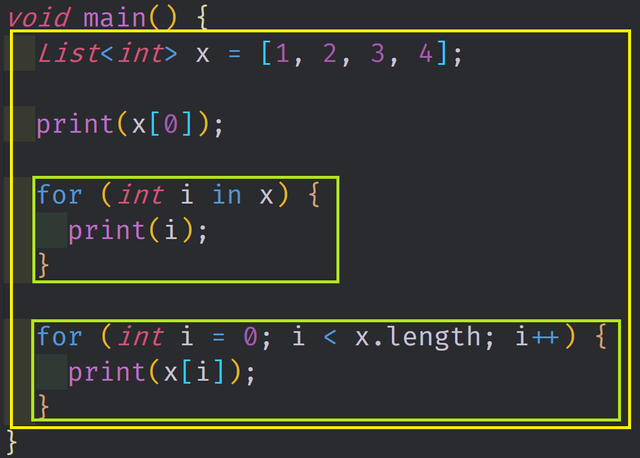
This image features some fairly basic code but it has a good example of lexical scoping. In the top of the main function, an X value was defined. This x
value is exposed to anything that appears inside of the yellow box, including the two green boxes. In the first green box, we have a for loop that defines an integer variable i
. This i
variable is used to iterate through the list x
and its scoped to this green box. The second green box features yet another i
variable. This new i
variable can be defined because the former i
variable is no longer in the scope when it is declared. Even though both of these variables use the same name, they are different from one another and can co-exist in the code since they are in seperate scopes.
Working with Iterators/iterables and Collections
Collections are a very common type to use in any programming language. In Dart, the main collection types are the List, Set, Map and Queue. List and Set both directly inherit from a class called iterable and Map and Queue both have their own parent classes that are very similar to this iterable class. The iterable class is important because it allows the program to read over a collection of data without mutating the data. It does this by way of lazy evaluation; the iterable only reads a value if it is asked to read that value. The iterable is what enables the for-in
loop as well as the three primary functional methods, map
, reduce
and filter
or where
in the case of Dart.
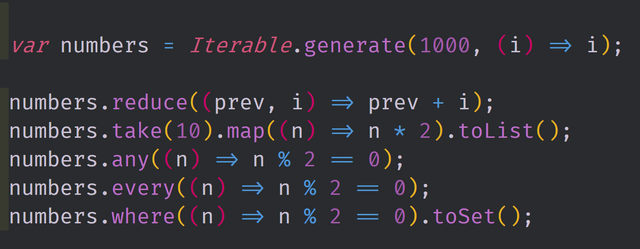
In the image above, the code defines an iterable value that contains 1000 items starting from 0 and ending at 999. Below it are some examples of the various methods that are attached to the iterable class. In the first line, the reduce
method is being used to add all of the values in the iterable together into a single value; 0 + 1 + 2 + 3... + 999
. On the next line, the take
method is chained into a map
method. The take
method uses the iterable's lazy nature to only evaluate the first 10 values then the map
method takes those values and multiplies them all by 2 giving us a new list of values via toList()
. The final three lines show off three of the conditional operators in Dart which are all variants of the where
or filter
method. The any
method iterates through the collection and find out if any item matches the conditional. If a single item does, then it will return a true
value. The every
method is the exact opposite in that it only returns true
if all of the values in the list meet the conditional statement. Finally, we have the where
method which will return values that satisfy the conditional in a iterable type. In this case, we are turning that iterable into a set
collection type through a chained toSet()
method.
The Source Code for this video may be found here: https://github.com/tensor-programming/dart_for_beginners/tree/tensor-programming-patch-5
Video Tutorial
Curriculum
- A Beginners Guide to Dart - Inheritance, Abstract Classes, Interfaces, Mixins and Casting - Part Five
- A Beginners Guide to Dart - Methods, Final, Static, and Class Inheritance - Part Four
- A Beginners Guide to Dart - Intro to Classes and Objects - Part Three
- A Beginners Guide to Dart - Control Flow and Low Level Compilation - Part Two
- A Beginners Guide to Dart - Types, Functions, Variables and Objects - Part One
Hi @tensor,
Great to have you doing these Dart tutorials for beginners.
You outline is easy to follow and your video tutorial is easy to understand with the flow of teaching.
It is a pleasure to watch these videos.
Your contribution has been evaluated according to Utopian policies and guidelines, as well as a predefined set of questions pertaining to the category.
To view those questions and the relevant answers related to your post, click here.
Need help? Chat with us on Discord.
Thank you @rosatravels. Always great to read your comments on my contributions.
Thank you for your review, @rosatravels! Keep up the good work!
Hi @tensor!
Your post was upvoted by @steem-ua, new Steem dApp, using UserAuthority for algorithmic post curation!
Your post is eligible for our upvote, thanks to our collaboration with @utopian-io!
Feel free to join our @steem-ua Discord server
Hi, @tensor!
You just got a 5.99% upvote from SteemPlus!
To get higher upvotes, earn more SteemPlus Points (SPP). On your Steemit wallet, check your SPP balance and click on "How to earn SPP?" to find out all the ways to earn.
If you're not using SteemPlus yet, please check our last posts in here to see the many ways in which SteemPlus can improve your Steem experience on Steemit and Busy.
Hey, @tensor!
Thanks for contributing on Utopian.
We’re already looking forward to your next contribution!
Get higher incentives and support Utopian.io!
Simply set @utopian.pay as a 5% (or higher) payout beneficiary on your contribution post (via SteemPlus or Steeditor).
Want to chat? Join us on Discord https://discord.gg/h52nFrV.
Vote for Utopian Witness!