Steem development #1: First step into developing an app with the Steem Blockchain
As a software developer by trade, exploring the Steem blockchain was something that peaked my interested as soon as I joined Steem. I'lll go over how I made my first simple application onto of the Steem blockchain, and how you can get started, too! No programming experience is needed, I'll explain the concepts as we go.
The app I'll be building is simple - just a web page that displays a user's followers. Similar to what the "followers" tab on steemworld does. The final result will look like this:
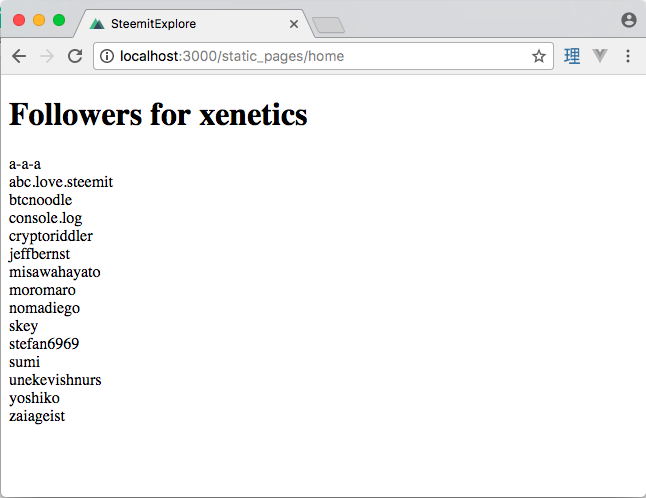
Preparation
If you are following along, the first thing you will need to do is install the required software. I'll be using Ruby on Rails, a web framework written in the Ruby programming languages. Many of your favorite applications such as Airbnb, Hulu and Twitter (in the early days) are built with Rails. You can install it by following the step by step instructions on Install Rails.
Creating the application
Now we are ready to start hacking. I'll generate a new Rails project running rails new steemit-app
in the terminal. A bunch of stuff prints out as the application is created. You can type cd steemit-app
to navigate inside the folder. Inside steemit-app
, there should be bunch more folders, like app
for the application, db
for the database, and some others.
Now we need to add one more thing - Steemit's Ruby library, or gem as well call them in the Ruby world, the the project. The Steemit developer documents recommends Radiator, which lets you access the Steem blockchain directly, created by the awesome @inertia, one of the (the main?) contributor to ChainBB. It even lets you sign transactions - this will let us expand the app in the future to allow for things like automatic voting, posting and realtime notifications.
To install radiator, inside of the steemit-app
folder, edit the file called Gemfile
and add the following line:
gem 'radiator', '~> 0.3.15 '
.
Now we simply run bundle
from the command line, to install our newly added radiator
gem.
Now, run rails server
to start the website server. If everything went well, you should be able to navigate to localhost:3000
in your web browser, and be greeted with:
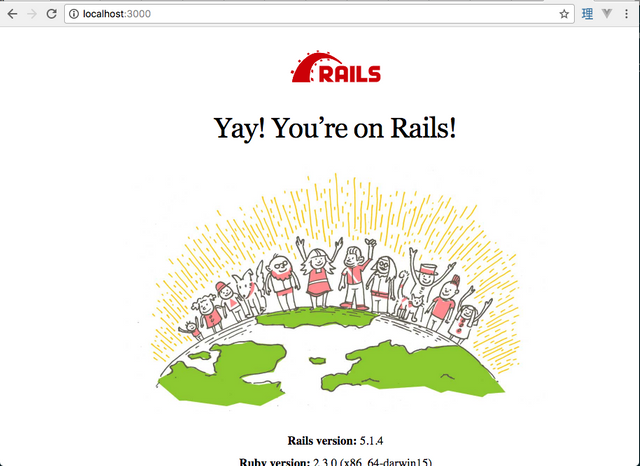
Great! Time to write some code.
Creating the controller
This is the last step. We will create a controller
- basically, a route on the website that returns some data. For example, https://steemit.com/@xenetics returns a page with my profile, and some data. There is a controller listening for requests - when I make a request to that URL, the server responds with my profile. In our case, we want to response with a list of followers.
Creating a new controller is easy in Rails. Run rails generate controller static_pages home
. This will create a controller called static_pages
, with a route called home
. This means if we visit localhost:3000/static_pages/home
, the server will respond with whatever data we tell it to. If everything went well, you should be able to visit that URL now, and see:
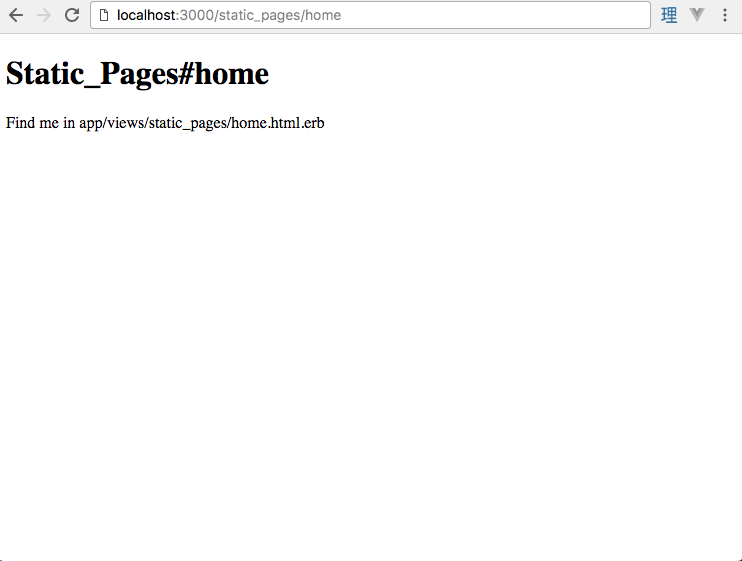
Showing the followers
Here comes the exciting part. Inside of app/controllers/static_pages
you should see:
class StaticPagesController < ApplicationController
def home
end
end
To show the users, we need to require
radiator, and then its Radiator::FollowApi
method, which you can find in the documentation. The complete code looks like this:
require 'radiator'
class StaticPagesController < ApplicationController
def home
@user = 'xenetics'
api = Radiator::FollowApi.new
api.get_followers(@user, 0, 'blog', 100) do |followers|
@followers = followers.map(&:follower)
end
end
end
I declare a @user
variable, create a new api
instance, and then assign all the followers (top 100, actually) to a @followers
variable.
The last thing we need to do is actually show this data in the view. Open /app/views/static_pages/home.html.erb
, and add the following code:
<h1>Followers for <%= @user %></h1>
<% @followers.each do |follower| %>
<div>
<%= follower %>
</div>
<% end %>
We iterate over each user and print their name out. That's it! If you typed everything correctly, you should be able to refresh the page and see:
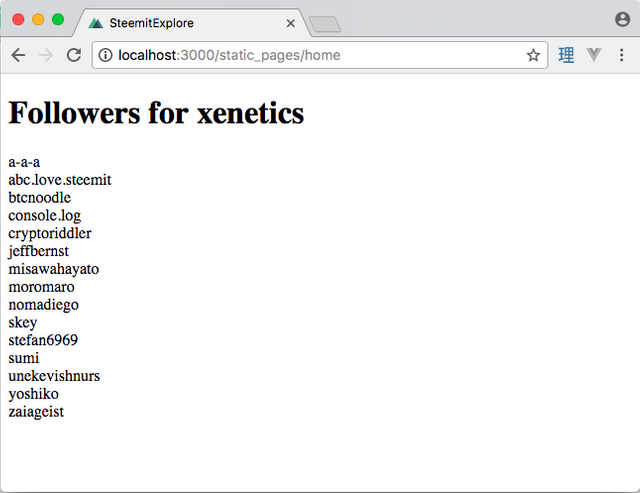
Great! Your first Steem blockchain app. I uploaded the final code here for reference.
I'll be improving the app in the future, so please let me know in the comments for features you'd like to learn about implementing, or anything else related.
Posted on Utopian.io - Rewarding Open Source Contributors
Hello,
Thanks for the contribution. It's a great content and will help onboarding potential Ruby developers for sure!
However, this looks like more a tutorial. You can select "Radiator" for the repository.
Since we can not move blog posts to tutorials at this time, so please edit this contribution as "DELETED" and create a new one on the correct category.
You can contact us on Discord.
[utopian-moderator]
Hey @emrebeyler, I just gave you a tip for your hard work on moderation. Upvote this comment to support the utopian moderators and increase your future rewards!
FYI: This seemed straightforward enough... The instructions are clear, I followed the steps, installed Ruby 2.3.3 on Windows 10, and added the radiator gem successfully:
$ bundle info radiator
* radiator (0.3.15)
Summary: STEEM RPC Ruby Client
Homepage: https://github.com/inertia186/radiator
Path: d:/git/RailsInstaller/Ruby2.3.3/lib/ruby/gems/2.3.0/gems/radiator-0.3.15
However the server does not start:
$ rails server
d:/git/RailsInstaller/Ruby2.3.3/lib/ruby/gems/2.3.0/gems/bundler-1.16.1/lib/bundler/runtime.rb:84:in `rescue in block (2 levels) in require': There was an error while trying to load the gem 'radiator'. (Bundler::GemRequireError)
Gem Load Error is: uninitialized constant Process::RLIMIT_NOFILE
I don't expect you to troubleshoot this, but just wanted you to be aware of a potential problem. I am not a Ruby developer, nor do I want to be, so I just tried to set this up out of curiosity. I'm familiar with JS though, so I'd be very interested in a JS version of this app.
Thanks for the feedback. Did you remember to run
bundle
after adding the gem? I can try and troubleshoot a little with you.I actually made something similar in JS recently, and since a few people asked, I'll try and get a JS version up this/early next week. JS is even easier to get started with.
Thanks again for the comment and feedback.
Yes indeed I ran
bundle
and I shared the output ofbundle info radiator
to show that the radiator gem is present.Thanks for offering to troubleshoot, but that is not necessary: I look forward to see your JS version of this tutorial, because your current tutorial is very well written. I don't intend to do anything with Ruby going forward, but a JS version I would probably experiment with and expand upon.
Sure, no problem. Thanks for the quick turnaround - will look into JS over the next few days. Do you think people prefer a JS environment using NPM to install dependencies, or just a CDN link style tutorial?
I'm not sure exactly what you mean by a "CDN link style tutorial". But I'm very familiar and comfortable with NPM and would probably prefer that. Even though it's easy to run into version-mismatch issues with NPM installs (which I suspect also to be the case in my Ruby install for your current tutorial).
Ok, sounds good. By CDN, I mean sometime you see people just make a
html
file and dump<script src="...">
instead of using npm to manage packages and the such, which obviously doesn't work well for anything other than a basic introduction to a new lib.Sounds good, I'd rather do things the right way and use npm to manage packages, maybe webpack to bundle or something.
Wonderful! NPM, webpack etc is the way to go for anything beyond the trivial.
BTW, I'm happy to test-run your tutorial before you publish it if you'd like.
Alright
[-]edward.maesen, let's do it. Published a draft here in the readme, also with a live demo. I decided to user React, since that is what Steemit.com is also built using, and it's the most popular UI framework at the moment.
https://github.com/lmiller1990/steem-followers-js
If you could go through it and let me know how things are going, that'd be great. Not sure if comments on this article is the best way to work on this or not, haha.
Oh man, this is great. I'm really interested in learning how to make apps on the Steem blockchain too. I'm learning full-stack JavaScript so I don't know Ruby, but it's crazy to see how simple it can be. Looking forward to more posts like this!
Hey @jeffbernst, just wanted to make sure you know that there is SteemJS.
Thanks! Yeah actually I was looking through some stuff today and came across it. It's awesome how easy it is to get some basic functionality set up!
Getting started with JS is actually even more simple, I think I'll look to do a JS app in the near future ;)
Thanks for the great article. I switch from Ruby to JS a few years back. Still, a very useful post for me.
Both are great langs. I'm actually a fulltime JS dev, and since a few people showed interest, I'll try and get a JS version up this/early next week. Thanks for the comment!
Would be great to see what you come up with. I'm currently on the same track. Started to build a SPA for the Steem blockchain a couple of days ago.